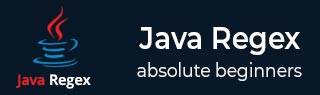
- Java Regex Tutorial
- Java Regex - Home
- Java Regex - Overview
- Java Regex - Capturing Groups
- Java Regex - MatchResult Interface
- Java Regex - Pattern Class
- Java Regex - Matcher Class
- PatternSyntaxException Class
- Java Regex Examples
- Java Regex - Characters
- Java Regex - Character Classes
- Predefined Character Classes
- POSIX Character Classes
- Java Regex - JAVA Character Classes
- Unicode Character Classes
- Java Regex - Boundary Matchers
- Java Regex - Greedy quantifiers
- Java Regex - Reluctant quantifiers
- Java Regex - Possessive quantifiers
- Java Regex - Logical Operators
- Java Regex Useful Resources
- Java Regex - Quick Guide
- Java Regex - Useful Resources
- Java Regex - Discussion
Java Regex - Matcher Class
Introduction
The java.util.regex.Matcher class acts as an engine that performs match operations on a character sequence by interpreting a Pattern.
Class declaration
Following is the declaration for java.util.regex.Matcher class −
public final class Matcher extends Object implements MatchResult
Class methods
Sr.No | Method & Description |
---|---|
1 | Matcher appendReplacement(StringBuffer sb, String replacement)
Implements a non-terminal append-and-replace step. |
2 | StringBuffer appendTail(StringBuffer sb)
Implements a terminal append-and-replace step. |
3 | int end()
Returns the offset after the last character matched. |
4 | int end(int group)
Returns the offset after the last character of the subsequence captured by the given group during the previous match operation. |
5 | boolean find()
Attempts to find the next subsequence of the input sequence that matches the pattern. |
6 | boolean find(int start)
Resets this matcher and then attempts to find the next subsequence of the input sequence that matches the pattern, starting at the specified index. |
7 | String group()
Returns the input subsequence captured by the given group during the previous match operation. |
8 | String group(String name)
Returns the input subsequence captured by the given named-capturing group during the previous match operation. |
9 | int groupCount()
Returns the number of capturing groups in this matcher's pattern. |
10 | boolean hasAnchoringBounds()
Queries the anchoring of region bounds for this matcher. |
11 | boolean hasTransparentBounds()
Queries the transparency of region bounds for this matcher. |
12 | boolean hitEnd()
Returns true if the end of input was hit by the search engine in the last match operation performed by this matcher. |
13 | boolean lookingAt()
Attempts to match the input sequence, starting at the beginning of the region, against the pattern. |
14 | boolean matches()
Attempts to match the entire region against the pattern. |
15 | Pattern pattern()
Returns the pattern that is interpreted by this matcher. |
16 | static String quoteReplacement(String s)
Returns a literal replacement String for the specified String. |
17 | Matcher region(int start, int end)
Sets the limits of this matcher's region. |
18 | int regionEnd()
Reports the end index (exclusive) of this matcher's region. |
19 | int regionStart()
Reports the start index of this matcher's region. |
20 | String replaceAll(String replacement)
Replaces every subsequence of the input sequence that matches the pattern with the given replacement string. |
21 | String replaceFirst(String replacement)
Replaces the first subsequence of the input sequence that matches the pattern with the given replacement string. |
22 | boolean requireEnd()
Returns true if more input could change a positive match into a negative one. |
23 | Matcher reset()
Resets this matcher. |
24 | Matcher reset(CharSequence input)
Resets this matcher with a new input sequence. |
25 | int start()
Returns the start index of the previous match. |
26 | int start(int group)
Returns the start index of the subsequence captured by the given group during the previous match operation. |
27 | MatchResult toMatchResult()
Returns the match state of this matcher as a MatchResult. |
28 | String toString()
Returns the string representation of this matcher. |
29 | Matcher useAnchoringBounds(boolean b)
Sets the anchoring of region bounds for this matcher. |
30 | Matcher usePattern(Pattern newPattern)
Changes the Pattern that this Matcher uses to find matches with. |
31 | Matcher useTransparentBounds(boolean b)
Sets the transparency of region bounds for this matcher. |
Methods inherited
This class inherits methods from the following classes −
- Java.lang.Object