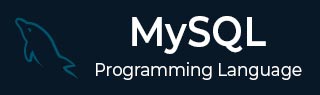
- MySQL Basics
- MySQL - Home
- MySQL - Introduction
- MySQL - Features
- MySQL - Versions
- MySQL - Variables
- MySQL - Installation
- MySQL - Administration
- MySQL - PHP Syntax
- MySQL - Node.js Syntax
- MySQL - Java Syntax
- MySQL - Python Syntax
- MySQL - Connection
- MySQL - Workbench
- MySQL Databases
- MySQL - Create Database
- MySQL - Drop Database
- MySQL - Select Database
- MySQL - Show Database
- MySQL - Copy Database
- MySQL - Database Export
- MySQL - Database Import
- MySQL - Database Info
- MySQL Users
- MySQL - Create Users
- MySQL - Drop Users
- MySQL - Show Users
- MySQL - Change Password
- MySQL - Grant Privileges
- MySQL - Show Privileges
- MySQL - Revoke Privileges
- MySQL - Lock User Account
- MySQL - Unlock User Account
- MySQL Tables
- MySQL - Create Tables
- MySQL - Show Tables
- MySQL - Alter Tables
- MySQL - Rename Tables
- MySQL - Clone Tables
- MySQL - Truncate Tables
- MySQL - Temporary Tables
- MySQL - Repair Tables
- MySQL - Describe Tables
- MySQL - Add/Delete Columns
- MySQL - Show Columns
- MySQL - Rename Columns
- MySQL - Table Locking
- MySQL - Drop Tables
- MySQL - Derived Tables
- MySQL Queries
- MySQL - Queries
- MySQL - Constraints
- MySQL - Insert Query
- MySQL - Select Query
- MySQL - Update Query
- MySQL - Delete Query
- MySQL - Replace Query
- MySQL - Insert Ignore
- MySQL - Insert on Duplicate Key Update
- MySQL - Insert Into Select
- MySQL Indexes
- MySQL - Indexes
- MySQL - Create Index
- MySQL - Drop Index
- MySQL - Show Indexes
- MySQL - Unique Index
- MySQL - Clustered Index
- MySQL - Non-Clustered Index
- MySQL Operators and Clauses
- MySQL - Where Clause
- MySQL - Limit Clause
- MySQL - Distinct Clause
- MySQL - Order By Clause
- MySQL - Group By Clause
- MySQL - Having Clause
- MySQL - AND Operator
- MySQL - OR Operator
- MySQL - Like Operator
- MySQL - IN Operator
- MySQL - ANY Operator
- MySQL - EXISTS Operator
- MySQL - NOT Operator
- MySQL - NOT EQUAL Operator
- MySQL - IS NULL Operator
- MySQL - IS NOT NULL Operator
- MySQL - Between Operator
- MySQL - UNION Operator
- MySQL - UNION vs UNION ALL
- MySQL - MINUS Operator
- MySQL - INTERSECT Operator
- MySQL - INTERVAL Operator
- MySQL Joins
- MySQL - Using Joins
- MySQL - Inner Join
- MySQL - Left Join
- MySQL - Right Join
- MySQL - Cross Join
- MySQL - Full Join
- MySQL - Self Join
- MySQL - Delete Join
- MySQL - Update Join
- MySQL - Union vs Join
- MySQL Keys
- MySQL - Unique Key
- MySQL - Primary Key
- MySQL - Foreign Key
- MySQL - Composite Key
- MySQL - Alternate Key
- MySQL Triggers
- MySQL - Triggers
- MySQL - Create Trigger
- MySQL - Show Trigger
- MySQL - Drop Trigger
- MySQL - Before Insert Trigger
- MySQL - After Insert Trigger
- MySQL - Before Update Trigger
- MySQL - After Update Trigger
- MySQL - Before Delete Trigger
- MySQL - After Delete Trigger
- MySQL Data Types
- MySQL - Data Types
- MySQL - VARCHAR
- MySQL - BOOLEAN
- MySQL - ENUM
- MySQL - DECIMAL
- MySQL - INT
- MySQL - FLOAT
- MySQL - BIT
- MySQL - TINYINT
- MySQL - BLOB
- MySQL - SET
- MySQL Regular Expressions
- MySQL - Regular Expressions
- MySQL - RLIKE Operator
- MySQL - NOT LIKE Operator
- MySQL - NOT REGEXP Operator
- MySQL - regexp_instr() Function
- MySQL - regexp_like() Function
- MySQL - regexp_replace() Function
- MySQL - regexp_substr() Function
- MySQL Fulltext Search
- MySQL - Fulltext Search
- MySQL - Natural Language Fulltext Search
- MySQL - Boolean Fulltext Search
- MySQL - Query Expansion Fulltext Search
- MySQL - ngram Fulltext Parser
- MySQL Functions & Operators
- MySQL - Date and Time Functions
- MySQL - Arithmetic Operators
- MySQL - Numeric Functions
- MySQL - String Functions
