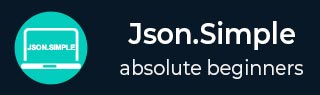
- JSON.simple Tutorial
- JSON.simple - Home
- JSON.simple - Overview
- JSON.simple - Environment Setup
- JSON.simple - JAVA Mapping
- Decoding Examples
- Escaping Special Characters
- JSON.simple - Using JSONValue
- JSON.simple - Exception Handling
- JSON.simple - Container Factory
- JSON.simple - Content Handler
- Encoding Examples
- JSON.simple - Encode JSONObject
- JSON.simple - Encode JSONArray
- Merging Examples
- JSON.simple - Merging Objects
- JSON.simple - Merging Arrays
- Combination Examples
- JSON.simple - Primitive, Object, Array
- JSON.simple - Primitive, Map, List
- Primitive, Object, Map, List
- Customization Examples
- JSON.simple - Customized Output
- Customized Output Streaming
- JSON.simple Useful Resources
- JSON.simple - Quick Guide
- JSON.simple - Useful Resources
- JSON.simple - Discussion
JSON.simple - Using JSONValue
JSONValue provide a static method parse() to parse the given json string to return a JSONObject which can then be used to get the values parsed. See the example below.
Example
import org.json.simple.JSONArray; import org.json.simple.JSONObject; import org.json.simple.JSONValue; public class JsonDemo { public static void main(String[] args) { String s = "[0,{\"1\":{\"2\":{\"3\":{\"4\":[5,{\"6\":7}]}}}}]"; Object obj = JSONValue.parse(s); JSONArray array = (JSONArray)obj; System.out.println("The 2nd element of array"); System.out.println(array.get(1)); System.out.println(); JSONObject obj2 = (JSONObject)array.get(1); System.out.println("Field \"1\""); System.out.println(obj2.get("1")); s = "{}"; obj = JSONValue.parse(s); System.out.println(obj); s = "[5,]"; obj = JSONValue.parse(s); System.out.println(obj); s = "[5,,2]"; obj = JSONValue.parse(s); System.out.println(obj); } }
Output
The 2nd element of array {"1":{"2":{"3":{"4":[5,{"6":7}]}}}} Field "1" {"2":{"3":{"4":[5,{"6":7}]}}} {} [5] [5,2]
Advertisements