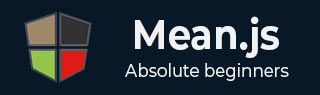
- MEAN.JS Tutorial
- MEAN.JS - Home
- MEAN.JS - Overview
- MEAN.JS - Architecture
- Build Node Web App
- MEAN.JS - Mean Project Setup
- Building Static Route Node Express
- MEAN.JS - Build Data Model
- MEAN.JS - REST API
- Front End with Angular
- Angular Components in App
- Building Single Page with Angular
- Building an SPA: The next level
- MEAN.JS Useful Resources
- MEAN.JS - Quick Guide
- MEAN.JS - Useful Resources
- MEAN.JS - Discussion
MEAN.JS - Build Data Model
In this chapter, we shall demonstrate how to use data model in our Node-express application.
MongoDB is an open source NoSQL database that saves the data in JSON format. It uses the document oriented data model to store the data instead of using table and rows as we use in the relational databases. In this chapter, we are using Mongodb to build data model.
Data model specifies what data is present in a document, and what data should be there in a document. Refer the Official MongoDB installation, to install the MongoDB.
We shall use our previous chapter code. You can download the source code in this link. Download the zip file; extract it in your system. Open the terminal and run the below command to install npm module dependencies.
$ cd mean-demo $ npm install
Adding Mongoose to Application
Mongoose is a data modelling library that specifies environment and structure for the data by making MongoDB powerful. You can install Mongoose as an npm module through the command line. Go to your root folder and run the below command −
$ npm install --save mongoose
The above command will download the new package and install it into the node_modules folder. The --save flag will add this package to package.json file.
{ "name": "mean_tutorial", "version": "1.0.0", "description": "this is basic tutorial example for MEAN stack", "main": "server.js", "scripts": { "test": "test" }, "keywords": [ "MEAN", "Mongo", "Express", "Angular", "Nodejs" ], "author": "Manisha", "license": "ISC", "dependencies": { "express": "^4.17.1", "mongoose": "^5.5.13" } }
Setting up Connection File
To work with data model, we will be using app/models folder. Let's create model students.js as below −
var mongoose = require('mongoose'); // define our students model // module.exports allows us to pass this to other files when it is called module.exports = mongoose.model('Student', { name : {type : String, default: ''} });
You can setup the connection file by creating the file and using it in the application. Create a file called db.js in config/db.js. The file contents are as below −
module.exports = { url : 'mongodb://localhost:27017/test' }
Here test is the database name.
Here it is assumed that you have installed MongoDB locally. Once installed start Mongo and create a database by name test. This db will have a collection by name students. Insert some data to this colection. In our case, we have inserted a record using db.students.insertOne( { name: 'Manisha' , place: 'Pune', country: 'India'} );
Bring the db.js file into application, i.e., in server.js. Contents of the file are as shown below −
// modules ================================================= const express = require('express'); const app = express(); var mongoose = require('mongoose'); // set our port const port = 3000; // configuration =========================================== // config files var db = require('./config/db'); console.log("connecting--",db); mongoose.connect(db.url); //Mongoose connection created // frontend routes ========================================================= app.get('/', (req, res) ⇒ res.send('Welcome to Tutorialspoint!')); //defining route app.get('/tproute', function (req, res) { res.send('This is routing for the application developed using Node and Express...'); }); // sample api route // grab the student model we just created var Student = require('./app/models/student'); app.get('/api/students', function(req, res) { // use mongoose to get all students in the database Student.find(function(err, students) { // if there is an error retrieving, send the error. // nothing after res.send(err) will execute if (err) res.send(err); res.json(students); // return all students in JSON format }); }); // startup our app at http://localhost:3000 app.listen(port, () ⇒ console.log(`Example app listening on port ${port}!`));
Next, run the application with the below command −
$ npm start
You will get a confirmation as shown in the image below −

Now, go to browser and type http://localhost:3000/api/students. You will get the page as shown in the image below −