- MySQL - Aggregate Functions
- MySQL Misc Concepts
- MySQL - NULL Values
- MySQL - Transactions
- MySQL - Using Sequences
- MySQL - Handling Duplicates
- MySQL - SQL Injection
- MySQL - SubQuery
- MySQL - Comments
- MySQL - Check Constraints
- MySQL - Storage Engines
- MySQL - Export Table into CSV File
- MySQL - Import CSV File into Database
- MySQL - UUID
- MySQL - Common Table Expressions
- MySQL - On Delete Cascade
- MySQL - Upsert
- MySQL - Horizontal Partitioning
- MySQL - Vertical Partitioning
- MySQL - Cursor
- MySQL - Stored Functions
- MySQL - Signal
- MySQL - Resignal
- MySQL - Character Set
- MySQL - Collation
- MySQL - Wildcards
- MySQL - Alias
- MySQL - ROLLUP
- MySQL - Today Date
- MySQL - Literals
- MySQL - Stored Procedure
- MySQL - Explain
- MySQL - JSON
- MySQL - Standard Deviation
- MySQL - Find Duplicate Records
- MySQL - Delete Duplicate Records
- MySQL - Select Random Records
- MySQL - Show Processlist
- MySQL - Change Column Type
- MySQL - Reset Auto-Increment
- MySQL - Coalesce() Function
MySQL - Interval Operator
MySQL INTERVAL Operator
The INTERVAL operator in MySQL is used to create an interval between two different events or times. This interval can be in seconds, minutes, hours, days, etc. Thus, MySQL mainly uses this operator to perform date and time calculations, such as adding or subtracting a specified time interval from date and time values.
INTERVAL operator is used with various date and time functions, and helps in real-time scenarios for calculating the deadlines, scheduling events, etc.
Syntax
Following is the syntax of INTERVAL operator in MySQL −
INTERVAL expr unit
Where,
- expr: is a keyword that specifies the interval value.
- unit: keyword determines the interval unit (such as DAY, HOUR, MINUTE, etc.).
Note: The INTERVAL and UNIT are case-insensitive.
Standard Formats For Interval Expressions and Units
Following is the table of MySQL standard formats for the interval expressions and its corresponding unit −
unit | expr |
---|---|
DAY | DAYS |
DAY_HOUR | 'DAYS HOURS' |
DAY_MICROSECOND | 'DAYS HOURS:MINUTES:SECONDS.MICROSECONDS' |
DAY_MINUTE | 'DAYS HOURS:MINUTES' |
DAY_SECOND | 'DAYS HOURS:MINUTES:SECONDS' |
HOUR | HOURS |
HOUR_MICROSECOND | 'HOURS:MINUTES:SECONDS.MICROSECONDS' |
HOUR_MINUTE | 'HOURS:MINUTES' |
HOUR_SECOND | 'HOURS:MINUTES:SECONDS' |
MICROSECOND | MICROSECONDS |
MINUTE | MINUTES |
MINUTE_MICROSECOND | 'MINUTES:SECONDS.MICROSECONDS' |
MINUTE_SECOND | 'MINUTES:SECONDS' |
MONTH | MONTHS |
QUARTER | QUARTERS |
SECOND | SECONDS |
SECOND_MICROSECOND | 'SECONDS.MICROSECONDS' |
WEEK | WEEKS |
YEAR | YEARS |
YEAR_MONTH | 'YEAR_MONTHS' |
Example
The following query adds 10 days to the date “2023-04-14” −
SELECT '2023-04-14' + INTERVAL 10 DAY;
Output
The output for the query above is produced as given below −
'2023-04-14' + INTERVAL 10 DAY |
---|
2023-04-24 |
Example
The following query subtracts 5 days from the date "2023-04-14" −
SELECT '2023-04-14' - INTERVAL 5 DAY;
Output
The output for the query above is produced as given below −
'2023-04-14' - INTERVAL 5 DAY |
---|
2023-04-09 |
Example
Here, we are adding two hours to the datetime value "2023-04-14 09:45:30.000" −
SELECT '2023-04-14 09:45:30.000' + INTERVAL 2 HOUR;
Output
Following is the output −
'2023-04-14 09:45:30.000' + INTERVAL 2 HOUR |
---|
2023-04-14 11:45:30 |
Example
The following query is subtracting sixty minutes from the datetime value "2023-04-14 09:45:30.000" −
SELECT '2023-04-14 09:45:30.000' - INTERVAL 60 MINUTE;
Output
Following is the output −
'2023-04-14 09:45:30.000' - INTERVAL 60 MINUTE |
---|
2023-04-14 08:45:30 |
Example
Here, we are adding and deleting one from the date '2023-04-14' −
