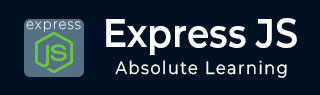
- ExpressJS Tutorial
- ExpressJS - Home
- ExpressJS - Overview
- ExpressJS - Environment
- ExpressJS - Hello World
- ExpressJS - Routing
- ExpressJS - HTTP Methods
- ExpressJS - URL Building
- ExpressJS - Middleware
- ExpressJS - Templating
- ExpressJS - Static Files
- ExpressJS - Form Data
- ExpressJS - Database
- ExpressJS - Cookies
- ExpressJS - Sessions
- ExpressJS - Authentication
- ExpressJS - RESTful APIs
- ExpressJS - Scaffolding
- ExpressJS - Error handling
- ExpressJS - Debugging
- ExpressJS - Best Practices
- ExpressJS - Resources
- ExpressJS Useful Resources
- ExpressJS - Quick Guide
- ExpressJS - Useful Resources
- ExpressJS - Discussion
ExpressJS - Database
We keep receiving requests, but end up not storing them anywhere. We need a Database to store the data. For this, we will make use of the NoSQL database called MongoDB.
To install and read about Mongo, follow this link.
In order to use Mongo with Express, we need a client API for node. There are multiple options for us, but for this tutorial, we will stick to mongoose. Mongoose is used for document Modeling in Node for MongoDB. For document modeling, we create a Model (much like a class in document oriented programming), and then we produce documents using this Model (like we create documents of a class in OOP). All our processing will be done on these "documents", then finally, we will write these documents in our database.
Setting up Mongoose
Now that you have installed Mongo, let us install Mongoose, the same way we have been installing our other node packages −
npm install --save mongoose
Before we start using mongoose, we have to create a database using the Mongo shell. To create a new database, open your terminal and enter "mongo". A Mongo shell will start, enter the following code −
use my_db
A new database will be created for you. Whenever you open up the mongo shell, it will default to "test" db and you will have to change to your database using the same command as above.
To use Mongoose, we will require it in our index.js file and then connect to the mongodb service running on mongodb://localhost.
var mongoose = require('mongoose'); mongoose.connect('mongodb://localhost/my_db');
Now our app is connected to our database, let us create a new Model. This model will act as a collection in our database. To create a new Model, use the following code, before defining any route −
var personSchema = mongoose.Schema({ name: String, age: Number, nationality: String }); var Person = mongoose.model("Person", personSchema);
The above code defines the schema for a person and is used to create a Mongoose Mode Person.
Saving Documents
Now, we will create a new html form; this form will help you get the details of a person and save it to our database. To create the form, create a new view file called person.pug in views directory with the following content −
html head title Person body form(action = "/person", method = "POST") div label(for = "name") Name: input(name = "name") br div label(for = "age") Age: input(name = "age") br div label(for = "nationality") Nationality: input(name = "nationality") br button(type = "submit") Create new person
Also add a new get route in index.js to render this document −
app.get('/person', function(req, res){ res.render('person'); });
Go to "localhost:3000/person" to check if the form is displaying the correct output. Note that this is just the UI, it is not working yet. The following screenshot shows how the form is displayed −
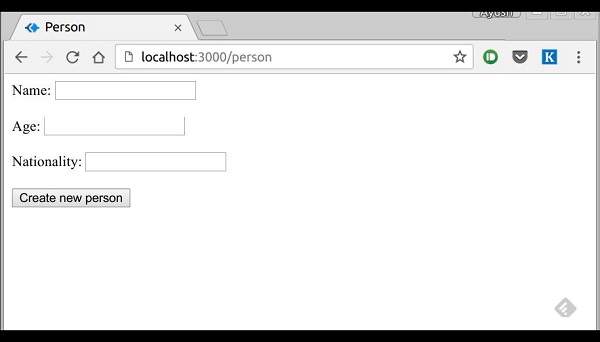
We will now define a post route handler at '/person' which will handle this request
app.post('/person', function(req, res){ var personInfo = req.body; //Get the parsed information if(!personInfo.name || !personInfo.age || !personInfo.nationality){ res.render('show_message', { message: "Sorry, you provided worng info", type: "error"}); } else { var newPerson = new Person({ name: personInfo.name, age: personInfo.age, nationality: personInfo.nationality }); newPerson.save(function(err, Person){ if(err) res.render('show_message', {message: "Database error", type: "error"}); else res.render('show_message', { message: "New person added", type: "success", person: personInfo}); }); } });
In the above code, if we receive any empty field or do not receive any field, we will send an error response. But if we receive a well-formed document, then we create a newPerson document from Person model and save it to our DB using the newPerson.save() function. This is defined in Mongoose and accepts a callback as argument. This callback has 2 arguments – error and response. These arguments will render the show_message view.
To show the response from this route, we will also need to create a show_message view. Create a new view with the following code −
html head title Person body if(type == "error") h3(style = "color:red") #{message} else h3 New person, name: #{person.name}, age: #{person.age} and nationality: #{person.nationality} added!
