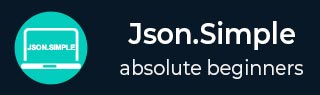
- JSON.simple Tutorial
- JSON.simple - Home
- JSON.simple - Overview
- JSON.simple - Environment Setup
- JSON.simple - JAVA Mapping
- Decoding Examples
- Escaping Special Characters
- JSON.simple - Using JSONValue
- JSON.simple - Exception Handling
- JSON.simple - Container Factory
- JSON.simple - Content Handler
- Encoding Examples
- JSON.simple - Encode JSONObject
- JSON.simple - Encode JSONArray
- Merging Examples
- JSON.simple - Merging Objects
- JSON.simple - Merging Arrays
- Combination Examples
- JSON.simple - Primitive, Object, Array
- JSON.simple - Primitive, Map, List
- Primitive, Object, Map, List
- Customization Examples
- JSON.simple - Customized Output
- Customized Output Streaming
- JSON.simple Useful Resources
- JSON.simple - Quick Guide
- JSON.simple - Useful Resources
- JSON.simple - Discussion
JSON.simple - Quick Guide
JSON.simple - Overview
JSON.simple is a simple Java based toolkit for JSON. You can use JSON.simple to encode or decode JSON data.
Features
Specification Compliant − JSON.simple is fully compliant with JSON Specification - RFC4627.
Lightweight − It have very few classes and provides the necessary functionalities like encode/decode and escaping json.
Reuses Collections − Most of the operations are done using Map/List interfaces increasing the reusablity.
Streaming supported − Supports streaming of JSON output text.
SAX like Content Handler − Provides a SAX-like interface to stream large amount of JSON data.
High performance − Heap based parser is used and provide high performance.
No dependency − No external library dependency. Can be independently included.
JDK1.2 compatible − Source code and the binary are JDK1.2 compatible
JSON.simple - Environment Setup
Local Environment Setup
JSON.simple is a library for Java, so the very first requirement is to have JDK installed in your machine.
System Requirement
JDK | Memory | Disk Space | Operating System |
---|---|---|---|
1.5 or above. | No minimum requirement. | No minimum requirement. | No minimum requirement. |
Step 1: Verify Java Installation in Your Machine
First of all, open the console and execute a java command based on the operating system you are working on.
OS | Task | Command |
---|---|---|
Windows | Open Command Console | c:\> java -version |
Linux | Open Command Terminal | $ java -version |
Mac | Open Terminal | machine:< joseph$ java -version |
Let's verify the output for all the operating systems −
OS | Output |
---|---|
Windows | java version "1.8.0_101" Java(TM) SE Runtime Environment (build 1.8.0_101) |
Linux | java version "1.8.0_101" Java(TM) SE Runtime Environment (build 1.8.0_101) |
Mac | java version "1.8.0_101" Java(TM) SE Runtime Environment (build 1.8.0_101) |
If you do not have Java installed on your system, then download the Java Software Development Kit (SDK) from the following link www.oracle.com. We are assuming Java 1.8.0_101 as the installed version for this tutorial.
Step 2: Set JAVA Environment
Set the JAVA_HOME environment variable to point to the base directory location where Java is installed on your machine. For example.
OS | Output |
---|---|
Windows | Set the environment variable JAVA_HOME to C:\Program Files\Java\jdk1.8.0_101 |
Linux | export JAVA_HOME = /usr/local/java-current |
Mac | export JAVA_HOME = /Library/Java/Home |
Append Java compiler location to the System Path.
OS | Output |
---|---|
Windows | Append the string C:\Program Files\Java\jdk1.8.0_101\bin at the end of the system variable, Path. |
Linux | export PATH = $PATH:$JAVA_HOME/bin/ |
Mac | not required |
Verify Java installation using the command java -version as explained above.
Step 3: Download JSON.simple Archive
Download the latest version of JSON.simple jar file from json-simple @ MVNRepository. At the time of writing this tutorial, we have downloaded json-simple-1.1.1.jar, and copied it into C:\>JSON folder.
OS | Archive name |
---|---|
Windows | json-simple-1.1.1.jar |
Linux | json-simple-1.1.1.jar |
Mac | json-simple-1.1.1.jar |
Step 4: Set JSON_JAVA Environment
Set the JSON_JAVA environment variable to point to the base directory location where JSON.simple jar is stored on your machine. Let's assuming we've stored json-simple-1.1.1.jar in the JSON folder.
