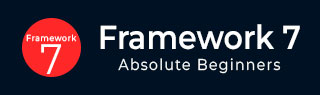
- Framework7 Tutorial
- Framework7 - Home
- Framework7 - Overview
- Framework7 - Environment
- Framework7 Components
- Framework7 - Layouts
- Framework7 - Navbars
- Framework7 - Toolbars
- Framework7 - Search Bar
- Framework7 - Status Bar
- Framework7 - Side Panels
- Framework7 - Content Block
- Framework7 - Layout Grid
- Framework7 - Overlays
- Framework7 - Preloaders
- Framework7 - Progress Bar
- Framework7 - List Views
- Framework7 - Accordion
- Framework7 - Cards
- Framework7 - Chips
- Framework7 - Buttons
- Framework7 - Action Button
- Framework7 - Forms
- Framework7 - Tabs
- Framework7 - Swiper Slider
- Framework7 - Photo Browser
- Framework7 - Autocomplete
- Framework7 - Picker
- Framework7 - Calendar
- Framework7 - Refresh
- Framework7 - Infinite Scroll
- Framework7 - Messages
- Framework7 - Message Bar
- Framework7 - Notifications
- Framework7 - Lazy Load
- Framework7 Styling
- Framework7 - Color Themes
- Framework7 - Hairlines
- Framework7 Templates
- Framework7 - Templates Overview
- Framework7 - Auto Compilation
- Framework7 - Template7 Pages
- Framework7 Fast Clicks
- Framework7 - Active State
- Framework7 - Tap Hold Event
- Framework7 - Touch Ripple
- Framework7 Useful Resources
- Framework7 - Quick Guide
- Framework7 - Useful Resources
- Framework7 - Discussion
Framework7 - Messages
Description
Messages are component of Framework7, which gives visualization of comments and messaging system in the application.
Messages Layout
The framework7 has the following messages layout structure −
<div class = "page"> <div class = "page-content messages-content"> <div class = "messages"> <!-- Defines the date stamp --> <div class = "messages-date">Monday, Apr 26 <span>10:30</span></div> <!-- Displays the sent message and by default, it will be in green color on right side --> <div class = "message message-sent"> <!-- Define the text with bubble type --> <div class = "message-text">Hello</div> </div> <!-- Displays the another sent message --> <div class = "message message-sent"> <!-- Define the text with bubble type --> <div class = "message-text">How are you?</div> </div> <!-- Displays the received message and by default, it will be in grey color on left side --> <div class = "message message-with-avatar message-received"> <!-- Provides sender name --> <div class = "message-name">Smith</div> <!-- Define the text with bubble type --> <div class = "message-text">I am fine, thanks</div> <!-- Defines the another date stamp --> <div class = "messages-date">Tuesday, April 28 <span>11:16</span></div> </div> </div> </div>
The layout contains the following classes in different areas −
Messages Page Layout
The following table shows the classes of messages page layout with description.
S.No | Classes & Description |
---|---|
1 | messages-content It is a required additional class added to "page-content" and used for messages wrapper. |
2 | messages It is a required element for messages bubbles. |
3 | messages-date It uses date stamp container to display date and time of message sent or received. |
4 | message It is a single message to be displayed. |
Single Message Inner Parts
The following table shows the classes of simple message inner parts with description.
S.No | Classes & Description |
---|---|
1 | message-name It provides the sender name. |
2 | message-text Define the text with bubble type. |
3 | message-avatar It specifies the sender avatar. |
4 | message-label It specifies the text label below the bubble. |
Additional classes for Single Message Container
The following table shows additional classes for single message container description.
S.No | Classes & Description |
---|---|
1 | message-sent It specifies that message was sent by the user and is displayed with green background color on the right side. |
2 | message-received It is used for displaying the single message indicating that, the message was received by user and stays on the left side with grey background color. |
3 | message-pic It is an additional class for displaying image with a single message. |
4 | message-with-avatar It is an additional class for displaying a single message (received or sent) with avatar. |
5 | message-with-tail You can add a bubble tail for single message (received or sent). |
Additional Available Classes for Single Message
The following table shows the available classes for a single message with description.
S.No | Classes & Description |
---|---|
1 | message-hide-name It is an additional class for hiding message name for a single message (received or sent). |
2 | message-hide-avatar It is an additional class for hiding message avatar for a single message (received or sent). |
3 | message-hide-label It is an additional class for hiding message label for a single message (received or sent). |
4 | message-last You can use this class to indicate the last received or sent message in current conversation by one sender for a single message (received or sent). |
5 | message-first You can use this class to indicate first received or first sent message in current conversation by one sender for a single message (received or sent). |
Initializing Messages with JavaScript
You can initialize the messages with JavaScript by using the following method −
myApp.messages(messagesContainer, parameters)
The method takes two options −
messagesContainer − It is a required HTML element or string that includes messages container HTML element.
parameters − It specifies an object with messages parameters.
Messages Parameters
The following table shows the parameters of messages with description.
S.No | Parameter & Description | Type | Default |
---|---|---|---|
1 | autoLayout It adds additional required classes to each message by enabling it. |
boolean | true |
2 | newMessagesFirst You can display message on top instead of displaying on bottom by enabling it. |
boolean | false |
3 | messages It displays an array of messages in which each message should be represented as object with message parameters. |
array | - |
4 | messageTemplate It displays the single message template. |
string | - |
Messages Properties
The following table shows the properties of messages with description.
S.No | Property & Description |
---|---|
1 | myMessages.params You can initialize the passed parameters with object. |
2 | myMessages.container Defines the DOM7 element with a message bar HTML container. |
Messages Methods
The following table shows the methods of messages with description.
