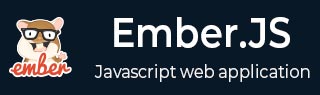
- EmberJS Tutorial
- EmberJS - Home
- EmberJS - Overview
- EmberJS - Installation
- EmberJS - Core Concepts
- Creating and Running Application
- EmberJS - Object Model
- EmberJS - Router
- EmberJS - Templates
- EmberJS - Components
- EmberJS - Models
- EmberJS - Managing Dependencies
- EmberJS - Application Concerns
- EmberJS - Configuring Ember.js
- EmberJS - Ember Inspector
- EmberJS Useful Resources
- EmberJS - Quick Guide
- EmberJS - Useful Resources
- EmberJS - Discussion
EmberJS-Testing Routes
Testing Routes
You can test the routes by using two ways. One is acceptance and another one is unit tests. Acceptance tests are generally used to test workflow of your application and unit tests are generally used to test a small piece of code.
Example
<!DOCTYPE html> <html> <head> <title>EmberJs Tesing Routes</title> <!-- CSS for testing programs --> <link href="https://code.jquery.com/qunit/qunit-git.css" rel="stylesheet" type="text/css" /> <script src="https://cdnjs.cloudflare.com/ajax/libs/handlebars.js/3.0.1/handlebars.min.js"></script> <script src="https://code.jquery.com/jquery-2.1.3.min.js"></script> <script src="https://builds.emberjs.com/tags/v1.10.0-beta.3/ember-template-compiler.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/ember.js/1.10.0/ember.prod.js"></script> <script src="https://code.jquery.com/qunit/qunit-1.18.0.js"></script> <script src="https://rawgit.com/rwjblue/ember-qunit-builds/master/ember-qunit.js"></script> <script src="https://builds.emberjs.com/release/ember.debug.js"></script> <script src="https://builds.emberjs.com/beta/ember-data.js"></script> </head> <body> <div id="qunit"> </div> <div id="ember-testing"></div> <script type="text/x-handlebars"> {{#link-to 'index'}}Index{{/link-to}} {{#link-to 'home'}}Home{{/link-to}} {{outlet}} </script> <script type="text/x-handlebars" data-template-name="index"> <h2>Index</h2> <button {{action "displayAlert" navtext}}>Index </button> </script> <script type="text/x-handlebars" data-template-name="home"> <h2>Home</h2> <button {{action "displayAlert" navtext}}>Home </button> </script> <script type="text/javascript"> //Creates an instance of Ember.Application and assign it to a global variable App = Ember.Application.create(); //The 'map' method can be invoked to define URL mappings App.Router.map(function() { this.route('home'); }); //Customize the behavior of a route by creating an Ember.Route subclass App.ApplicationRoute = Ember.Route.extend({ _displayText: function(navtext) { document.write(navtext); }, actions: { displayText: function(navtext) { this._displayText(navtext); } } }); //The IndexController template stores its properties and sends its actions to the Controller App.IndexController = Ember.Controller.extend({ navtext: 'Index' }); //The HomeController template stores its properties and sends its actions to the Controller App.HomeController = Ember.Controller.extend({ navtext: 'Home' }); //emq.globalize(); //The 'DefaultResolver' defines the default lookup rules before consulting the container for registered items setResolver(Ember.DefaultResolver.create({ namespace: App })); App.setupForTesting(); //This method is used to prepare the application for testing var res; //The 'moduleFor' helper is used to setup a test container moduleFor('route:application', 'Unit: route/application', { setup: function() { res = window.document.write; //Store a reference to the browser }, teardown: function() { window.document.write = res; //Restore original functions } }); test('Display is called on displayText', function() { //'this.subject()' is used to get an instance of the 'IndexController' and 'HomeController' var route = this.subject(); //The text to be display var expectedText = 'Tutorialspoint'; //Defines window.document.write to perform a qunit test window.document.write = function(navtext) { equal(navtext, expectedText, 'expected ' + navtext + ' to ' + expectedText); } //Call the _displayText function which triggers the qunit test above route._displayText(expectedText); }); </script> </body> </html>
Output
Let's carry out the following steps to see how above code works −
Save above code in testing_routes.htm file
Open this HTML file in a browser.
Advertisements
To Continue Learning Please Login