Sr.No | OS & Description |
---|---|
1 | Windows Set the environment variable JSON_JAVA to C:\JSON |
2 | Linux export JSON_JAVA = /usr/local/JSON |
3 | Mac export JSON_JAVA = /Library/JSON |
Step 5: Set CLASSPATH Variable
Set the CLASSPATH environment variable to point to the JSON.simple jar location.
Sr.No | OS & Description |
---|---|
1 | Windows Set the environment variable CLASSPATH to %CLASSPATH%;%JSON_JAVA%\json-simple-1.1.1.jar;.; |
2 | Linux export CLASSPATH = $CLASSPATH:$JSON_JAVA/json-simple-1.1.1.jar:. |
3 | Mac export CLASSPATH = $CLASSPATH:$JSON_JAVA/json-simple-1.1.1.jar:. |
JSON.simple - JAVA Mapping
JSON.simple maps entities from the left side to the right side while decoding or parsing, and maps entities from the right to the left while encoding.
JSON | Java |
---|---|
string | java.lang.String |
number | java.lang.Number |
true|false | java.lang.Boolean |
null | null |
array | java.util.List |
object | java.util.Map |
On decoding, the default concrete class of java.util.List is org.json.simple.JSONArray and the default concrete class of java.util.Map is org.json.simple.JSONObject.
JSON.simple - Escaping Special Characters
The following characters are reserved characters and can not be used in JSON and must be properly escaped to be used in strings.
Backspace to be replaced with \b
Form feed to be replaced with \f
Newline to be replaced with \n
Carriage return to be replaced with \r
Tab to be replaced with \t
Double quote to be replaced with \"
Backslash to be replaced with \\
JSONObject.escape() method can be used to escape such reserved keywords in a JSON String. Following is the example −
Example
import org.json.simple.JSONObject; public class JsonDemo { public static void main(String[] args) { JSONObject jsonObject = new JSONObject(); String text = "Text with special character /\"\'\b\f\t\r\n."; System.out.println(text); System.out.println("After escaping."); text = jsonObject.escape(text); System.out.println(text); } }
Output
Text with special character /"' . After escaping. Text with special character \/\"'\b\f\t\r\n.
JSON.simple - Using JSONValue
JSONValue provide a static method parse() to parse the given json string to return a JSONObject which can then be used to get the values parsed. See the example below.
Example
import org.json.simple.JSONArray; import org.json.simple.JSONObject; import org.json.simple.JSONValue; public class JsonDemo { public static void main(String[] args) { String s = "[0,{\"1\":{\"2\":{\"3\":{\"4\":[5,{\"6\":7}]}}}}]"; Object obj = JSONValue.parse(s); JSONArray array = (JSONArray)obj; System.out.println("The 2nd element of array"); System.out.println(array.get(1)); System.out.println(); JSONObject obj2 = (JSONObject)array.get(1); System.out.println("Field \"1\""); System.out.println(obj2.get("1")); s = "{}"; obj = JSONValue.parse(s); System.out.println(obj); s = "[5,]"; obj = JSONValue.parse(s); System.out.println(obj); s = "[5,,2]"; obj = JSONValue.parse(s); System.out.println(obj); } }
Output
The 2nd element of array {"1":{"2":{"3":{"4":[5,{"6":7}]}}}} Field "1" {"2":{"3":{"4":[5,{"6":7}]}}} {} [5] [5,2]
JSON.simple - Exception Handling
JSONParser.parse() throws ParseException in case of invalid JSON. Following example shows how to handle ParseException.
Example
import org.json.simple.parser.JSONParser; import org.json.simple.parser.ParseException; class JsonDemo { public static void main(String[] args) { JSONParser parser = new JSONParser(); String text = "[[null, 123.45, \"a\tb c\"]}, true"; try{ Object obj = parser.parse(text); System.out.println(obj); }catch(ParseException pe) { System.out.println("position: " + pe.getPosition()); System.out.println(pe); } } }
Output
position: 24 Unexpected token RIGHT BRACE(}) at position 24.
