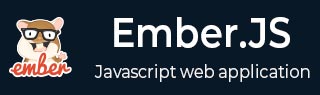
- EmberJS Tutorial
- EmberJS - Home
- EmberJS - Overview
- EmberJS - Installation
- EmberJS - Core Concepts
- Creating and Running Application
- EmberJS - Object Model
- EmberJS - Router
- EmberJS - Templates
- EmberJS - Components
- EmberJS - Models
- EmberJS - Managing Dependencies
- EmberJS - Application Concerns
- EmberJS - Configuring Ember.js
- EmberJS - Ember Inspector
- EmberJS Useful Resources
- EmberJS - Quick Guide
- EmberJS - Useful Resources
- EmberJS - Discussion
EmberJS- Pushing Records Into The Store
Pushing Records Into The Store
You can also push records into the store's cache without requesting the records from an application. The push() method helps to push the records into the store.
Ember.Route.extend({ model: function() { this.store.push(//do the logic) } });
In the above code, push() method pushes records into the store.
Example
<!DOCTYPE html> <html> <head> <title>Emberjs Pushing Records Into The Store</title> <!-- CDN's--> <script src="https://cdnjs.cloudflare.com/ajax/libs/handlebars.js/3.0.1/handlebars.min.js"></script> <script src="https://code.jquery.com/jquery-2.1.3.min.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/ember.js/1.10.0/ember.min.js"></script> <script src="https://builds.emberjs.com/tags/v1.10.0-beta.3/ember-template-compiler.js"></script> <script src="https://builds.emberjs.com/release/ember.debug.js"></script> <script src="https://builds.emberjs.com/beta/ember-data.js"></script> </head> <body> <script type="text/x-handlebars"> {{outlet}} </script> <script type="text/x-handlebars" data-template-name="index"> <h3>Fruit Name and Color: </h3> {{#each item in model}} <p>Color of the fruit: {{item.color}}<br> Name of the fruit: {{item.name}}</p> {{/each}} </script> <script type="text/javascript"> App = Ember.Application.create(); App.Color = DS.Model.extend({ //data model color: DS.attr(), name: DS.attr() }); App.ApplicationRoute = Ember.Route.extend({ model: function() { //push() method pushes records into the store this.store.push('color', { id: 1, color: "Red", name: "Apple" }); this.store.push('color', { id: 2, color: "Yellow", name: "Mango" }); //returns the all stored data return this.store.all('color'); } }); </script> </body> </html>
Output
Let's carry out the following steps to see how above code works −
Save above code in model_pushing_records.htm file
Open this HTML file in a browser.
Advertisements
To Continue Learning Please Login