SELECT DATE_ADD('2023-04-14', INTERVAL 1 MONTH) ADD_ONE_MONTH, DATE_SUB('2023-04-14',INTERVAL 1 MONTH) SUB_ONE_MONTH;
Output
On executing the given query, the output is displayed as follows −
ADD_ONE_MONTH | SUB_ONE_MONTH |
---|---|
2023-05-14 | 2023-03-14 |
Example
In the following query, we are using the TIMESTAMPADD() function to add two hours to the timestamp value −
SELECT TIMESTAMPADD (HOUR, 2, '2020-01-01 03:30:43.000') 2_HOURS_LATER;
Output
Let us compile and run the query, to produce the following result −
2_HOURS_LATER |
---|
2020-01-01 05:30:43 |
Example
Now, let us create a table with a name OTT using the following query −
CREATE TABLE OTT ( ID INT NOT NULL, SUBSCRIBER_NAME VARCHAR (200) NOT NULL, MEMBERSHIP VARCHAR (200), EXPIRED_DATE DATE NOT NULL );
Using the following query, we are inserting some records into the above-created table using the INSERT INTO statement as shown below −
INSERT INTO OTT VALUES (1, 'Dhruv', 'Silver', '2023-04-30'), (2, 'Arjun','Platinum', '2023-04-01'), (3, 'Dev','Silver', '2023-04-23'), (4, 'Riya','Gold', '2023-04-05'), (5, 'Aarohi','Platinum', '2023-04-02'), (6, 'Lisa','Platinum', '2023-04-25'), (7, 'Roy','Gold', '2023-04-26');
The table is created as −
ID | SUBSCRIBER_NAME | MEMBERSHIP | EXPIRED_DATE |
---|---|---|---|
1 | Dhruv | Silver | 2023-04-30 |
2 | Arjun | Platinum | 2023-04-01 |
3 | Dev | Silver | 2023-04-23 |
4 | Riya | Gold | 2023-04-05 |
5 | Aarohi | Platinum | 2023-04-02 |
6 | Lisa | Platinum | 2023-04-25 |
7 | Roy | Gold | 2023-04-26 |
Now, we are selecting data from the OTT table for the subscribers whose membership is about to expire within the next 7 days from the specific date of '2023-04-01'.
SELECT ID, SUBSCRIBER_NAME, MEMBERSHIP, EXPIRED_DATE, DATEDIFF(expired_date, '2023-04-01') EXPIRING_IN FROM OTT WHERE '2023-04-01' BETWEEN DATE_SUB(EXPIRED_DATE, INTERVAL 7 DAY) AND EXPIRED_DATE;
On executing the given query, the output is displayed as follows −
ID | SUBSCRIBER_NAME | MEMBERSHIP | EXPIRED_DATE | EXPIRED_IN |
---|---|---|---|---|
1 | Arjun | Platinum | 2023-04-01 | 0 |
2 | Riya | Gold | 2023-04-05 | 4 |
3 | Aarohi | Platinum | 2023-04-02 | 1 |
Interval Operator Using Client Program
In addition to executing the Interval Operator in MySQL table using an SQL query, we can also apply the INTERVAL operator on a table using a client program.
Syntax
Following are the syntaxes of the Interval Operator in MySQL table in various programming languages −
To execute the Interval operator in MySQL table through a PHP program, we need to execute INTERVAL statement using the query() function of mysqli connector.
$sql = "INTERVAL expr unit"; $mysqli->query($sql);
To execute the Interval operator in MySQL table through a JavaScript program, we need to execute INTERVAL statement using the query() function of mysql2 connector.
sql = "INTERVAL expr unit"; con.query(sql);
To execute the Interval operator in MySQL table through a Java program, we need to execute INTERVAL statement using the executeQuery() function of JDBC type 4 driver.
String sql = "INTERVAL expr unit"; statement.executeQuery(sql);
To execute the Interval operator in MySQL table through a Python program, we need to execute INTERVAL statement using the execute() function provided by MySQL Connector/Python.
interval_query = "INTERVAL expr unit" cursorObj.execute(interval_query);
Example
Following are the implementations of this operation in various programming languages −
$dbhost = 'localhost'; $dbuser = 'root'; $dbpass = 'password'; $dbname = 'TUTORIALS'; $mysqli = new mysqli($dbhost, $dbuser, $dbpass, $dbname); if($mysqli->connect_errno ) { printf("Connect failed: %s
", $mysqli->connect_error); exit(); } //printf('Connected successfully.