JSON.simple - Container Factory
ContainerFactory can be used to create Custom container for parsed JSON objects/arrays. First we need to create a ContainerFactory object and then use it in parse Method of JSONParser to get the required object. See the example below −
Example
import java.util.LinkedHashMap; import java.util.LinkedList; import java.util.List; import java.util.Map; import org.json.simple.parser.ContainerFactory; import org.json.simple.parser.JSONParser; import org.json.simple.parser.ParseException; class JsonDemo { public static void main(String[] args) { JSONParser parser = new JSONParser(); String text = "{\"first\": 123, \"second\": [4, 5, 6], \"third\": 789}"; ContainerFactory containerFactory = new ContainerFactory() { @Override public Map createObjectContainer() { return new LinkedHashMap<>(); } @Override public List creatArrayContainer() { return new LinkedList<>(); } }; try { Map map = (Map)parser.parse(text, containerFactory); map.forEach((k,v)->System.out.println("Key : " + k + " Value : " + v)); } catch(ParseException pe) { System.out.println("position: " + pe.getPosition()); System.out.println(pe); } } }
Output
Key : first Value : 123 Key : second Value : [4, 5, 6] Key : third Value : 789
JSON.simple - ContentHandler
ContentHandler interface is used to provide a SAX like interface to stream the large json. It provides stoppable capability as well. Following example illustrates the concept.
Example
import java.io.IOException; import java.util.List; import java.util.Stack; import org.json.simple.JSONArray; import org.json.simple.JSONObject; import org.json.simple.parser.ContentHandler; import org.json.simple.parser.JSONParser; import org.json.simple.parser.ParseException; class JsonDemo { public static void main(String[] args) { JSONParser parser = new JSONParser(); String text = "{\"first\": 123, \"second\": [4, 5, 6], \"third\": 789}"; try { CustomContentHandler handler = new CustomContentHandler(); parser.parse(text, handler,true); } catch(ParseException pe) { } } } class CustomContentHandler implements ContentHandler { @Override public boolean endArray() throws ParseException, IOException { System.out.println("inside endArray"); return true; } @Override public void endJSON() throws ParseException, IOException { System.out.println("inside endJSON"); } @Override public boolean endObject() throws ParseException, IOException { System.out.println("inside endObject"); return true; } @Override public boolean endObjectEntry() throws ParseException, IOException { System.out.println("inside endObjectEntry"); return true; } public boolean primitive(Object value) throws ParseException, IOException { System.out.println("inside primitive: " + value); return true; } @Override public boolean startArray() throws ParseException, IOException { System.out.println("inside startArray"); return true; } @Override public void startJSON() throws ParseException, IOException { System.out.println("inside startJSON"); } @Override public boolean startObject() throws ParseException, IOException { System.out.println("inside startObject"); return true; } @Override public boolean startObjectEntry(String key) throws ParseException, IOException { System.out.println("inside startObjectEntry: " + key); return true; } }
Output
inside startJSON inside startObject inside startObjectEntry: first inside primitive: 123 inside endObjectEntry inside startObjectEntry: second inside startArray inside primitive: 4 inside primitive: 5 inside primitive: 6 inside endArray inside endObjectEntry inside startObjectEntry: third inside primitive: 789 inside endObjectEntry inside endObject inside endJSON
JSON.simple - Encode JSONObject
Using JSON.simple, we can encode a JSON Object using following ways −
Encode a JSON Object - to String − Simple encoding.
Encode a JSON Object - Streaming − Output can be used for streaming.
Encode a JSON Object - Using Map − Encoding by preserving the order.
Encode a JSON Object - Using Map and Streaming − Encoding by preserving the order and to stream.
Following example illustrates the above concepts.
