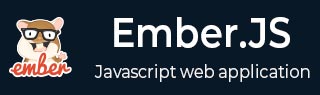
- EmberJS Tutorial
- EmberJS - Home
- EmberJS - Overview
- EmberJS - Installation
- EmberJS - Core Concepts
- Creating and Running Application
- EmberJS - Object Model
- EmberJS - Router
- EmberJS - Templates
- EmberJS - Components
- EmberJS - Models
- EmberJS - Managing Dependencies
- EmberJS - Application Concerns
- EmberJS - Configuring Ember.js
- EmberJS - Ember Inspector
- EmberJS Useful Resources
- EmberJS - Quick Guide
- EmberJS - Useful Resources
- EmberJS - Discussion
EmberJS-Testing User Interaction
Testing User Interaction
User can interact with the page by using the helpers and check for the changes in the DOM. When an unauthorized user try to access a particular URL, then you can make him to be transitioned to a login page.
Example
<!DOCTYPE html> <html> <head> <title>Emberjs Testing Interaction</title> <!-- CDN's--> <script src="https://cdnjs.cloudflare.com/ajax/libs/handlebars.js/3.0.1/handlebars.min.js"></script> <script src="https://code.jquery.com/jquery-2.1.3.min.js"></script> <script src="https://builds.emberjs.com/tags/v1.10.0-beta.3/ember-template-compiler.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/ember.js/1.10.0/ember.prod.js"></script> <!-- CDN's for testing Ember.js programs --< <script src="https://code.jquery.com/qunit/qunit-1.18.0.js"></script> <script src="https://rawgit.com/rwjblue/ember-qunit-builds/master/ember-qunit.js"></script> <script src="https://builds.emberjs.com/release/ember.debug.js"></script> <script src="https://builds.emberjs.com/beta/ember-data.js"></script> </head> <body> <div id="qunit"></div> <div id="ember-testing"></div> <!-- These are used to link different url's --> <script type="text/x-handlebars"> <ul> <li>{{#link-to "index"}}Login 1{{/link-to}}</li> <li>{{#link-to "Login2"}}Login 2{{/link-to}}</li> </ul> {{/outlet}} </script> <script type="text/x-handlebars" data-template-name="index"> <p>This is login 1</p> </script> <!-- Display this line if not authenticated --> <script type="text/x-handlebars" data-template-name="login"> <p>This is my Login</p> </script> <script type="text/x-handlebars" data-template-name="Login2"> <p>This is login 2</p> </script> <script type="text/javascript"> //Creates an instance of Ember.Application and assign it to a global variable App = Ember.Application.create(); //The map method of application's router can be invoked to define URL mappings App.Router.map(function() { this.route('login'); this.route('Login2'); }); // 'Login2Route' should render by defining a route with the same name as the template App.Login2Route = Ember.Route.extend({ beforeModel: function() { var myval = this.modelFor('application'); //If we pass an empty object, then it create a link to a route using 'transitionTo' method if (Ember.isEmpty(myval)) { this.transitionTo('login'); } } }); //These methods are used to prepare the application for testing App.setupForTesting(); App.injectTestHelpers(); App.rootElement = '#ember-testing'; //Ember.js applications's root element module('Emberjs', { setup: function() { App.reset(); //After each test, reset the state of the application } }); //Here, it tests the workflow of an application test('redirect to login if not authenticated', function() { visit('/'); click('.Login2'); //'andThen' helper wait for the preceding asynchronous test helpers to complete andThen(function() { equal(currentRouteName(), 'login'); }); }); </script> </body> </html>
Output
Let's carry out the following steps to see how above code works −
Save above code in testing_userinteraction.htm file
Open this HTML file in a browser.
Advertisements
To Continue Learning Please Login