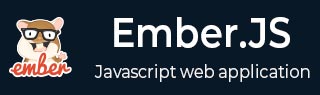
- EmberJS Tutorial
- EmberJS - Home
- EmberJS - Overview
- EmberJS - Installation
- EmberJS - Core Concepts
- Creating and Running Application
- EmberJS - Object Model
- EmberJS - Router
- EmberJS - Templates
- EmberJS - Components
- EmberJS - Models
- EmberJS - Managing Dependencies
- EmberJS - Application Concerns
- EmberJS - Configuring Ember.js
- EmberJS - Ember Inspector
- EmberJS Useful Resources
- EmberJS - Quick Guide
- EmberJS - Useful Resources
- EmberJS - Discussion
EmberJS- Built-In Views
Built-In Views
Ember.js supports for built-in basic controls like text inputs, check boxes and select lists which are used via input helpers. Some set of built-in views are −
Ember.Checkbox
Ember.TextField
Ember.TextArea
Ember.Select
Example
<!DOCTYPE html> <html> <head> <title>Emberjs Built-In Views</title> <!-- CDN's--> <script src="https://cdnjs.cloudflare.com/ajax/libs/handlebars.js/3.0.1/handlebars.min.js"></script> <script src="https://code.jquery.com/jquery-2.1.3.min.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/ember.js/1.10.0/ember.min.js"></script> <script src="https://builds.emberjs.com/tags/v1.10.0-beta.3/ember-template-compiler.js"></script> <script src="https://builds.emberjs.com/release/ember.debug.js"></script> <script src="https://builds.emberjs.com/beta/ember-data.js"></script> </head> <body> <script type="text/x-handlebars"> <!-- defining the built-in Ember.select view --> {{view Ember.Select <!-- creating a property called content with the value --> contentBinding="App.peopleController" selectionBinding="App.selectedPersonController.person" optionLabelPath="content.fullName" optionValuePath="content.id"}} <p>Selected: {{App.selectedPersonController.person.fullName}} (ID: {{App.selectedPersonController.person.id}})</p> </script> <script type="text/javascript"> App = Ember.Application.create(); App.Person = Ember.Object.extend({ id: null, firstName: null, lastName: null, //defining computed property as fullName fullName: function() { return this.get('firstName') + " " + this.get('lastName'); }.property('firstName', 'lastName') }); //at the first time selected person value is null App.selectedPersonController = Ember.Object.create({ person: null }); //creating the array controller which holds the details of person with id App.peopleController = Ember.ArrayController.create({ content: [ App.Person.create({id: 1, firstName: 'Mack', lastName: 'SK'}), App.Person.create({id: 2, firstName: 'Smith', lastName: 'DK'}) ] }); </script> </body> </html>
Output
Let's carry out the following steps to see how above code works −
Save above code in built_in_view.htm file
Open this HTML file in a browser.
Advertisements
To Continue Learning Please Login