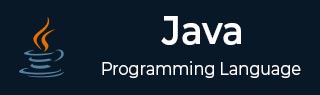
Java Tutorial
- Java - Home
- Java - Overview
- Java - History
- Java - Features
- Java vs C++
- Java Virtual Machine(JVM)
- Java - JDK vs JRE vs JVM
- Java - Hello World Program
- Java - Environment Setup
- Java - Basic Syntax
- Java - Variable Types
- Java - Data Types
- Java - Type Casting
- Java - Unicode System
- Java - Basic Operators
- Java - Comments
- Java - User Input
Java Control Statements
- Java - Loop Control
- Java - Decision Making
- Java - If-else
- Java - Switch
- Java - For Loops
- Java - For-Each Loops
- Java - While Loops
- Java - do-while Loops
- Java - Break
- Java - Continue
Object Oriented Programming
- Java - OOPs Concepts
- Java - Object & Classes
- Java - Class Attributes
- Java - Class Methods
- Java - Methods
- Java - Variables Scope
- Java - Constructors
- Java - Access Modifiers
- Java - Inheritance
- Java - Aggregation
- Java - Polymorphism
- Java - Overriding
- Java - Method Overloading
- Java - Dynamic Binding
- Java - Static Binding
- Java - Instance Initializer Block
- Java - Abstraction
- Java - Encapsulation
- Java - Interfaces
- Java - Packages
- Java - Inner Classes
- Java - Static Class
- Java - Anonymous Class
- Java - Singleton Class
- Java - Wrapper Classes
- Java - Enums
- Java - Enum Constructor
- Java - Enum Strings
Java Built-in Classes
Java File Handling
- Java - Files
- Java - Create a File
- Java - Write to File
- Java - Read Files
- Java - Delete Files
- Java - Directories
- Java - I/O Streams
Java Error & Exceptions
- Java - Exceptions
- Java - try-catch Block
- Java - try-with-resources
- Java - Multi-catch Block
- Java - Nested try Block
- Java - Finally Block
- Java - throw Exception
- Java - Exception Propagation
- Java - Built-in Exceptions
- Java - Custom Exception
Java Multithreading
- Java - Multithreading
- Java - Thread Life Cycle
- Java - Creating a Thread
- Java - Starting a Thread
- Java - Joining Threads
- Java - Naming Thread
- Java - Thread Scheduler
- Java - Thread Pools
- Java - Main Thread
- Java - Thread Priority
- Java - Daemon Threads
- Java - Thread Group
- Java - Shutdown Hook
Java Synchronization
- Java - Synchronization
- Java - Block Synchronization
- Java - Static Synchronization
- Java - Inter-thread Communication
- Java - Thread Deadlock
- Java - Interrupting a Thread
- Java - Thread Control
- Java - Reentrant Monitor
Java Networking
- Java - Networking
- Java - Socket Programming
- Java - URL Processing
- Java - URL Class
- Java - URLConnection Class
- Java - HttpURLConnection Class
- Java - Socket Class
- Java - Generics
Java Collections
Java List Interface
Java Queue Interface
Java Map Interface
- Java - Map Interface
- Java - HashMap
- Java - LinkedHashMap
- Java - WeakHashMap
- Java - EnumMap
- Java - SortedMap Interface
- Java - TreeMap
- Java - The IdentityHashMap Class
Java Set Interface
- Java - Set Interface
- Java - HashSet
- Java - EnumSet
- Java - LinkedHashSet
- Java - SortedSet Interface
- Java - TreeSet
Java Data Structures
- Java - Data Structures
- Java - Enumeration
- Java - BitSet Class
- Java - Dictionary
- Java - Hashtable
- Java - Properties
Java Collections Algorithms
Advanced Java
- Java - Command-Line Arguments
- Java - Lambda Expressions
- Java - Sending Email
- Java - Applet Basics
- Java - Javadoc Comments
- Java - Autoboxing and Unboxing
- Java - File Mismatch Method
- Java - REPL (JShell)
- Java - Multi-Release Jar Files
- Java - Private Interface Methods
- Java - Inner Class Diamond Operator
- Java - Multiresolution Image API
- Java - Collection Factory Methods
- Java - Module System
- Java - Nashorn JavaScript
- Java - Optional Class
- Java - Method References
- Java - Functional Interfaces
- Java - Default Methods
- Java - Base64 Encode Decode
- Java - Switch Expressions
- Java - Teeing Collectors
- Java - Microbenchmark
- Java - Text Blocks
- Java - Null Pointer Exception
- Java - Packaging Tools
- Java - Sealed Classes
- Java - Record Classes
- Java - Hidden Classes
- Java - Compact Number Formatting
Java Miscellaneous
- Java - Recursion
- Java - Regular Expressions
- Java - Serialization
- Java - Strings
- Java - Process API Improvements
- Java - Stream API Improvements
- Java - Enhanced @Deprecated Annotation
- Java - CompletableFuture API Improvements
- Java - Array Methods
- Java - Streams
- Java - Datetime Api
- Java 8 - New Features
- Java 9 - New Features
Java APIs & Frameworks
Java Useful Resources
Java - Socket isInputShutdown() Method
Description
The Java Socket isInputShutdown() returns whether the read-half of the socket connection is closed.
