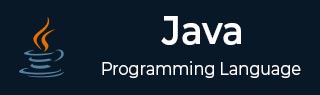
Java Tutorial
- Java - Home
- Java - Overview
- Java - History
- Java - Features
- Java vs C++
- Java Virtual Machine(JVM)
- Java - JDK vs JRE vs JVM
- Java - Hello World Program
- Java - Environment Setup
- Java - Basic Syntax
- Java - Variable Types
- Java - Data Types
- Java - Type Casting
- Java - Unicode System
- Java - Basic Operators
- Java - Comments
- Java - User Input
Java Control Statements
- Java - Loop Control
- Java - Decision Making
- Java - If-else
- Java - Switch
- Java - For Loops
- Java - For-Each Loops
- Java - While Loops
- Java - do-while Loops
- Java - Break
- Java - Continue
Object Oriented Programming
- Java - OOPs Concepts
- Java - Object & Classes
- Java - Class Attributes
- Java - Class Methods
- Java - Methods
- Java - Variables Scope
- Java - Constructors
- Java - Access Modifiers
- Java - Inheritance
- Java - Aggregation
- Java - Polymorphism
- Java - Overriding
- Java - Method Overloading
- Java - Dynamic Binding
- Java - Static Binding
- Java - Instance Initializer Block
- Java - Abstraction
- Java - Encapsulation
- Java - Interfaces
- Java - Packages
- Java - Inner Classes
- Java - Static Class
- Java - Anonymous Class
- Java - Singleton Class
- Java - Wrapper Classes
- Java - Enums
- Java - Enum Constructor
- Java - Enum Strings
Java Built-in Classes
Java File Handling
- Java - Files
- Java - Create a File
- Java - Write to File
- Java - Read Files
- Java - Delete Files
- Java - Directories
- Java - I/O Streams
Java Error & Exceptions
- Java - Exceptions
- Java - try-catch Block
- Java - try-with-resources
- Java - Multi-catch Block
- Java - Nested try Block
- Java - Finally Block
- Java - throw Exception
- Java - Exception Propagation
- Java - Built-in Exceptions
- Java - Custom Exception
Java Multithreading
- Java - Multithreading
- Java - Thread Life Cycle
- Java - Creating a Thread
- Java - Starting a Thread
- Java - Joining Threads
- Java - Naming Thread
- Java - Thread Scheduler
- Java - Thread Pools
- Java - Main Thread
- Java - Thread Priority
- Java - Daemon Threads
- Java - Thread Group
- Java - Shutdown Hook
Java Synchronization
- Java - Synchronization
- Java - Block Synchronization
- Java - Static Synchronization
- Java - Inter-thread Communication
- Java - Thread Deadlock
- Java - Interrupting a Thread
- Java - Thread Control
- Java - Reentrant Monitor
Java Networking
- Java - Networking
- Java - Socket Programming
- Java - URL Processing
- Java - URL Class
- Java - URLConnection Class
- Java - HttpURLConnection Class
- Java - Socket Class
- Java - Generics
Java Collections
Java List Interface
Java Queue Interface
Java Map Interface
- Java - Map Interface
- Java - HashMap
- Java - LinkedHashMap
- Java - WeakHashMap
- Java - EnumMap
- Java - SortedMap Interface
- Java - TreeMap
- Java - The IdentityHashMap Class
Java Set Interface
- Java - Set Interface
- Java - HashSet
- Java - EnumSet
- Java - LinkedHashSet
- Java - SortedSet Interface
- Java - TreeSet
Java Data Structures
- Java - Data Structures
- Java - Enumeration
- Java - BitSet Class
- Java - Dictionary
- Java - Hashtable
- Java - Properties
Java Collections Algorithms
Advanced Java
- Java - Command-Line Arguments
- Java - Lambda Expressions
- Java - Sending Email
- Java - Applet Basics
- Java - Javadoc Comments
- Java - Autoboxing and Unboxing
- Java - File Mismatch Method
- Java - REPL (JShell)
- Java - Multi-Release Jar Files
- Java - Private Interface Methods
- Java - Inner Class Diamond Operator
- Java - Multiresolution Image API
- Java - Collection Factory Methods
- Java - Module System
- Java - Nashorn JavaScript
- Java - Optional Class
- Java - Method References
- Java - Functional Interfaces
- Java - Default Methods
- Java - Base64 Encode Decode
- Java - Switch Expressions
- Java - Teeing Collectors
- Java - Microbenchmark
- Java - Text Blocks
- Java - Null Pointer Exception
- Java - Packaging Tools
- Java - Sealed Classes
- Java - Record Classes
- Java - Hidden Classes
- Java - Compact Number Formatting
Java Miscellaneous
- Java - Recursion
- Java - Regular Expressions
- Java - Serialization
- Java - Strings
- Java - Process API Improvements
- Java - Stream API Improvements
- Java - Enhanced @Deprecated Annotation
- Java - CompletableFuture API Improvements
- Java - Array Methods
- Java - Streams
- Java - Datetime Api
- Java 8 - New Features
- Java 9 - New Features
Java APIs & Frameworks
Java Useful Resources
Java - HttpURLConnection setChunkedStreamingMode()
The Java HttpURLConnection setChunkedStreamingMode() method enables streaming of a HTTP request body without internal buffering, when the content length is not known in advance. In this mode, chunked transfer encoding is used to send the request body. Note, not all HTTP servers support this mode.
