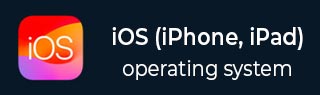
- iOS Tutorial
- iOS - Home
- iOS - Getting Started
- iOS - Environment Setup
- iOS - Objective-C Basics
- iOS - First iPhone Application
- iOS - Actions and Outlets
- iOS - Delegates
- iOS - UI Elements
- iOS - Accelerometer
- iOS - Universal Applications
- iOS - Camera Management
- iOS - Location Handling
- iOS - SQLite Database
- iOS - Sending Email
- iOS - Audio & Video
- iOS - File Handling
- iOS - Accessing Maps
- iOS - In-App Purchase
- iOS - iAd Integration
- iOS - GameKit
- iOS - Storyboards
- iOS - Auto Layouts
- iOS - Twitter & Facebook
- iOS - Memory Management
- iOS - Application Debugging
- iOS Useful Resources
- iOS - Quick Guide
- iOS - Useful Resources
- iOS - Discussion
iOS - Accessing Maps
Maps are always helpful for us to locate places. Maps are integrated in iOS using the MapKit framework.
Steps Involved
Step 1 − Create a simple view-based application.
Step 2 − Select your project file, then select targets and then add MapKit.framework.
Step 3 − We should also add Corelocation.framework.
Step 4 − Add a MapView to ViewController.xib and create an ibOutlet and name it as mapView.
Step 5 − Create a new file by selecting File → New → File... → select Objective C class and click next.
Step 6 − Name the class as MapAnnotation with "sub class of" as NSObject.
Step 7 − Select create.
Step 8 − Update MapAnnotation.h as follows −
#import <Foundation/Foundation.h> #import <MapKit/MapKit.h> @interface MapAnnotation : NSObject<MKAnnotation> @property (nonatomic, strong) NSString *title; @property (nonatomic, readwrite) CLLocationCoordinate2D coordinate; - (id)initWithTitle:(NSString *)title andCoordinate: (CLLocationCoordinate2D)coordinate2d; @end
Step 9 − Update MapAnnotation.m as follows −
#import "MapAnnotation.h" @implementation MapAnnotation -(id)initWithTitle:(NSString *)title andCoordinate: (CLLocationCoordinate2D)coordinate2d { self.title = title; self.coordinate =coordinate2d; return self; } @end
Step 10 − Update ViewController.h as follows −
#import <UIKit/UIKit.h> #import <MapKit/MapKit.h> #import <CoreLocation/CoreLocation.h> @interface ViewController : UIViewController<MKMapViewDelegate> { MKMapView *mapView; } @end
Step 11 − Update ViewController.m as follows −
#import "ViewController.h" #import "MapAnnotation.h" @interface ViewController () @end @implementation ViewController - (void)viewDidLoad { [super viewDidLoad]; mapView = [[MKMapView alloc]initWithFrame: CGRectMake(10, 100, 300, 300)]; mapView.delegate = self; mapView.centerCoordinate = CLLocationCoordinate2DMake(37.32, -122.03); mapView.mapType = MKMapTypeHybrid; CLLocationCoordinate2D location; location.latitude = (double) 37.332768; location.longitude = (double) -122.030039; // Add the annotation to our map view MapAnnotation *newAnnotation = [[MapAnnotation alloc] initWithTitle:@"Apple Head quaters" andCoordinate:location]; [mapView addAnnotation:newAnnotation]; CLLocationCoordinate2D location2; location2.latitude = (double) 37.35239; location2.longitude = (double) -122.025919; MapAnnotation *newAnnotation2 = [[MapAnnotation alloc] initWithTitle:@"Test annotation" andCoordinate:location2]; [mapView addAnnotation:newAnnotation2]; [self.view addSubview:mapView]; } // When a map annotation point is added, zoom to it (1500 range) - (void)mapView:(MKMapView *)mv didAddAnnotationViews:(NSArray *)views { MKAnnotationView *annotationView = [views objectAtIndex:0]; id <MKAnnotation> mp = [annotationView annotation]; MKCoordinateRegion region = MKCoordinateRegionMakeWithDistance ([mp coordinate], 1500, 1500); [mv setRegion:region animated:YES]; [mv selectAnnotation:mp animated:YES]; } - (void)didReceiveMemoryWarning { [super didReceiveMemoryWarning]; // Dispose of any resources that can be recreated. } @end
Output
When we run the application, we'll get the output as shown below −
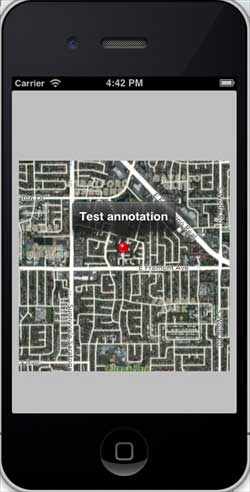
When we scroll the map up, we will get the output as shown below −
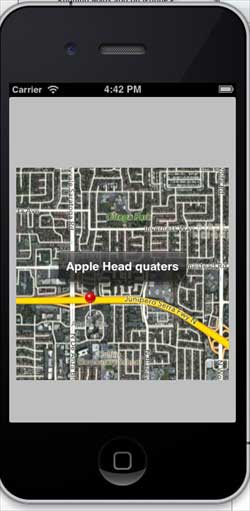