S.No | Method & Description |
---|---|
1 | myMessages.addMessage(messageParameters, method, animate); The message can be added to the beginning or to the end by using the method parameter. It has the following parameters −
|
2 | myMessages.appendMessage(messageParameters, animate); It adds a message to the end of message container. |
3 | myMessages.prependMessage(messageParameters, animate); It adds a message to the beginning of message container. |
4 | myMessages.addMessages(messages, method, animate); You can add multiple messages at one time. It has the following parameter −
|
5 | myMessages.removeMessage(message); It is used to remove the message. It has the following parameter −
|
6 | myMessages.removeMessages(messages); You can remove the multiple messages. It has the following parameter −
|
7 | myMessages.scrollMessages(); You can scroll messages from top to bottom and vice versa depending on the first parameter of new message. |
8 | myMessages.layout(); Auto layout can be applied to the messages. |
9 | myMessages.clean(); It is used to clean the messages. |
10 | myMessages.destroy(); It is used to destroy the messages. |
Single Message Parameters
The following table shows the parameters for a single message with description.
S.No | Parameter & Description | Type | Default |
---|---|---|---|
1 | text It defines the message text, which could be a HTML string. |
string | - |
2 | name It specifies the sender name. |
string | - |
3 | avatar It specifies the sender avatar URL string. |
string | - |
4 | time It specifies the time string of the message like '9:45 AM', '18:35'. |
string | - |
5 | type It provides type of message whether it could be sent or recieved message. |
string | sent |
6 | label It defines the label of the message. |
string | - |
7 | day It gives the day string of the message like 'sunday', 'monday', 'yesterday' etc. |
string | - |
Initializing Messages with HTML
You can initialize the messages with HTML without JavaScript by using the additional messages-init class to messages and use the data- attributes to pass the required parameters as shown in the code snippet given below −
... <div class = "page-content messages-content"> <!-- Initialize the messages using additional "messages-init" class--> <div class = "messages messages-init" data-auto-layout = "true" data-new-messages-first = "false"> ... </div> </div> ...
Example
The following example demonstrates the use of messages in the Framework7 −
<!DOCTYPE html> <html> <head> <meta name = "viewport" content = "width = device-width, initial-scale = 1, maximum-scale = 1, minimum-scale = 1, user-scalable = no, minimal-ui" /> <meta name = "apple-mobile-web-app-capable" content = "yes" /> <meta name = "apple-mobile-web-app-status-bar-style" content = "black" /> <title>Messages</title> <link rel = "stylesheet" href = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/css/framework7.ios.min.css" /> <link rel = "stylesheet" href = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/css/framework7.ios.colors.min.css" /> </head> <body> <div class = "views"> <div class = "view view-main"> <div class = "pages"> <div data-page = "home" class = "page navbar-fixed toolbar-fixed"> <div class = "navbar"> <div class = "navbar-inner"> <div class = "left"> </div> <div class = "center">Messages</div> <div class = "right"> </div> </div> </div> <div class = "toolbar messagebar"> <div class = "toolbar-inner"> <textarea placeholder = "Message"></textarea><a href = "#" class = "link">Send</a> </div> </div> <div class = "page-content messages-content"> <div class = "messages"> <div class = "messages-date">Friday, Apr 26 <span>10:30</span></div> <div class = "message message-sent"> <div class = "message-text">Hello</div> </div> <div class = "message message-sent"> <div class = "message-text">Happy Birthday</div> </div> <div class = "message message-received"> <div class = "message-name">Smith</div> <div class = "message-text">Thank you</div> <div style = "background-image:url(/framework7/images/person.png)" class = "message-avatar"></div> </div> <div class = "messages-date">Saturday, Apr 27 <span>9:30</span></div> <div class = "message message-sent"> <div class = "message-text">Good Morning...</div> </div> <div class = "message message-sent"> <div class = "message-text"><img src = "/framework7/images/gm.jpg"></div> <div class = "message-label">Delivered</div> </div> <div class = "message message-received"> <div class = "message-name">Smith</div> <div class = "message-text">Very Good Morning...</div> <div style = "background-image:url(/framework7/images/person.png)" class = "message-avatar"></div> </div> </div> </div> </div> </div> </div> </div> <script type = "text/javascript" src = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/js/framework7.min.js"></script> <script> var myApp = new Framework7(); var $$ = Dom7; // It indicates conversation flag var conversationStarted = false; // Here initiliaze the messages var myMessages = myApp.messages('.messages', { autoLayout:true }); // Initiliaze the messagebar var myMessagebar = myApp.messagebar('.messagebar'); // Displays the text after clicking the button $$('.messagebar .link').on('click', function () { // specifies the message text var messageText = myMessagebar.value().trim(); // If there is no message, then exit from there if (messageText.length === 0) return; // Specifies the empty messagebar myMessagebar.clear() // Defines the random message type var messageType = (['sent', 'received'])[Math.round(Math.random())]; // Provides the avatar and name for the received message var avatar, name; if(messageType === 'received') { name = 'Smith'; } // It adds the message myMessages.addMessage ({ // It provides the message text text: messageText, // It displays the random message type type: messageType, // Specifies the avatar and name of the sender avatar: avatar, name: name, // Displays the day, date and time of the message day: !conversationStarted ? 'Today' : false, time: !conversationStarted ? (new Date()).getHours() + ':' + (new Date()).getMinutes() : false }) // Here you can update the conversation flag conversationStarted = true; }); </script> </body> </html>
Output
Let us carry out the following steps to see how the above given code works −
Save the above given HTML code as messages.html file in your server root folder.
Open this HTML file as http://localhost/messages.html and the output is displayed as shown below.
When you type a message into the message box and click the Send button, it specifies that your message has been sent and is displayed with green background color on the right side.
The message which you recieve, appears on the left side with the grey background along with the sender name.