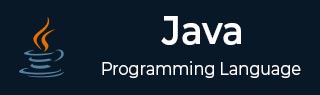
Java Tutorial
- Java - Home
- Java - Overview
- Java - History
- Java - Features
- Java vs C++
- Java Virtual Machine(JVM)
- Java - JDK vs JRE vs JVM
- Java - Hello World Program
- Java - Environment Setup
- Java - Basic Syntax
- Java - Variable Types
- Java - Data Types
- Java - Type Casting
- Java - Unicode System
- Java - Basic Operators
- Java - Comments
- Java - User Input
Java Control Statements
- Java - Loop Control
- Java - Decision Making
- Java - If-else
- Java - Switch
- Java - For Loops
- Java - For-Each Loops
- Java - While Loops
- Java - do-while Loops
- Java - Break
- Java - Continue
Object Oriented Programming
- Java - OOPs Concepts
- Java - Object & Classes
- Java - Class Attributes
- Java - Class Methods
- Java - Methods
- Java - Variables Scope
- Java - Constructors
- Java - Access Modifiers
- Java - Inheritance
- Java - Aggregation
- Java - Polymorphism
- Java - Overriding
- Java - Method Overloading
- Java - Dynamic Binding
- Java - Static Binding
- Java - Instance Initializer Block
- Java - Abstraction
- Java - Encapsulation
- Java - Interfaces
- Java - Packages
- Java - Inner Classes
- Java - Static Class
- Java - Anonymous Class
- Java - Singleton Class
- Java - Wrapper Classes
- Java - Enums
- Java - Enum Constructor
- Java - Enum Strings
Java Built-in Classes
Java File Handling
- Java - Files
- Java - Create a File
- Java - Write to File
- Java - Read Files
- Java - Delete Files
- Java - Directories
- Java - I/O Streams
Java Error & Exceptions
- Java - Exceptions
- Java - try-catch Block
- Java - try-with-resources
- Java - Multi-catch Block
- Java - Nested try Block
- Java - Finally Block
- Java - throw Exception
- Java - Exception Propagation
- Java - Built-in Exceptions
- Java - Custom Exception
Java Multithreading
- Java - Multithreading
- Java - Thread Life Cycle
- Java - Creating a Thread
- Java - Starting a Thread
- Java - Joining Threads
- Java - Naming Thread
- Java - Thread Scheduler
- Java - Thread Pools
- Java - Main Thread
- Java - Thread Priority
- Java - Daemon Threads
- Java - Thread Group
- Java - Shutdown Hook
Java Synchronization
- Java - Synchronization
- Java - Block Synchronization
- Java - Static Synchronization
- Java - Inter-thread Communication
- Java - Thread Deadlock
- Java - Interrupting a Thread
- Java - Thread Control
- Java - Reentrant Monitor
Java Networking
- Java - Networking
- Java - Socket Programming
- Java - URL Processing
- Java - URL Class
- Java - URLConnection Class
- Java - HttpURLConnection Class
- Java - Socket Class
- Java - Generics
Java Collections
Java List Interface
Java Queue Interface
Java Map Interface
- Java - Map Interface
- Java - HashMap
- Java - LinkedHashMap
- Java - WeakHashMap
- Java - EnumMap
- Java - SortedMap Interface
- Java - TreeMap
- Java - The IdentityHashMap Class
Java Set Interface
- Java - Set Interface
- Java - HashSet
- Java - EnumSet
- Java - LinkedHashSet
- Java - SortedSet Interface
- Java - TreeSet
Java Data Structures
- Java - Data Structures
- Java - Enumeration
- Java - BitSet Class
- Java - Dictionary
- Java - Hashtable
- Java - Properties
Java Collections Algorithms
Advanced Java
- Java - Command-Line Arguments
- Java - Lambda Expressions
- Java - Sending Email
- Java - Applet Basics
- Java - Javadoc Comments
- Java - Autoboxing and Unboxing
- Java - File Mismatch Method
- Java - REPL (JShell)
- Java - Multi-Release Jar Files
- Java - Private Interface Methods
- Java - Inner Class Diamond Operator
- Java - Multiresolution Image API
- Java - Collection Factory Methods
- Java - Module System
- Java - Nashorn JavaScript
- Java - Optional Class
- Java - Method References
- Java - Functional Interfaces
- Java - Default Methods
- Java - Base64 Encode Decode
- Java - Switch Expressions
- Java - Teeing Collectors
- Java - Microbenchmark
- Java - Text Blocks
- Java - Null Pointer Exception
- Java - Packaging Tools
- Java - Sealed Classes
- Java - Record Classes
- Java - Hidden Classes
- Java - Compact Number Formatting
Java Miscellaneous
- Java - Recursion
- Java - Regular Expressions
- Java - Serialization
- Java - Strings
- Java - Process API Improvements
- Java - Stream API Improvements
- Java - Enhanced @Deprecated Annotation
- Java - CompletableFuture API Improvements
- Java - Array Methods
- Java - Streams
- Java - Datetime Api
- Java 8 - New Features
- Java 9 - New Features
Java APIs & Frameworks
Java Useful Resources
Java - throw keyword
An exception (or exceptional event) is a problem that arises during the execution of a program. When an Exception occurs the normal flow of the program is disrupted and the program/Application terminates abnormally, which is not recommended, therefore, these exceptions are to be handled.
