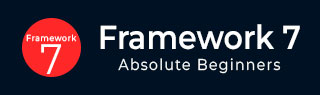
- Framework7 Tutorial
- Framework7 - Home
- Framework7 - Overview
- Framework7 - Environment
- Framework7 Components
- Framework7 - Layouts
- Framework7 - Navbars
- Framework7 - Toolbars
- Framework7 - Search Bar
- Framework7 - Status Bar
- Framework7 - Side Panels
- Framework7 - Content Block
- Framework7 - Layout Grid
- Framework7 - Overlays
- Framework7 - Preloaders
- Framework7 - Progress Bar
- Framework7 - List Views
- Framework7 - Accordion
- Framework7 - Cards
- Framework7 - Chips
- Framework7 - Buttons
- Framework7 - Action Button
- Framework7 - Forms
- Framework7 - Tabs
- Framework7 - Swiper Slider
- Framework7 - Photo Browser
- Framework7 - Autocomplete
- Framework7 - Picker
- Framework7 - Calendar
- Framework7 - Refresh
- Framework7 - Infinite Scroll
- Framework7 - Messages
- Framework7 - Message Bar
- Framework7 - Notifications
- Framework7 - Lazy Load
- Framework7 Styling
- Framework7 - Color Themes
- Framework7 - Hairlines
- Framework7 Templates
- Framework7 - Templates Overview
- Framework7 - Auto Compilation
- Framework7 - Template7 Pages
- Framework7 Fast Clicks
- Framework7 - Active State
- Framework7 - Tap Hold Event
- Framework7 - Touch Ripple
- Framework7 Useful Resources
- Framework7 - Quick Guide
- Framework7 - Useful Resources
- Framework7 - Discussion
Framework7 - Open & Close Popover Using JavaScript
Description
The popover can also be opened and closed with JavaScript by using the related app methods as shown below −
myApp.popover(popover, target) − It is used to open the popover around the target element and it accepts the following arguments −
popover − It is a required argument, which is an HTMLElement or string (with CSS Selector) of popover to open.
target − It is a required argument, which is an HTMLElement or string (with CSS Selector) of target element used to set popover position around this element.
myApp.closeModal(popover) − It is used to close the popover and accepts popover argument, which is an HTMLElement or string (with CSS Selector). It is an optional argument and if not specified, any of the opened popover will be closed.
If you open popover using JavaScript, the target element needs to be passed to set the popover around the target element.
Example
The following example demonstrates the use of opening and closing popover using JavaScript in Framework7 −
<!DOCTYPE html> <html> <head> <meta name = "viewport" content = "width = device-width, initial-scale = 1, maximum-scale = 1, minimum-scale = 1, user-scalable = no, minimal-ui" /> <meta name = "apple-mobile-web-app-capable" content = "yes" /> <meta name = "apple-mobile-web-app-status-bar-style" content = "black" /> <title>Open and close Popover Using JavaScript</title> <link rel = "stylesheet" href = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/css/framework7.ios.min.css" /> <link rel = "stylesheet" href = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/css/framework7.ios.colors.min.css" /> </head> <body> <div class = "views"> <div class = "view view-main"> <div class = "pages"> <div data-page = "home" class = "page navbar-fixed"> <div class = "navbar"> <div class = "navbar-inner"> <div class = "left"> <a href = "#" class = "link open-menus">Menus</a></div> <div class = "center">Open and close Popover Using JavaScript</div> <div class = "right"> <a href = "#" class = "link open-about">About</a></div> </div> </div> <div class = "page-content"> <div class = "content-block"> <p><a href = "#" class = "open-menus">Open menus Popover</a></p> <p><a href = "#" class = "open-about">Open About Popover</a></p> <p>Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat <a href = "#" class = "open-about">About</a> nibh iaculis quis. Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum <a href = "#" class = "open-menus">Menus</a>.</p> </div> </div> </div> </div> </div> </div> <style>.popover{width:200px;}</style> <div class = "popover popover-about"> <div class = "popover-angle"></div> <div class = "popover-inner"> <div class = "content-block"> <p>About</p> <p>Lorem ipsum dolor sit amet, consectetur adipiscing elit. Quisque ac diam ac quam euismod porta vel a nunc.</p> </div> </div> </div> <div class = "popover popover-menus"> <div class = "popover-angle"></div> <div class = "popover-inner"> <div class = "list-block"> <ul> <li><a href = "#" class = "list-button item-link">Menu 1</a></li> <li><a href = "#" class = "list-button item-link">Menu 2</a></li> <li><a href = "#" class = "list-button item-link">Menu 3</a></li> <li><a href = "#" class = "list-button item-link">Menu 4</a></li> <li><a href = "#" class = "list-button item-link">Menu 5</a></li> </ul> </div> </div> </div> <script type = "text/javascript" src = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/js/framework7.min.js"></script> <script> var myApp = new Framework7(); var $$ = Dom7; $$('.open-about').on('click', function () { var clickedLink = this; myApp.popover('.popover-about', clickedLink); }); $$('.open-menus').on('click', function () { var clickedLink = this; myApp.popover('.popover-menus', clickedLink); }); </script> </body> </html>
Output
Let us carry out the following steps to see how the above given code works −
Save the above given HTML code as popover_open_close_js.html file in your server root folder.
Open this HTML file as http://localhost/popover_open_close_js.html and the output is displayed as shown below.
When you click on the first link, the menus popover window opens with several menu items. Similarly, when you click on the second link, the about popover window opens.
The menu and the about popover windows also open by clicking on the menu and about options. The popover will open and close around the target element using javascript.