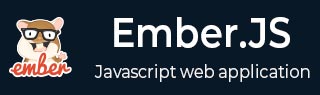
- EmberJS Tutorial
- EmberJS - Home
- EmberJS - Overview
- EmberJS - Installation
- EmberJS - Core Concepts
- Creating and Running Application
- EmberJS - Object Model
- EmberJS - Router
- EmberJS - Templates
- EmberJS - Components
- EmberJS - Models
- EmberJS - Managing Dependencies
- EmberJS - Application Concerns
- EmberJS - Configuring Ember.js
- EmberJS - Ember Inspector
- EmberJS Useful Resources
- EmberJS - Quick Guide
- EmberJS - Useful Resources
- EmberJS - Discussion
Invoking Actions on Component Collaborators
You can invoke actions on component collaborators directly from template.
Syntax
import Ember from 'ember'; export default Ember.Component.extend ({ target_attribute: Ember.inject.service(), // code for component implementation });
Example
The example given below specifies invoking actions on component collaborators directly from template in your application. Create a component with the name ember-actions and open the component template file ember-actions.js created under app/components/ with the following code −
import Ember from 'ember'; var inject = Ember.inject; export default Ember.Component.extend ({ message: inject.service(), text: 'This is test file', actions: { pressMe: function () { var testText = this.get('start').thisistest(); this.set('text', testText); } } });
Create a service, which can be made available in the different parts of the application. Use the following command to create the service −
ember generate service emberactionmessage
Now open the emberactionmessage.js service file, which is created under app/services/ with the following code −
import Ember from 'ember'; export default Ember.Service.extend ({ isAuthenticated: true, thisistest: function () { return "Welcome to Tutorialspoint"; } });
Next create an initializer, which configures the application as it boots. The initializer can be created by using the following command −
ember generate initializer init
Open the init.js initializer file, which is created under app/initializers/ with the following code −
export function initialize(app) { app.inject('component', 'start', 'service:emberactionmessage'); } export default { name: 'init', initialize: initialize };
Open the ember-actions.hbs file created under app/templates/components/ and enter the following code −
<button {{action "pressMe"}}>Click here to see the text</button><br> <h4>{{text}}</h4> {{yield}}
Create the application.hbs file and add the following code −
{{ember-actions}} {{outlet}}
Output
Run the ember server; you will receive the following output −

Next click on the button, it will display the text from the service page as shown in the screenshot below −
