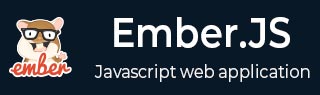
- EmberJS Tutorial
- EmberJS - Home
- EmberJS - Overview
- EmberJS - Installation
- EmberJS - Core Concepts
- Creating and Running Application
- EmberJS - Object Model
- EmberJS - Router
- EmberJS - Templates
- EmberJS - Components
- EmberJS - Models
- EmberJS - Managing Dependencies
- EmberJS - Application Concerns
- EmberJS - Configuring Ember.js
- EmberJS - Ember Inspector
- EmberJS Useful Resources
- EmberJS - Quick Guide
- EmberJS - Useful Resources
- EmberJS - Discussion
EmberJS- Setting up a Controller
Setting up a Controller
In Ember.js it is necessary to set up the controller that helps the template to display the retrieved information. Ember.js supports two built-in controllers Ember.ObjectController and Ember.ArrayController. These are presents a model's properties to a template, along with any additional display-specific properties.
Set up the model property to know which model to present, this can be done by using the route handler's setupController hook. The setupController hook gets first argument as a route handler's associated controller. You can also set the route's controllerName property other than default.
Ember.Route.extend({ setupController: function(controller, model) { controller.set('model', model); } });
In the above code, sets the model property in the route handler's setupController hook.
Example
<!DOCTYPE html> <html> <head> <title>Emberjs Setting up a Controller</title> <!-- CDN's--> <script src="https://cdnjs.cloudflare.com/ajax/libs/handlebars.js/3.0.1/handlebars.min.js"></script> <script src="https://code.jquery.com/jquery-2.1.3.min.js"></script> <script src="https://builds.emberjs.com/tags/v1.10.0-beta.3/ember-template-compiler.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/ember.js/1.10.0/ember.min.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/ember.js/1.10.0/ember.prod.js"></script> <script src="https://builds.emberjs.com/release/ember.debug.js"></script> </head> <body> <script type="text/x-handlebars" data-template-name="application"> {{outlet}} </script> <script type="text/x-handlebars" data-template-name="index"> <!-- using the default controller as index --> <b>Employee Details</b> {{#each}} <p>{{index}}. {{empDetails}}</p> {{/each}} </script> <script type="text/javascript"> App = Ember.Application.create({}); App.IndexRoute = Ember.Route.extend({ //Setting up the model property model: function(){ //declaring the array values return [ {firstName: 'Mack', lastName: 'KK'}, {firstName: 'Micky', lastName: 'SK'}, {firstName: 'Smith', lastName: 'KD'} ]; } }); App.IndexController = Ember.ArrayController.extend({ //define name of the array controller itemController:'name' }); App.NameController = Ember.ObjectController.extend({ //Defining the computed property empDetails, that holds firstName and lastName as values empDetails: function(){ //returning the computed property values return this.get('firstName')+ ' . ' + this.get('lastName'); }.property('firstName', 'lastName'), //Defining the computed property index, that holds an array index values index: function(){ //defining the array index as target to get an index values of an array return this.get('target').indexOf(this); }.property('target.[]') }); </script> </body> </html>
Output
Let's carry out the following steps to see how above code works:
Save above code in routing_cntrler.htm file
Open this HTML file in a browser.
To Continue Learning Please Login