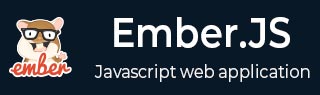
- EmberJS Tutorial
- EmberJS - Home
- EmberJS - Overview
- EmberJS - Installation
- EmberJS - Core Concepts
- Creating and Running Application
- EmberJS - Object Model
- EmberJS - Router
- EmberJS - Templates
- EmberJS - Components
- EmberJS - Models
- EmberJS - Managing Dependencies
- EmberJS - Application Concerns
- EmberJS - Configuring Ember.js
- EmberJS - Ember Inspector
- EmberJS Useful Resources
- EmberJS - Quick Guide
- EmberJS - Useful Resources
- EmberJS - Discussion
EmberJS-Creating and Deleting Records
Creating and Deleting Records
The records are created by using the Ember.js createRecord method and you can't assign promises as relationship currently.The this.store is available in the controllers and routes.
You can also delete the stored record by using deleteRecord() on any instance of DS.Model. This flags the record as isDeleted(true/false) and you can also persisted the deleted records using save().
store.createRecord('routeName',{ //declare the properties });
In the above code, 'routeName' is name of the template being used and declare properties for that 'routeName'.
Example
<!DOCTYPE html> <html> <head> <title>Emberjs Creating and Deleting Records</title> <!-- CDN's--> <script src="https://cdnjs.cloudflare.com/ajax/libs/handlebars.js/3.0.1/handlebars.min.js"></script> <script src="https://code.jquery.com/jquery-2.1.3.min.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/ember.js/1.10.0/ember.min.js"></script> <script src="https://builds.emberjs.com/tags/v1.10.0-beta.3/ember-template-compiler.js"></script> <script src="https://builds.emberjs.com/release/ember.debug.js"></script> <script src="https://builds.emberjs.com/beta/ember-data.js"></script> </head> <body> <script type="text/x-handlebars" data-template-name="fruits"> <h1>Enter Fruit names to Add</h1> {{input type="text" value=newTitle action="createFru"}} {{#each fru in model itemController="fru"}} <p><b>{{fru.title}}:</b></p> <button{{action "removeFru"}}>Click To Remove</button> {{/each}} </script> <script type="text/javascript"> Fruits = Ember.Application.create(); Fruits.ApplicationAdapter = DS.FixtureAdapter.extend(); Fruits.Router.map(function () { //fruits route this.resource('fruits', { path: '/' }); }); Fruits.FruitsRoute = Ember.Route.extend({ model: function () { return this.store.find('fru'); } }); Fruits.Fru = DS.Model.extend({ //data model title: DS.attr('string') }); //attach fixtures(sample data) to the model's class Fruits.Fru.FIXTURES = [{ id: 1, title: 'Apple' }]; Fruits.FruController = Ember.ObjectController.extend({ actions: { removeFru: function () { var delitem = this.get('model'); delitem.deleteRecord(); delitem.save(); } } }); Fruits.FruitsController = Ember.ArrayController.extend({ actions: { createFru: function () { // Get the fru title set by the textfield var title = this.get('newTitle'); // Create the new Fruits model var fru = this.store.createRecord('fru', { title: title }); // Save the new model fru.save(); } } }); </script> </body> </html>
Output
Let's carry out the following steps to see how above code works −
Save above code in models_crte_dlte.htm file
Open this HTML file in a browser.
Advertisements
To Continue Learning Please Login