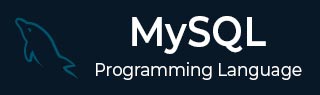
- MySQL Basics
- MySQL - Home
- MySQL - Introduction
- MySQL - Features
- MySQL - Versions
- MySQL - Variables
- MySQL - Installation
- MySQL - Administration
- MySQL - PHP Syntax
- MySQL - Node.js Syntax
- MySQL - Java Syntax
- MySQL - Python Syntax
- MySQL - Connection
- MySQL - Workbench
- MySQL Databases
- MySQL - Create Database
- MySQL - Drop Database
- MySQL - Select Database
- MySQL - Show Database
- MySQL - Copy Database
- MySQL - Database Export
- MySQL - Database Import
- MySQL - Database Info
- MySQL Users
- MySQL - Create Users
- MySQL - Drop Users
- MySQL - Show Users
- MySQL - Change Password
- MySQL - Grant Privileges
- MySQL - Show Privileges
- MySQL - Revoke Privileges
- MySQL - Lock User Account
- MySQL - Unlock User Account
- MySQL Tables
- MySQL - Create Tables
- MySQL - Show Tables
- MySQL - Alter Tables
- MySQL - Rename Tables
- MySQL - Clone Tables
- MySQL - Truncate Tables
- MySQL - Temporary Tables
- MySQL - Repair Tables
- MySQL - Describe Tables
- MySQL - Add/Delete Columns
- MySQL - Show Columns
- MySQL - Rename Columns
- MySQL - Table Locking
- MySQL - Drop Tables
- MySQL - Derived Tables
- MySQL Queries
- MySQL - Queries
- MySQL - Constraints
- MySQL - Insert Query
- MySQL - Select Query
- MySQL - Update Query
- MySQL - Delete Query
- MySQL - Replace Query
- MySQL - Insert Ignore
- MySQL - Insert on Duplicate Key Update
- MySQL - Insert Into Select
- MySQL Indexes
- MySQL - Indexes
- MySQL - Create Index
- MySQL - Drop Index
- MySQL - Show Indexes
- MySQL - Unique Index
- MySQL - Clustered Index
- MySQL - Non-Clustered Index
- MySQL Operators and Clauses
- MySQL - Where Clause
- MySQL - Limit Clause
- MySQL - Distinct Clause
- MySQL - Order By Clause
- MySQL - Group By Clause
- MySQL - Having Clause
- MySQL - AND Operator
- MySQL - OR Operator
- MySQL - Like Operator
- MySQL - IN Operator
- MySQL - ANY Operator
- MySQL - EXISTS Operator
- MySQL - NOT Operator
- MySQL - NOT EQUAL Operator
- MySQL - IS NULL Operator
- MySQL - IS NOT NULL Operator
- MySQL - Between Operator
- MySQL - UNION Operator
- MySQL - UNION vs UNION ALL
- MySQL - MINUS Operator
- MySQL - INTERSECT Operator
- MySQL - INTERVAL Operator
- MySQL Joins
- MySQL - Using Joins
- MySQL - Inner Join
- MySQL - Left Join
- MySQL - Right Join
- MySQL - Cross Join
- MySQL - Full Join
- MySQL - Self Join
- MySQL - Delete Join
- MySQL - Update Join
- MySQL - Union vs Join
- MySQL Keys
- MySQL - Unique Key
- MySQL - Primary Key
- MySQL - Foreign Key
- MySQL - Composite Key
- MySQL - Alternate Key
- MySQL Triggers
- MySQL - Triggers
- MySQL - Create Trigger
- MySQL - Show Trigger
- MySQL - Drop Trigger
- MySQL - Before Insert Trigger
- MySQL - After Insert Trigger
- MySQL - Before Update Trigger
- MySQL - After Update Trigger
- MySQL - Before Delete Trigger
- MySQL - After Delete Trigger
- MySQL Data Types
- MySQL - Data Types
- MySQL - VARCHAR
- MySQL - BOOLEAN
- MySQL - ENUM
- MySQL - DECIMAL
- MySQL - INT
- MySQL - FLOAT
- MySQL - BIT
- MySQL - TINYINT
- MySQL - BLOB
- MySQL - SET
- MySQL Regular Expressions
- MySQL - Regular Expressions
- MySQL - RLIKE Operator
- MySQL - NOT LIKE Operator
- MySQL - NOT REGEXP Operator
- MySQL - regexp_instr() Function
- MySQL - regexp_like() Function
- MySQL - regexp_replace() Function
- MySQL - regexp_substr() Function
- MySQL Fulltext Search
- MySQL - Fulltext Search
- MySQL - Natural Language Fulltext Search
- MySQL - Boolean Fulltext Search
- MySQL - Query Expansion Fulltext Search
- MySQL - ngram Fulltext Parser
- MySQL Functions & Operators
- MySQL - Date and Time Functions
- MySQL - Arithmetic Operators
- MySQL - Numeric Functions
- MySQL - String Functions
- MySQL - Aggregate Functions
- MySQL Misc Concepts
- MySQL - NULL Values
- MySQL - Transactions
- MySQL - Using Sequences
- MySQL - Handling Duplicates
- MySQL - SQL Injection
- MySQL - SubQuery
- MySQL - Comments
- MySQL - Check Constraints
- MySQL - Storage Engines
- MySQL - Export Table into CSV File
- MySQL - Import CSV File into Database
- MySQL - UUID
- MySQL - Common Table Expressions
- MySQL - On Delete Cascade
- MySQL - Upsert
- MySQL - Horizontal Partitioning
- MySQL - Vertical Partitioning
- MySQL - Cursor
- MySQL - Stored Functions
- MySQL - Signal
- MySQL - Resignal
- MySQL - Character Set
- MySQL - Collation
- MySQL - Wildcards
- MySQL - Alias
- MySQL - ROLLUP
- MySQL - Today Date
- MySQL - Literals
- MySQL - Stored Procedure
- MySQL - Explain
- MySQL - JSON
- MySQL - Standard Deviation
- MySQL - Find Duplicate Records
- MySQL - Delete Duplicate Records
- MySQL - Select Random Records
- MySQL - Show Processlist
- MySQL - Change Column Type
- MySQL - Reset Auto-Increment
- MySQL - Coalesce() Function
MySQL − Queries
MySQL is an open-source relational management system (RDBMS) that allows us to store and manage data or information. The queries in MySQL are commands that are used to retrieve or manipulate the data from a database table.