'); $sql = "SELECT '2023-04-14' + INTERVAL 10 DAY AS DATE"; $result = $mysqli->query($sql); if ($result->num_rows > 0) { printf("Date '2023-04-14' after 10 days: \n"); while($row = $result->fetch_assoc()) { printf("DATE: %s", $row["DATE"],); printf("\n"); } } else { printf('Error.
'); } mysqli_free_result($result); $mysqli->close();
Output
The output obtained is as follows −
Date '2023-04-14' after 10 days: DATE: 2023-04-24
var mysql = require('mysql2'); var con = mysql.createConnection({ host: "localhost", user: "root", password: "Nr5a0204@123" }); //Connecting to MySQL con.connect(function (err) { if (err) throw err; console.log("Connected!"); console.log("--------------------------"); //Creating a Database sql = "create database TUTORIALS" con.query(sql); //Select database sql = "USE TUTORIALS" con.query(sql); //Creating OTT table sql = "CREATE TABLE OTT (ID INT NOT NULL,SUBSCRIBER_NAME VARCHAR (200) NOT NULL,MEMBERSHIP VARCHAR (200),EXPIRED_DATE DATE NOT NULL);" con.query(sql); //Inserting Records sql = "INSERT INTO OTT(ID, SUBSCRIBER_NAME, MEMBERSHIP, EXPIRED_DATE) VALUES(1, 'Dhruv', 'Silver', '2023-04-30'),(2, 'Arjun','Platinum', '2023-04-01'),(3, 'Dev','Silver', '2023-04-23'),(4, 'Riya','Gold', '2023-04-05'),(5, 'Aarohi','Platinum', '2023-04-02'),(6, 'Lisa','Platinum', '2023-04-25'),(7, 'Roy','Gold', '2023-04-26');" con.query(sql); //Using INTERSECT Operator sql = "SELECT ID, SUBSCRIBER_NAME, MEMBERSHIP, EXPIRED_DATE, DATEDIFF(expired_date, '2023-04-01') Expiring_in FROM OTT WHERE '2023-04-01' BETWEEN DATE_SUB(EXPIRED_DATE, INTERVAL 7 DAY) AND EXPIRED_DATE;" con.query(sql, function(err, result){ if (err) throw err console.log(result) }); });
Output
The output produced is as follows −
Connected! -------------------------- [ { ID: 2, SUBSCRIBER_NAME: 'Arjun', MEMBERSHIP: 'Platinum', EXPIRED_DATE: 2023-03-31T18:30:00.000Z, Expiring_in: 0 }, { ID: 4, SUBSCRIBER_NAME: 'Riya', MEMBERSHIP: 'Gold', EXPIRED_DATE: 2023-04-04T18:30:00.000Z, Expiring_in: 4 }, { ID: 5, SUBSCRIBER_NAME: 'Aarohi', MEMBERSHIP: 'Platinum', EXPIRED_DATE: 2023-04-01T18:30:00.000Z, Expiring_in: 1 } ]
import java.sql.Connection; import java.sql.DriverManager; import java.sql.ResultSet; import java.sql.Statement; public class IntervalClause { public static void main(String[] args) { String url = "jdbc:mysql://localhost:3306/TUTORIALS"; String user = "root"; String password = "password"; ResultSet rs; try { Class.forName("com.mysql.cj.jdbc.Driver"); Connection con = DriverManager.getConnection(url, user, password); Statement st = con.createStatement(); //System.out.println("Database connected successfully...!"); String sql = "SELECT '2023-04-14' + INTERVAL 10 DAY"; rs = st.executeQuery(sql); System.out.print("Date '2023-04-14' after 10 days: "); while(rs.next()){ String date = rs.getNString(1); System.out.println(date); System.out.println(); } }catch(Exception e) { e.printStackTrace(); } } }
Output
The output obtained is as shown below −
Date '2023-04-14' after 10 days: 2023-04-24
import mysql.connector #establishing the connection connection = mysql.connector.connect( host='localhost', user='root', password='password', database='tut' ) cursorObj = connection.cursor() interval_query = f""" SELECT '2023-05-28' + INTERVAL 10 DAY; """ cursorObj.execute(interval_query) # Fetching all the rows that meet the criteria filtered_rows = cursorObj.fetchall() for row in filtered_rows: print(row) cursorObj.close() connection.close()
Output
Following is the output of the above code −
('2023-06-07',)