Example
import java.io.IOException; import java.io.StringWriter; import java.util.LinkedHashMap; import java.util.Map; import org.json.simple.JSONObject; import org.json.simple.JSONValue; class JsonDemo { public static void main(String[] args) throws IOException { JSONObject obj = new JSONObject(); String jsonText; obj.put("name", "foo"); obj.put("num", new Integer(100)); obj.put("balance", new Double(1000.21)); obj.put("is_vip", new Boolean(true)); jsonText = obj.toString(); System.out.println("Encode a JSON Object - to String"); System.out.print(jsonText); StringWriter out = new StringWriter(); obj.writeJSONString(out); jsonText = out.toString(); System.out.println("\nEncode a JSON Object - Streaming"); System.out.print(jsonText); Map obj1 = new LinkedHashMap(); obj1.put("name", "foo"); obj1.put("num", new Integer(100)); obj1.put("balance", new Double(1000.21)); obj1.put("is_vip", new Boolean(true)); jsonText = JSONValue.toJSONString(obj1); System.out.println("\nEncode a JSON Object - Preserving Order"); System.out.print(jsonText); out = new StringWriter(); JSONValue.writeJSONString(obj1, out); jsonText = out.toString(); System.out.println("\nEncode a JSON Object - Preserving Order and Stream"); System.out.print(jsonText); } }
Output
Encode a JSON Object - to String {"balance":1000.21,"is_vip":true,"num":100,"name":"foo"} Encode a JSON Object - Streaming {"balance":1000.21,"is_vip":true,"num":100,"name":"foo"} Encode a JSON Object - Preserving Order {"name":"foo","num":100,"balance":1000.21,"is_vip":true} Encode a JSON Object - Preserving Order and Stream {"name":"foo","num":100,"balance":1000.21,"is_vip":true}
JSON.simple - Encode JSONArray
Using JSON.simple, we can encode a JSON Array using following ways −
Encode a JSON Array - to String − Simple encoding.
Encode a JSON Array - Streaming − Output can be used for streaming.
Encode a JSON Array - Using List − Encoding by using the List.
Encode a JSON Array - Using List and Streaming − Encoding by using List and to stream.
Following example illustrates the above concepts.
Example
import java.io.IOException; import java.io.StringWriter; import java.util.LinkedList; import java.util.List; import org.json.simple.JSONArray; import org.json.simple.JSONValue; class JsonDemo { public static void main(String[] args) throws IOException { JSONArray list = new JSONArray(); String jsonText; list.add("foo"); list.add(new Integer(100)); list.add(new Double(1000.21)); list.add(new Boolean(true)); list.add(null); jsonText = list.toString(); System.out.println("Encode a JSON Array - to String"); System.out.print(jsonText); StringWriter out = new StringWriter(); list.writeJSONString(out); jsonText = out.toString(); System.out.println("\nEncode a JSON Array - Streaming"); System.out.print(jsonText); List list1 = new LinkedList(); list1.add("foo"); list1.add(new Integer(100)); list1.add(new Double(1000.21)); list1.add(new Boolean(true)); list1.add(null); jsonText = JSONValue.toJSONString(list1); System.out.println("\nEncode a JSON Array - Using List"); System.out.print(jsonText); out = new StringWriter(); JSONValue.writeJSONString(list1, out); jsonText = out.toString(); System.out.println("\nEncode a JSON Array - Using List and Stream"); System.out.print(jsonText); } }
Output
Encode a JSON Array - to String ["foo",100,1000.21,true,null] Encode a JSON Array - Streaming ["foo",100,1000.21,true,null] Encode a JSON Array - Using List ["foo",100,1000.21,true,null] Encode a JSON Array - Using List and Stream ["foo",100,1000.21,true,null]
JSON.simple - Merging Objects
In JSON.simple, we can merge two JSON Objects easily using JSONObject.putAll() method.
Following example illustrates the above concept.
Example
import java.io.IOException; import org.json.simple.JSONObject; class JsonDemo { public static void main(String[] args) throws IOException { JSONObject obj1 = new JSONObject(); obj1.put("name", "foo"); obj1.put("num", new Integer(100)); JSONObject obj2 = new JSONObject(); obj2.put("balance", new Double(1000.21)); obj2.put("is_vip", new Boolean(true)); obj1.putAll(obj2); System.out.println(obj1); } }
Output
{"balance":1000.21,"is_vip":true,"num":100,"name":"foo"}
JSON.simple - Merging Arrays
In JSON.simple, we can merge two JSON Arrays easily using JSONArray.addAll() method.
Following example illustrates the above concept.
Example
import java.io.IOException; import org.json.simple.JSONArray; class JsonDemo { public static void main(String[] args) throws IOException { JSONArray list1 = new JSONArray(); list1.add("foo"); list1.add(new Integer(100)); JSONArray list2 = new JSONArray(); list2.add(new Double(1000.21)); list2.add(new Boolean(true)); list2.add(null); list1.addAll(list2); System.out.println(list1); } }
Output
["foo",100,1000.21,true,null]
JSON.simple - Primitive, Object, Array
Using JSONArray object, we can create a JSON which comprises of primitives, object and array.
Following example illustrates the above concept.
