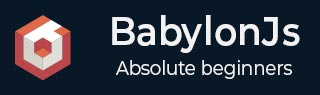
- BabylonJS Tutorial
- BabylonJS - Home
- BabylonJS - Introduction
- BabylonJS - Environment Setup
- BabylonJS - Overview
- BabylonJS - Basic Elements
- BabylonJS - Materials
- BabylonJS - Animations
- BabylonJS - Cameras
- BabylonJS - Lights
- BabylonJS - Parametric Shapes
- BabylonJS - Mesh
- VectorPosition and Rotation
- BabylonJS - Decals
- BabylonJS - Curve3
- BabylonJS - Dynamic Texture
- BabylonJS - Parallax Mapping
- BabylonJS - Lens Flares
- BabylonJS - Create ScreenShot
- BabylonJS - Reflection Probes
- Standard Rendering Pipeline
- BabylonJS - ShaderMaterial
- BabylonJS - Bones and Skeletons
- BabylonJS - Physics Engine
- BabylonJS - Playing Sounds & Music
- BabylonJS Useful Resources
- BabylonJS - Quick Guide
- BabylonJS - Useful Resources
- BabylonJS - Discussion
BabylonJS - Mirror and Bump Texture
With mirror texture, you get mirror like reflection of the meshes created.
Syntax for Mirror Texture
mirrorMaterial.reflectionTexture = new BABYLON.MirrorTexture("mirror", 512, scene, true); //Create a mirror texture mirrorMaterial.reflectionTexture.mirrorPlane = new BABYLON.Plane(0, -1.0, 0, -10.0);
Bump texture creates bump and wrinkles on the surface of the meshes the material is attached to.
Syntax for Bump Texture
bumpMaterial.bumpTexture = new BABYLON.Texture("images/btexture1.jpg", scene);
Demo
<!doctype html> <html> <head> <meta charset = "utf-8"> <title>BabylonJs - Basic Element-Creating Scene</title> <script src = "babylon.js"></script> <style> canvas {width: 100%; height: 100%;} </style> </head> <body> <canvas id = "renderCanvas"></canvas> <script type = "text/javascript"> var canvas = document.getElementById("renderCanvas"); var engine = new BABYLON.Engine(canvas, true); var createScene = function() { var scene = new BABYLON.Scene(engine); scene.clearColor = new BABYLON.Color3(0, 0, 1); var light = new BABYLON.PointLight("Omni", new BABYLON.Vector3(10, 10, 50), scene); var light2 = new BABYLON.PointLight("Omni", new BABYLON.Vector3(10, 10, -20), scene); var camera = new BABYLON.ArcRotateCamera("Camera", -Math.PI/2, Math.PI/4, 25, BABYLON.Vector3.Zero(), scene); camera.attachControl(canvas, true); var sphere1 = BABYLON.Mesh.CreateSphere("Sphere1", 16.0, 10.0, scene); var box1 = BABYLON.Mesh.CreateBox("box", 5.0, scene); var sphere3 = BABYLON.Mesh.CreateSphere("Sphere3", 16.0, 10.0, scene); var box2 = BABYLON.Mesh.CreateBox("box1", 3.0, scene); sphere1.position.x = -20; box1.position.x = 0; sphere3.position.x = 20; box2.position.z = 10; // Mirror var plane = BABYLON.Mesh.CreatePlane("plan", 70, scene); plane.position.y = -10; plane.rotation = new BABYLON.Vector3(Math.PI / 2, 0, 0); var bumpMaterial = new BABYLON.StandardMaterial("texture1", scene); bumpMaterial.diffuseColor = new BABYLON.Color3(0, 0, 1);//Blue bumpMaterial.bumpTexture = new BABYLON.Texture("images/btexture1.jpg", scene); var simpleMaterial = new