We will receive the following response on successfully submitting the form(show_message.pug) −

We now have an interface to create persons.
Retrieving Documents
Mongoose provides a lot of functions for retrieving documents, we will focus on 3 of those. All these functions also take a callback as the last parameter, and just like the save function, their arguments are error and response. The three functions are as follows −
Model.find(conditions, callback)
This function finds all the documents matching the fields in conditions object. Same operators used in Mongo also work in mongoose. For example,
Person.find(function(err, response){ console.log(response); });
This will fetch all the documents from the person's collection.
Person.find({name: "Ayush", age: 20}, function(err, response){ console.log(response); });
This will fetch all documents where field name is "Ayush" and age is 20.
We can also provide projection we need, i.e., the fields we need. For example, if we want only the names of people whose nationality is "Indian", we use −
Person.find({nationality: "Indian"}, "name", function(err, response){ console.log(response); });
Model.findOne(conditions, callback)
This function always fetches a single, most relevant document. It has the same exact arguments as Model.find().
Model.findById(id, callback)
This function takes in the _id(defined by mongo) as the first argument, an optional projection string and a callback to handle the response. For example,
Person.findById("507f1f77bcf86cd799439011", function(err, response){ console.log(response); });
Let us now create a route to view all people records −
var express = require('express'); var app = express(); var mongoose = require('mongoose'); mongoose.connect('mongodb://localhost/my_db'); var personSchema = mongoose.Schema({ name: String, age: Number, nationality: String }); var Person = mongoose.model("Person", personSchema); app.get('/people', function(req, res){ Person.find(function(err, response){ res.json(response); }); }); app.listen(3000);
Updating Documents
Mongoose provides 3 functions to update documents. The functions are described below −
Model.update(condition, updates, callback)
This function takes a conditions and updates an object as input and applies the changes to all the documents matching the conditions in the collection. For example, following code will update the nationality "American" in all Person documents −
Person.update({age: 25}, {nationality: "American"}, function(err, response){ console.log(response); });
Model.findOneAndUpdate(condition, updates, callback)
It finds one document based on the query and updates that according to the second argument. It also takes a callback as last argument. Let us perform the following example to understand the function
Person.findOneAndUpdate({name: "Ayush"}, {age: 40}, function(err, response) { console.log(response); });
Model.findByIdAndUpdate(id, updates, callback)
This function updates a single document identified by its id. For example,
Person.findByIdAndUpdate("507f1f77bcf86cd799439011", {name: "James"}, function(err, response){ console.log(response); });
Let us now create a route to update people. This will be a PUT route with the id as a parameter and details in the payload.
var express = require('express'); var app = express(); var mongoose = require('mongoose'); mongoose.connect('mongodb://localhost/my_db'); var personSchema = mongoose.Schema({ name: String, age: Number, nationality: String }); var Person = mongoose.model("Person", personSchema); app.put('/people/:id', function(req, res){ Person.findByIdAndUpdate(req.params.id, req.body, function(err, response){ if(err) res.json({message: "Error in updating person with id " + req.params.id}); res.json(response); }); }); app.listen(3000);
To test this route, enter the following in your terminal (replace the id with an id from your created people) −
curl -X PUT --data "name = James&age = 20&nationality = American "http://localhost:3000/people/507f1f77bcf86cd799439011
This will update the document associated with the id provided in the route with the above details.
Deleting Documents
We have covered Create, Read and Update, now we will see how Mongoose can be used to Delete documents. We have 3 functions here, exactly like update.
Model.remove(condition, [callback])
This function takes a condition object as input and removes all documents matching the conditions. For example, if we need to remove all people aged 20, use the following syntax −
Person.remove({age:20});
Model.findOneAndRemove(condition, [callback])
This functions removes a single, most relevant document according to conditions object. Let us execute the following code to understand the same.
Person.findOneAndRemove({name: "Ayush"});
Model.findByIdAndRemove(id, [callback])
This function removes a single document identified by its id. For example,
Person.findByIdAndRemove("507f1f77bcf86cd799439011");
Let us now create a route to delete people from our database.
var express = require('express'); var app = express(); var mongoose = require('mongoose'); mongoose.connect('mongodb://localhost/my_db'); var personSchema = mongoose.Schema({ name: String, age: Number, nationality: String }); var Person = mongoose.model("Person", personSchema); app.delete('/people/:id', function(req, res){ Person.findByIdAndRemove(req.params.id, function(err, response){ if(err) res.json({message: "Error in deleting record id " + req.params.id}); else res.json({message: "Person with id " + req.params.id + " removed."}); }); }); app.listen(3000);
To check the output, use the following curl command −
curl -X DELETE http://localhost:3000/people/507f1f77bcf86cd799439011
This will remove the person with given id producing the following message −
{message: "Person with id 507f1f77bcf86cd799439011 removed."}
This wraps up how we can create simple CRUD applications using MongoDB, Mongoose and Express. To explore Mongoose further, read the API docs.