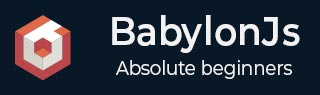
- BabylonJS Tutorial
- BabylonJS - Home
- BabylonJS - Introduction
- BabylonJS - Environment Setup
- BabylonJS - Overview
- BabylonJS - Basic Elements
- BabylonJS - Materials
- BabylonJS - Animations
- BabylonJS - Cameras
- BabylonJS - Lights
- BabylonJS - Parametric Shapes
- BabylonJS - Mesh
- VectorPosition and Rotation
- BabylonJS - Decals
- BabylonJS - Curve3
- BabylonJS - Dynamic Texture
- BabylonJS - Parallax Mapping
- BabylonJS - Lens Flares
- BabylonJS - Create ScreenShot
- BabylonJS - Reflection Probes
- Standard Rendering Pipeline
- BabylonJS - ShaderMaterial
- BabylonJS - Bones and Skeletons
- BabylonJS - Physics Engine
- BabylonJS - Playing Sounds & Music
- BabylonJS Useful Resources
- BabylonJS - Quick Guide
- BabylonJS - Useful Resources
- BabylonJS - Discussion
BabylonJS - Specular
The Specular property gives reflection like mirror when light falls on it. Specular offers two properties specularColor and specularTexture. The color and texture is noticed in the direction where light falls. It behaves like a spot light on the mesh. You can see the same in the demos below.
Syntax for specular color
materialforbox.specularColor = new BABYLON.Color3(1.0, 0.2, 0.7);
Syntax for specular texture
materialforbox.specularTexture = new BABYLON.Texture("grass.png", scene);
Demo for specular color
<!doctype html> <html> <head> <meta charset = "utf-8"> <title>BabylonJs - Basic Element-Creating Scene</title> <script src = "babylon.js"></script> <style> canvas {width: 100%; height: 100%;} </style> </head> <body> <canvas id = "renderCanvas"></canvas> <script type = "text/javascript"> var canvas = document.getElementById("renderCanvas"); var engine = new BABYLON.Engine(canvas, true); var createScene = function() { var scene = new BABYLON.Scene(engine); var camera = new BABYLON.ArcRotateCamera("Camera", 1, 0.8, 10, new BABYLON.Vector3(0, 0, 0), scene); camera.attachControl(canvas, true); var light = new BABYLON.HemisphericLight("light1", new BABYLON.Vector3(0, 1, 0), scene); light.intensity = 0.7; var materialforbox = new BABYLON.StandardMaterial("texture1", scene); var box = BABYLON.Mesh.CreateBox("box", '3', scene); box.material = materialforbox; materialforbox.specularColor = new BABYLON.Color3(1, 0.8, 0.8); return scene; }; var scene = createScene(); engine.runRenderLoop(function() { scene.render(); }); </script> </body> </html>
Output for specular color
The above line of code generates the following output −
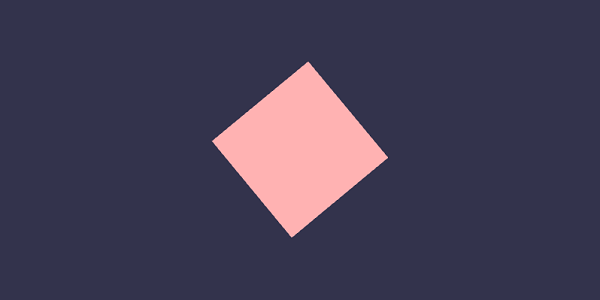
Demo for specular texture
<!doctype html> <html> <head> <meta charset = "utf-8"> <title>BabylonJs - Basic Element-Creating Scene</title> <script src = "babylon.js"></script> <style> canvas {width: 100%; height: 100%;} </style> </head> <body> <canvas id = "renderCanvas"></canvas> <script type = "text/javascript"> var canvas = document.getElementById("renderCanvas"); var engine = new BABYLON.Engine(canvas, true); var createScene = function() { var scene = new BABYLON.Scene(engine); var camera = new BABYLON.ArcRotateCamera("Camera", 1, 0.8, 10, new BABYLON.Vector3(0, 0, 0), scene); camera.attachControl(canvas, true); var light = new BABYLON.HemisphericLight("light1", new BABYLON.Vector3(0, 1, 0), scene); light.intensity = 0.7; var materialforbox = new BABYLON.StandardMaterial("texture1", scene); var box = BABYLON.Mesh.CreateBox("box", '3', scene); box.material = materialforbox; materialforbox.specularTexture = new BABYLON.Texture("images/rainbow.png", scene); return scene; }; var scene = createScene(); engine.runRenderLoop(function() { scene.render(); }); </script> </body> </html>
Output for specular texture
The above line of code generates the following output −
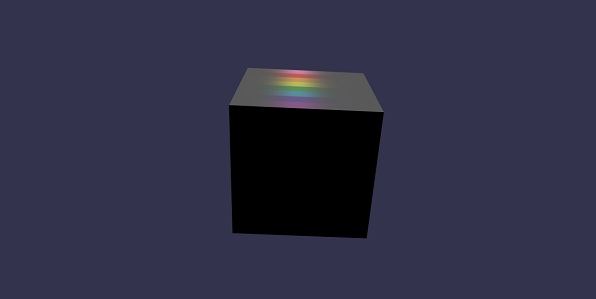
In this demo, we have used image called rainbow.png. The images are stored in images/ folder locally and also pasted below for reference. You can download any image of your choice and use in the demo link.
Texture used for box − images/rainbow.png
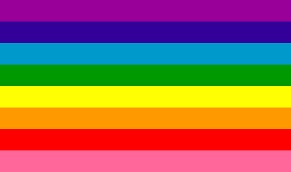
babylonjs_materials.htm
Advertisements