BABYLON.StandardMaterial("texture2", scene); simpleMaterial.diffuseColor = new BABYLON.Color3(1, 0, 0);//Red var textMat = new BABYLON.StandardMaterial("texture3", scene); textMat.diffuseTexture = new BABYLON.Texture("images/btexture1.jpg", scene); // Multimaterial var multimat = new BABYLON.MultiMaterial("multi", scene); multimat.subMaterials.push(simpleMaterial); multimat.subMaterials.push(bumpMaterial); multimat.subMaterials.push(textMat); //Creation of a mirror material var mirrorMaterial = new BABYLON.StandardMaterial("texture4", scene); mirrorMaterial.diffuseColor = new BABYLON.Color3(0.4, 0.4, 0.4); mirrorMaterial.reflectionTexture = new BABYLON.MirrorTexture("mirror", 512, scene, true); //Create a mirror texture mirrorMaterial.reflectionTexture.mirrorPlane = new BABYLON.Plane(0, -1.0, 0, -10.0); mirrorMaterial.reflectionTexture.renderList = [sphere1, box1, sphere3, box2]; mirrorMaterial.reflectionTexture.level = 0.4;//Select the level (0.0 > 1.0) of the reflection box1.subMeshes = []; var verticesCount = box1.getTotalVertices(); box1.subMeshes.push(new BABYLON.SubMesh(0, 0, verticesCount, 0, 6, box1)); box1.subMeshes.push(new BABYLON.SubMesh(1, 1, verticesCount, 6, 6, box1)); box1.subMeshes.push(new BABYLON.SubMesh(2, 2, verticesCount, 12, 6, box1)); box1.subMeshes.push(new BABYLON.SubMesh(3, 3, verticesCount, 18, 6, box1)); box1.subMeshes.push(new BABYLON.SubMesh(4, 4, verticesCount, 24, 6, box1)); box1.subMeshes.push(new BABYLON.SubMesh(5, 5, verticesCount, 30, 6, box1)); plane.material = mirrorMaterial; sphere1.material = bumpMaterial; sphere3.material = simpleMaterial; box1.material = multimat;//simpleMaterial; return scene; }; var scene = createScene(); engine.runRenderLoop(function() { scene.render(); }); </script> </body> </html>
Output
The above line of code generates the following output −
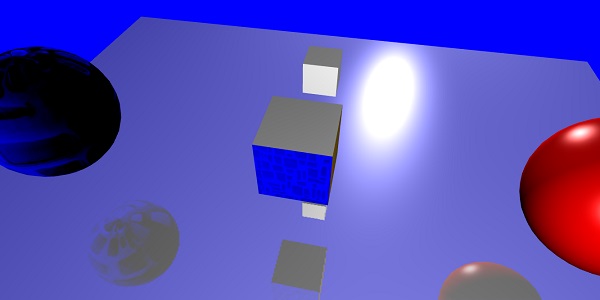
In this demo, we have used an image called btexture1.jpg. The images are stored in the images/ folder locally and are also pasted below for reference. You can download any image of your choice and use in the demo link.
images/btexture1.jpg
Following is the image used for bump structure −
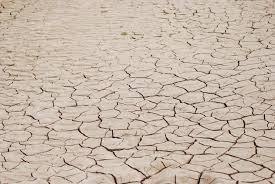
Explanation
First, we will create a standard material and to that we will apply bumptexture as follows −
bumpMaterial.bumpTexture = new BABYLON.Texture("images/btexture1.jpg", scene);
Create a standard material and apply mirror texture to it as follows −
mirrorMaterial.reflectionTexture = new BABYLON.MirrorTexture("mirror", 512, scene, true); //Create a mirror texture
For the same standard material, create a mirror plane and add the meshes to it which you need to see through the mirror as follows −
mirrorMaterial.reflectionTexture.mirrorPlane = new BABYLON.Plane(0, -1.0, 0, -10.0); mirrorMaterial.reflectionTexture.renderList = [sphere1, box1, sphere3, box2]; mirrorMaterial.reflectionTexture.level = 0.4;//Select the level (0.0 > 1.0) of the reflection