Example
import java.io.IOException; import org.json.simple.JSONArray; import org.json.simple.JSONObject; class JsonDemo { public static void main(String[] args) throws IOException { JSONArray list1 = new JSONArray(); list1.add("foo"); list1.add(new Integer(100)); JSONArray list2 = new JSONArray(); list2.add(new Double(1000.21)); list2.add(new Boolean(true)); list2.add(null); JSONObject obj = new JSONObject(); obj.put("name", "foo"); obj.put("num", new Integer(100)); obj.put("balance", new Double(1000.21)); obj.put("is_vip", new Boolean(true)); obj.put("list1", list1); obj.put("list2", list2); System.out.println(obj); } }
Output
{"list1":["foo",100],"balance":1000.21,"is_vip":true,"num":100,"list2":[1000.21,true,null],"name":"foo"}
JSON.simple - Primitive, Map, List Combination
Using JSONValue object, we can create a JSON which comprises of primitives, Map and List.
Following example illustrates the above concept.
Example
import java.io.IOException; import java.util.LinkedHashMap; import java.util.LinkedList; import java.util.List; import java.util.Map; import org.json.simple.JSONValue; class JsonDemo { public static void main(String[] args) throws IOException { Map m1 = new LinkedHashMap(); m1.put("k11","v11"); m1.put("k12","v12"); m1.put("k13", "v13"); List l1 = new LinkedList(); l1.add(m1); l1.add(new Integer(100)); String jsonString = JSONValue.toJSONString(l1); System.out.println(jsonString); } }
Output
[{"k11":"v11","k12":"v12","k13":"v13"},100]
JSON.simple - Primitive, Object, Map, List
Using JSONValue object, we can create a JSON which comprises of primitives, Object, Map and List.
Following example illustrates the above concept.
Example
import java.io.IOException; import java.util.LinkedHashMap; import java.util.LinkedList; import java.util.List; import java.util.Map; import org.json.simple.JSONObject; import org.json.simple.JSONValue; class JsonDemo { public static void main(String[] args) throws IOException { JSONObject obj = new JSONObject(); Map m1 = new LinkedHashMap(); m1.put("k11","v11"); m1.put("k12","v12"); m1.put("k13", "v13"); List l1 = new LinkedList(); l1.add(new Integer(100)); obj.put("m1", m1); obj.put("l1", l1); String jsonString = JSONValue.toJSONString(obj); System.out.println(jsonString); } }
Output
{"m1":{"k11":"v11","k12":"v12","k13":"v13"},"l1":[100]}
JSON.simple - Customized Output
We can customize JSON output based on custom class. Only requirement is to implement JSONAware interface.
Following example illustrates the above concept.
Example
import java.io.IOException; import org.json.simple.JSONArray; import org.json.simple.JSONAware; import org.json.simple.JSONObject; class JsonDemo { public static void main(String[] args) throws IOException { JSONArray students = new JSONArray(); students.add(new Student(1,"Robert")); students.add(new Student(2,"Julia")); System.out.println(students); } } class Student implements JSONAware { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toJSONString() { StringBuilder sb = new StringBuilder(); sb.append("{"); sb.append("name"); sb.append(":"); sb.append("\"" + JSONObject.escape(name) + "\""); sb.append(","); sb.append("rollNo"); sb.append(":"); sb.append(rollNo); sb.append("}"); return sb.toString(); } }
Output
[{name:"Robert",rollNo:1},{name:"Julia",rollNo:2}]
JSON.simple - Customized Output Streaming
We can customize JSON streaming output based on custom class. Only requirement is to implement JSONStreamAware interface.
Following example illustrates the above concept.
Example
import java.io.IOException; import java.io.StringWriter; import java.io.Writer; import java.util.LinkedHashMap; import java.util.Map; import org.json.simple.JSONArray; import org.json.simple.JSONStreamAware; import org.json.simple.JSONValue; class JsonDemo { public static void main(String[] args) throws IOException { JSONArray students = new JSONArray(); students.add(new Student(1,"Robert")); students.add(new Student(2,"Julia")); StringWriter out = new StringWriter(); students.writeJSONString(out); System.out.println(out.toString()); } } class Student implements JSONStreamAware { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public void writeJSONString(Writer out) throws IOException { Map obj = new LinkedHashMap(); obj.put("name", name); obj.put("rollNo", new Integer(rollNo)); JSONValue.writeJSONString(obj, out); } }
Output
[{name:"Robert",rollNo:1},{name:"Julia",rollNo:2}]