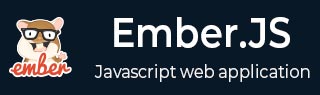
- EmberJS Tutorial
- EmberJS - Home
- EmberJS - Overview
- EmberJS - Installation
- EmberJS - Core Concepts
- Creating and Running Application
- EmberJS - Object Model
- EmberJS - Router
- EmberJS - Templates
- EmberJS - Components
- EmberJS - Models
- EmberJS - Managing Dependencies
- EmberJS - Application Concerns
- EmberJS - Configuring Ember.js
- EmberJS - Ember Inspector
- EmberJS Useful Resources
- EmberJS - Quick Guide
- EmberJS - Useful Resources
- EmberJS - Discussion
EmberJS- Computed Properties and Aggregate Data with @each
Computed Properties and Aggregate Data with @each
The computed property accesses all items in an array to determine its value. It makes simple add items to and remove the items from the array. The dependent key contains the special key @each and don't use nested form of @each. This updates the bindings and observer for the current computed property.
App.TodosController = Ember.Controller.extend ({ todos:[ Ember.Object.create({ isDone: true }), Ember.Object.create({ isDone: false }), Ember.Object.create({ isDone: true }) ], remaining: function() { var todos = this.get('todos'); return todos.filterBy('isDone', false).get('length'); }.property('todos.@each.isDone') });
In the above code, the todos.@each.isDone dependency key contains a special key @each. This key activates the observer when you add and remove the contents from the Todo. It will be updated in the remaining computed property.
Example
<!DOCTYPE html> <html> <head> <title>Emberjs Computed Properties and Aggregate Data with @Each</title> <!-- CDN's--> <script src="https://cdnjs.cloudflare.com/ajax/libs/handlebars.js/3.0.1/handlebars.min.js"></script> <script src="https://code.jquery.com/jquery-2.1.3.min.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/ember.js/1.10.0/ember.min.js"></script> <script src="https://builds.emberjs.com/tags/v1.10.0-beta.3/ember-template-compiler.js"></script> <script src="https://builds.emberjs.com/release/ember.debug.js"></script> <script src="https://builds.emberjs.com/beta/ember-data.js"></script> </head> <body> <script type="text/javascript"> App = Ember.Application.create(); //Extending the Ember.Controller App.TodosController = Ember.Controller.extend({ //todos is an array which holds the boolean values. todos: [ Ember.Object.create({ isDone: true }), Ember.Object.create({ isDone: false }), Ember.Object.create({ isDone: true }) ], //dispaly the remainig values of todos remaining: function() { var todos = this.get('todos'); //returnt the todos array. return todos.filterBy('isDone', false).get('length'); }.property('todos.@each.isDone') }); var car_obj = App.TodosController.create(); document.write("The remaining number of cars in todo list: "+car_obj.get('remaining')); </script> </body> </html>
Output
Let's carry out the following steps to see how above code works −
Save above code in computed_prop_agg_each.htm file
Open this HTML file in a browser.
Advertisements
To Continue Learning Please Login