An exception can occur for many different reasons. Following are some scenarios where an exception occurs.
A user has entered an invalid data.
A file that needs to be opened cannot be found.
A network connection has been lost in the middle of communications or the JVM has run out of memory.
Some of these exceptions are caused by user error, others by programmer error, and others by physical resources that have failed in some manner.
The Throws/Throw Keywords
If a method does not handle a checked exception, the method must declare it using the throws keyword. The throws keyword appears at the end of a method's signature.
You can throw an exception, either a newly instantiated one or an exception that you just caught, by using the throw keyword.
Try to understand the difference between throws and throw keywords, throws is used to postpone the handling of a checked exception and throw is used to invoke an exception explicitly.
The following method declares that it throws a RemoteException −
Syntax
public static void accesElement() throws CustomException { try { int a[] = new int[2]; System.out.println("Access element three :" + a[3]); } catch(Exception e) { throw new CustomException(e); } }
Example
In this example, we're calling a method accessElement() method which has declared to throw CustomException. So we need to write a try-catch block over accessElement() method. When program runs, it throws exception and it is printed.
// File Name : ExcepTest.java package com.tutorialspoint; public class ExcepTest { public static void main(String args[]) { try { accesElement(); } catch (CustomException e) { e.printStackTrace(); } System.out.println("Out of the block"); } public static void accesElement() throws CustomException { try { int a[] = new int[2]; System.out.println("Access element three :" + a[3]); } catch(Exception e) { throw new CustomException(e); } } } class CustomException extends Exception{ private static final long serialVersionUID = 1L; CustomException(Exception e){ super(e); } }
Output
com.tutorialspoint.CustomException: java.lang.ArrayIndexOutOfBoundsException: 3 at com.tutorialspoint.ExcepTest.accesElement(ExcepTest.java:20) at com.tutorialspoint.ExcepTest.main(ExcepTest.java:8) Caused by: java.lang.ArrayIndexOutOfBoundsException: 3 at com.tutorialspoint.ExcepTest.accesElement(ExcepTest.java:18) ... 1 more Out of the block
Following is another example showcasing use of throws keyword.
Example
In this example, we're calling a method accessElement() method which has declared to throw CustomException. So we are not having try-catch block over accessElement() method, the main method declares to throw the exception. When program runs, it throws exception and it is printed.
// File Name : ExcepTest.java package com.tutorialspoint; public class ExcepTest { public static void main(String args[]) throws CustomException { accesElement(); System.out.println("Out of the block"); } public static void accesElement() throws CustomException { try { int a[] = new int[2]; System.out.println("Access element three :" + a[3]); } catch(Exception e) { throw new CustomException(e); } } } class CustomException extends Exception{ private static final long serialVersionUID = 1L; CustomException(Exception e){ super(e); } }
Output
Exception in thread "main" com.tutorialspoint.CustomException: java.lang.ArrayIndexOutOfBoundsException: 3 at com.tutorialspoint.ExcepTest.accesElement(ExcepTest.java:16) at com.tutorialspoint.ExcepTest.main(ExcepTest.java:7) Caused by: java.lang.ArrayIndexOutOfBoundsException: 3 at com.tutorialspoint.ExcepTest.accesElement(ExcepTest.java:14) ... 1 more
As exception is not handled, last statement is not executed in this example.
A method can declare that it throws more than one exception, in which case the exceptions are declared in a list separated by commas. For example, the following method declares that it throws a RemoteException and an InsufficientFundsException −
Example
import java.io.*; public class className { public void withdraw(double amount) throws RemoteException, InsufficientFundsException { // Method implementation } // Remainder of class definition }
To Continue Learning Please Login