Declaration
Following is the declaration for java.net.Socket.isInputShutdown() method.
public boolean isInputShutdown()
Parameters
NA
Return Value
true if the input of the socket has been shutdown.
Exception
NA
Example 1
The following example shows the usage of Java Socket isInputShutdown() method to get the status of read-half of the socket connection to be closed or not. As first step, we've created a Socket instance using no argument constructor. Then in order to create a SocketAddress object, we've initialized an InetAddress instance of localhost address. Using InetSocketAddress object, we've created a SocketAddress object and then using bind() method, we bind the address to the socket. Once done, we're printing the status using isInputShutdown() method, local port and inetaddress as shown. In the end, we closed the socket using close() method.
package com.tutorialspoint; import java.io.IOException; import java.net.InetAddress; import java.net.InetSocketAddress; import java.net.Socket; import java.net.SocketAddress; public class SocketDemo { public static void main(String[] args) throws IOException { Socket socket = new Socket(); InetAddress inetAddress=InetAddress.getByName("localhost"); SocketAddress socketAddress=new InetSocketAddress(inetAddress, 6066); socket.bind(socketAddress); System.out.println("Is Input Stream shutdown: "+socket.isInputShutdown()); System.out.println("Port number: "+socket.getLocalPort()); System.out.println("Inet Address: "+socket.getInetAddress()); socket.close(); } }
Output
Let us compile and run the above program, this will produce the following result −
Is Input Stream shutdown: false Port number: 6066 Inet Address: null
Example 2
The following example shows the usage of Java Socket isInputShutdown() method to get the status of read-half of the socket connection to be closed or not. As first step, we've created a Socket instance using no argument constructor. Then in order to create a SocketAddress object, we've initialized an InetAddress instance of localhost address. Using InetSocketAddress object, we've created a SocketAddress object and then using bind() method, we bind the address to the socket. Using connect() method, we're establishing the connetion to the server. Now, we're printing the status using isInputShutdown() method. Then we're closing the input stream using shutdownInput() method and print the the status using isInputShutdown() method. In the end, we closed the socket using close() method.
package com.tutorialspoint; import java.io.IOException; import java.net.InetAddress; import java.net.InetSocketAddress; import java.net.Socket; import java.net.SocketAddress; public class SocketDemo { public static void main(String[] args) throws IOException { Socket socket = new Socket(); InetAddress inetAddress=InetAddress.getByName("localhost"); SocketAddress socketAddress=new InetSocketAddress(inetAddress, 6067); socket.bind(socketAddress); socket.connect(socketAddress); System.out.println("Is Input Stream shutdown: "+socket.isInputShutdown()); socket.shutdownInput(); System.out.println("Is Input Stream shutdown: "+socket.isInputShutdown()); socket.close(); } }
Output
Let us compile and run the above program, this will produce the following result −
Is Input Stream shutdown: false Is Input Stream shutdown: true
Example 3
The following example shows the usage of Java Socket isInputShutdown() method to get the status of TCP_NODELAY flag of socket instance, if socket is already closed. As first step, we've created a Socket instance using no argument constructor. Then in order to create a SocketAddress object, we've initialized an InetAddress instance of localhost address. Using InetSocketAddress object, we've created a SocketAddress object and then using bind() method, we bind the address to the socket. Using connect() method, we're establishing the connetion to the server.Using socket.close(), we're closing the socket. Now, we're printing the status using isInputShutdown() method.Then we're closing the input stream using shutdownInput() method and print the the status using isInputShutdown() method. In the end, we closed the socket using close() method.
package com.tutorialspoint; import java.io.IOException; import java.net.InetAddress; import java.net.InetSocketAddress; import java.net.Socket; import java.net.SocketAddress; public class SocketDemo { public static void main(String[] args) throws IOException { Socket socket = new Socket(); InetAddress inetAddress=InetAddress.getByName("localhost"); SocketAddress socketAddress=new InetSocketAddress(inetAddress, 6067); socket.bind(socketAddress); socket.connect(socketAddress); socket.close(); System.out.println("Is Input Stream shutdown: "+socket.isInputShutdown()); socket.shutdownInput(); System.out.println("Is Input Stream shutdown: "+socket.isInputShutdown()); socket.close(); } }
Output
Let us compile and run the above program, this will produce the following result −
Is Input Stream shutdown: false Exception in thread "main" java.net.SocketException: Socket is closed at java.base/java.net.Socket.shutdownInput(Socket.java:1538) at com.tutorialspoint.SocketDemo.main(SocketDemo.java:18)
To Continue Learning Please Login