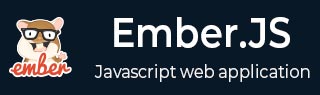
- EmberJS Tutorial
- EmberJS - Home
- EmberJS - Overview
- EmberJS - Installation
- EmberJS - Core Concepts
- Creating and Running Application
- EmberJS - Object Model
- EmberJS - Router
- EmberJS - Templates
- EmberJS - Components
- EmberJS - Models
- EmberJS - Managing Dependencies
- EmberJS - Application Concerns
- EmberJS - Configuring Ember.js
- EmberJS - Ember Inspector
- EmberJS Useful Resources
- EmberJS - Quick Guide
- EmberJS - Useful Resources
- EmberJS - Discussion
EmberJS-Acceptance Tests
Acceptance Tests
To test user interaction with application and flow of application, the acceptance tests are used. By using acceptance tests user can login via login form and can able to create blog post.
Example
<!DOCTYPE html> <html> <head> <title>Emberjs Acceptance Test</title> <!-- CDN's--> <script src="https://cdnjs.cloudflare.com/ajax/libs/handlebars.js/3.0.1/handlebars.min.js"></script> lt;script src="https://code.jquery.com/jquery-2.1.3.min.js"></script> <script src="https://builds.emberjs.com/tags/v1.10.0-beta.3/ember-template-compiler.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/ember.js/1.10.0/ember.prod.js"></script> <script src="https://builds.emberjs.com/release/ember.debug.js"></script> <script src="https://builds.emberjs.com/beta/ember-data.js"></script> </head> <body> <div id="qunit"></div> <div id="ember-testing-container"><div id="ember-testing"></div></div> <script type="text/x-handlebars" data-template-name="index"> <h2>Names are:</h2> <ul> {{#each}} <li>{{#link-to 'post' this}} {{firstName}} {{lastName}} {{/link-to}}</li> {{/each}} </ul> </script> <script type="text/x-handlebars" data-template-name="post"> <h2>Name :</h2> <p id='name'> {{firstName}} </p> </script> <script type="text/javascript"> //Creates an instance of Ember.Application and assign it to a global variable App = Ember.Application.create(); //The store cache of all records available in an application App.Store = DS.Store.extend({ //adapter translating requested records into the appropriate calls adapter: DS.FixtureAdapter }); App.Router.map(function() { //post route this.route('post', { path: ':person_id' }); }); App.IndexRoute = Ember.Route.extend({ model: function() { return this.store.find('person'); } }); App.Person = DS.Model.extend({ //setting firstName and lastName attributes as string firstName: DS.attr('string'), lastName: DS.attr('string') }); //attach fixtures(sample data) to the model's class App.Person.FIXTURES = [ {id: 1, firstName: 'John', lastName: 'Smith'}, {id: 2, firstName: 'Tom', lastName: 'Cruise'}, {id: 3, firstName: 'Shane', lastName: 'Watson'} ]; App.rootElement = '#ember-testing'; //Ember.js applications's root element //These two methods to prepare the application for testing App.setupForTesting(); App.injectTestHelpers(); module("Emberjs", { setup: function() { Ember.run(App, App.advanceReadiness); //'advanceReadiness' runs the application when not under test }, //This function is called for each test in the module teardown: function() { App.reset(); //After each test, reset the state of the application } }); //Here, it tests the workflow of application test("Check HTML is returned", function() { visit("/").then(function() { ok(exists("*"), "Found HTML!"); }); }); </script> </body> </html>
Output
Let's carry out the following steps to see how above code works −
Save above code in testing_acceptance_test.htm file
Open this HTML file in a browser.
Advertisements
To Continue Learning Please Login