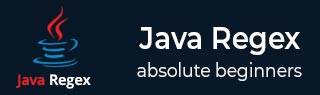
- Java Regex Tutorial
- Java Regex - Home
- Java Regex - Overview
- Java Regex - Capturing Groups
- Java Regex - MatchResult Interface
- Java Regex - Pattern Class
- Java Regex - Matcher Class
- PatternSyntaxException Class
- Java Regex Examples
- Java Regex - Characters
- Java Regex - Character Classes
- Predefined Character Classes
- POSIX Character Classes
- Java Regex - JAVA Character Classes
- Unicode Character Classes
- Java Regex - Boundary Matchers
- Java Regex - Greedy quantifiers
- Java Regex - Reluctant quantifiers
- Java Regex - Possessive quantifiers
- Java Regex - Logical Operators
- Java Regex Useful Resources
- Java Regex - Quick Guide
- Java Regex - Useful Resources
- Java Regex - Discussion
java.util.regex.Matcher.usePattern() Method
Description
The java.util.regex.Matcher.usePattern(Pattern newPattern) method changes the Pattern that this Matcher uses to find matches with.
Declaration
Following is the declaration for java.util.regex.Matcher.usePattern(Pattern newPattern) method.
public Matcher usePattern(Pattern newPattern)
Parameters
newPattern − The new pattern used by this matcher.
Return Value
this matcher.
Exceptions
IllegalArgumentException − If newPattern is null.
Example
The following example shows the usage of java.util.regex.Matcher.usePattern(Pattern newPattern) method.
package com.tutorialspoint; import java.util.regex.Matcher; import java.util.regex.Pattern; public class MatcherDemo { private static String REGEX = "(a*b)(foo)"; private static String INPUT = "aabfooaabfooabfoob"; public static void main(String[] args) { // create a pattern Pattern pattern = Pattern.compile(REGEX); // get a matcher object Matcher matcher = pattern.matcher(INPUT); while(matcher.find()) { //Prints the start index of the subsequence captured by the given group. System.out.println("Second Capturing Group, (foo) Match String start(): "+matcher.start(1)); } matcher.reset(); matcher.usePattern(Pattern.compile("(a*b)(foob)")); while(matcher.find()) { //Prints the start index of the subsequence captured by the given group. System.out.println("Second Capturing Group, (fooab) Match String start(): "+matcher.start(1)); } } }
Let us compile and run the above program, this will produce the following result −
Second Capturing Group, (foo) Match String start(): 0 Second Capturing Group, (foo) Match String start(): 6 Second Capturing Group, (foo) Match String start(): 12 Second Capturing Group, (fooab) Match String start(): 12
javaregex_matcher.htm
Advertisements