Following are the commonly used commands in MySQL: SELECT, UPDATE, DELETE, INSERT INTO, CREATE TABLE, ALTER TABLE, DROP TABLE, CREATE DATABASE, ALTER DATABASE, CREATE INDEX, DROP INDEX, etc.
Note: These keywords are not case-sensitive. For instance, create table is the same as CREATE TABLE.
MySQL Create Database
The create database query in MySQL can be used to create a database in the MySQL server.
Syntax
Following is the syntax for the query −
CREATE DATABASE databasename;
Example
In the following query, we are creating a database named tutorials.
CREATE DATABASE tutorials;
MySQL Use Database
The MySQL use database query is used to select a database to perform operations such as creating, inserting, updating tables or views, etc.
Syntax
Following is the syntax for the query −
USE database_name;
Example
The following query selects a database named tutorials −
USE tutorials;
MySQL Create Query
The MySQL create query can be used to create databases, tables, indexes, views, etc.
Syntax
Following is the syntax for the query −
CREATE [table table_name |index index_name | view view_name];
Example
Here, we are creating a table named STUDENTS using the following CREATE query −
CREATE TABLE CUSTOMERS ( ID int, NAME varchar(20), AGE int, PRIMARY KEY (ID) );
MySQL Insert Query
The MySQL insert query can be used to insert records within a specified table.
Syntax
Following is the syntax for the query −
INSERT INTO table_name (column1, column2, column3, ...) VALUES (value1, value2, value3, ...);
Example
In the following query, we are inserting some records into a table named CUSTOMERS −
INSERT INTO CUSTOMERS (ID, NAME, AGE) VALUES (1, "Nikhilesh", 28); INSERT INTO STUDENTS (ID, NAME, AGE) VALUES (2, "Akhil", 23); INSERT INTO STUDENTS (ID, NAME, AGE) VALUES (3, "Sushil", 35);
MySQL Update Query
The MySQL update query can be used to modify the existing records in a specified table.
Syntax
Following is the syntax for the query −
UPDATE table_name SET column1 = value1, column2 = value2, ... WHERE condition;
Example
UPDATE CUSTOMERS SET NAME = "Nikhil" WHERE ID = 1;
MySQL Alter Query
The ALTER query in MySQL can be used to add, delete, or modify columns in an existing table.
Syntax
Following is the syntax for the query −
ALTER TABLE table_name [ADD|DROP] column_name datatype;
Example
Here, we are trying to add a column named ADDRESS to the existing CUSTOMERS table.
ALTER TABLE CUSTOMERS ADD COLUMN ADDRESS varchar(50);
MySQL Delete Query
The Delete query in MySQL can be used to delete existing records in a specified table.
Syntax
Following is the syntax for the query −
DELETE FROM table_name WHERE condition;
Example
In the following query, we are deleting a record from CUSTOMERS table where the ID is equal to 3.
DELETE FROM CUSTOMERS WHERE ID = 3;
MySQL Truncate Table Query
The MySQL truncate table query can be used to remove all the records but not the table itself.
Syntax
Following is the syntax for the query −
TRUNCATE [TABLE] table_name;
Example
In the following query, we are removing all the records from the CUSTOMERS table using the truncate table query −
TRUNCATE TABLE CUSTOMERS;
MySQL Drop Query
The MySQL drop query is used to delete an existing table in a database.
Syntax
Following is the syntax for the query −
DROP TABLE table_name;
Example
Here, we are trying to delete the table named CUSTOMERS using the drop table query.
DROP TABLE CUSTOMERS;