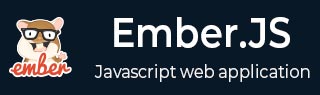
- EmberJS Tutorial
- EmberJS - Home
- EmberJS - Overview
- EmberJS - Installation
- EmberJS - Core Concepts
- Creating and Running Application
- EmberJS - Object Model
- EmberJS - Router
- EmberJS - Templates
- EmberJS - Components
- EmberJS - Models
- EmberJS - Managing Dependencies
- EmberJS - Application Concerns
- EmberJS - Configuring Ember.js
- EmberJS - Ember Inspector
- EmberJS Useful Resources
- EmberJS - Quick Guide
- EmberJS - Useful Resources
- EmberJS - Discussion
Router Update URL with replaceState Instead
You can prevent from adding an item to your browser's history by using the replaceState transition. You can specify this by using the queryParams configuration hash on the Route and opt into a replaceState transition by setting the replace transition to true.
Syntax
Ember.Route.extend ({ queryParams: { queryParameterName: { replace: true } } });
Example
The example given below shows how to update a URL with the replaceState transition. Create a new route and name it as paramreplaceState and open the router.js file to define the URL mappings −
import Ember from 'ember'; //Access to Ember.js library as variable Ember import config from './config/environment'; //It provides access to app's configuration data as variable config //The const declares read only variable const Router = Ember.Router.extend ({ location: config.locationType, rootURL: config.rootURL }); //Defines URL mappings that takes parameter as an object to create the routes Router.map(function() { this.route('paramreplaceState'); }); //It specifies Router variable available to other parts of the app export default Router;
Open the application.hbs file created under app/templates/ with the following code −
<h2>Update URL with replaceState</h2> {{#link-to 'paramreplaceState'}}Click Here{{/link-to}}
When you click the above link, the page should open with a button to change the URL after clicking on it. Open the paramreplaceState.hbs file with the following code −
//sending action to the addQuery method <button {{action 'change'}}>Replace State</button> {{outlet}}
Now open the paramreplaceState.js file created under app/controllers/ which is rendered by router when entering a Route −
import Ember from 'ember'; var words = "tutorialspoint"; export default Ember.Controller.extend ({ queryParams: ['query'], actions: { change: function() { //assigning value of variable 'words' to the 'query' i.e. query parameter this.set('query', words); } } });
Now use the queryParams configuration on the Route with the respective controller and set the replace config property to true in the paramreplaceState.js file created under app/routes/.
import Ember from 'ember'; export default Ember.Route.extend ({ queryParams: { query: { //assigning replace state as true replace: true } } });
Output
Run the ember server and you will receive the following output −

When you click on the link, it displays the button which sends an action to the addQuery method −

After clicking on the button, it will show the parameter value to the right of the "?" in a URL −

To Continue Learning Please Login