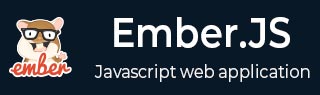
- EmberJS Tutorial
- EmberJS - Home
- EmberJS - Overview
- EmberJS - Installation
- EmberJS - Core Concepts
- Creating and Running Application
- EmberJS - Object Model
- EmberJS - Router
- EmberJS - Templates
- EmberJS - Components
- EmberJS - Models
- EmberJS - Managing Dependencies
- EmberJS - Application Concerns
- EmberJS - Configuring Ember.js
- EmberJS - Ember Inspector
- EmberJS Useful Resources
- EmberJS - Quick Guide
- EmberJS - Useful Resources
- EmberJS - Discussion
EmberJS- Computed Properties
Computed Properties
A computed property allows declaring functions as properties. The Ember.js automatically calls the computed properties when needed and combines one or more properties in one variable.
App.Car = Ember.Object.extend({ CarName: null, CarModel: null, fullDetails: function() { return this.get('CarName') + ' ' + this.get('CarModel'); }.property('CarName', 'CarModel') });
In the above code, fullDetails is a computed property which calls property() method to render the two values i.e. CarName and CarModel. Whenever fullDetails gets called, it returns CarName and CarModel.
Example
<!DOCTYPE html> <html> <head> <title>Emberjs Computed Properties</title> <!-- CDN's--> <script src="https://cdnjs.cloudflare.com/ajax/libs/handlebars.js/3.0.1/handlebars.min.js"></script> <script src="https://code.jquery.com/jquery-2.1.3.min.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/ember.js/1.10.0/ember.min.js"></script> <script src="https://builds.emberjs.com/tags/v1.10.0-beta.3/ember-template-compiler.js"></script> <script src="https://builds.emberjs.com/release/ember.debug.js"></script> <script src="https://builds.emberjs.com/beta/ember-data.js"></script> </head> <body> <script type="text/javascript"> App = Ember.Application.create(); App.Car = Ember.Object.extend({ //the values for below Variables to be supplied by `create` method CarName: null, CarModel: null, fullDetails: function(){ //returns values to the computed property function fullDetails return ' Car Name: '+this.get('CarName') + ' Car Model: ' + this.get('CarModel'); //property() method combines the variables of the Car }.property('CarName', 'CarModel') }); var car_obj = App.Car.create({ //initializing the values of Car variables CarName: "Alto", CarModel: "800", }); //Displaying the Car information document.write("Details of the car: <br>"); document.write(car_obj.get('fullDetails')); </script> </body> </html>
Output
Let's carry out the following steps to see how above code works −
Save above code in computed_prop.htm file
Open this HTML file in a browser.
The following table lists down the properties of the computed property −
S.N. | Properties & Description |
---|---|
1 |
It passes one or more values to the computed property without using the property() method. |
2 |
The chaining computed propertiy is used to aggregate with one or more predefined computed properties. |
3 |
Dynamically updates the computed property when they are called. |
4 |
Set up the computed properties by using the setter and getter. |
To Continue Learning Please Login