When output streaming is enabled, authentication and redirection cannot be handled automatically. A HttpRetryException will be thrown when reading the response if authentication or redirection are required. This exception can be queried for the details of the error. This method must be called before the URLConnection is connected.
Declaration
Following is the declaration for java.net.HttpURLConnection.setChunkedStreamingMode() method
public void setChunkedStreamingMode(int chunklen)
Parameters
chunklen − The number of bytes to write in each chunk. If chunklen is less than or equal to zero, a default value will be used.
Return Value
NA
Exception
IllegalStateException − if URLConnection is already connected or if a different streaming mode is already enabled.
Example 1
The following example shows the usage of Java HttpURLConnection setChunkedStreamingMode() method for a valid url with https protocol. In this example, we're creating an instance of URL class. Using url.openConnection() method, we're getting the HttpURLConnection instance. Using getInputStream(), we're getting the content of the website home page and printing the same −
package com.tutorialspoint; import java.io.BufferedReader; import java.io.IOException; import java.io.InputStreamReader; import java.net.HttpURLConnection; import java.net.URL; public class HttpUrlConnectionDemo { public static void main(String [] args) { try { URL url = new URL("https://www.tutorialspoint.com/index1.htm?language=en#j2se"); HttpURLConnection urlConnection = (HttpURLConnection) url.openConnection(); urlConnection.setChunkedStreamingMode(1000); urlConnection.setDoOutput(true); urlConnection.connect(); System.out.println("Connected."); if(urlConnection.getInputStream() != null) { BufferedReader in = new BufferedReader( new InputStreamReader(urlConnection.getInputStream())); String content = ""; String current; while((current = in.readLine()) != null) { content += current; } System.out.println(content); }else { System.out.println("Input Stream is null"); } urlConnection.disconnect(); System.out.println("Disconnected."); } catch (IOException e) { e.printStackTrace(); } } }
Let us compile and run the above program, this will produce the following result −
Output
Connected. <!DOCTYPE html><html lang="en"><head><title>Online Tutorials Library.... Disconnected.
Example 2
The following example shows the usage of Java HttpURLConnection setChunkedStreamingMode() method for a valid url with http protocol. In this example, we're creating an instance of URL class. Using url.openConnection() method, we're getting the HttpURLConnection instance. Using getInputStream(), we're getting the content of the website home page and printing the same −
package com.tutorialspoint; import java.io.BufferedReader; import java.io.IOException; import java.io.InputStreamReader; import java.net.HttpURLConnection; import java.net.URL; public class HttpUrlConnectionDemo { public static void main(String [] args) { try { URL url = new URL("http://www.tutorialspoint.com/index1.htm?language=en#j2se"); HttpURLConnection urlConnection = (HttpURLConnection) url.openConnection(); urlConnection.setChunkedStreamingMode(1000); urlConnection.setDoOutput(true); urlConnection.connect(); System.out.println("Connected."); if(urlConnection.getInputStream() != null) { BufferedReader in = new BufferedReader( new InputStreamReader(urlConnection.getInputStream())); String content = ""; String current; while((current = in.readLine()) != null) { content += current; } System.out.println(content); }else { System.out.println("Input Stream is null"); } urlConnection.disconnect(); System.out.println("Disconnected."); } catch (IOException e) { e.printStackTrace(); } } }
Let us compile and run the above program, this will produce the following result −
Output
Connected. <!DOCTYPE html><html lang="en"><head><title>Online Tutorials Library.... Disconnected.
Example 3
The following example shows the usage of Java HttpURLConnection setChunkedStreamingMode() method for a valid url with http protocol. In this example, we're creating an instance of URL class. Using url.openConnection() method, we're getting the HttpURLConnection instance. Using getInputStream(), we're getting the content of the website home page and printing the same −
package com.tutorialspoint; import java.io.BufferedReader; import java.io.IOException; import java.io.InputStreamReader; import java.net.HttpURLConnection; import java.net.URL; public class HttpUrlConnectionDemo { public static void main(String [] args) { try { URL url = new URL("http://www.google.com"); HttpURLConnection urlConnection = (HttpURLConnection) url.openConnection(); urlConnection.setChunkedStreamingMode(1000); urlConnection.setDoOutput(true); urlConnection.connect(); System.out.println("Connected."); if(urlConnection.getInputStream() != null) { BufferedReader in = new BufferedReader( new InputStreamReader(urlConnection.getInputStream())); String content = ""; String current; while((current = in.readLine()) != null) { content += current; } System.out.println(content); }else { System.out.println("Input Stream is null"); } urlConnection.disconnect(); System.out.println("Disconnected."); } catch (IOException e) { e.printStackTrace(); } } }
Let us compile and run the above program, this will produce the following result −
Output
Connected. java.io.IOException: Server returned HTTP response code: 405 for URL: http://www.google.com at sun.net.www.protocol.http.HttpURLConnection.getInputStream0(Unknown Source) at sun.net.www.protocol.http.HttpURLConnection.getInputStream(Unknown Source) at com.tutorialspoint.HttpUrlConnectionDemo.main(HttpUrlConnectionDemo.java:18)
To Continue Learning Please Login