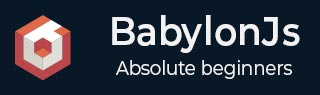
- BabylonJS Tutorial
- BabylonJS - Home
- BabylonJS - Introduction
- BabylonJS - Environment Setup
- BabylonJS - Overview
- BabylonJS - Basic Elements
- BabylonJS - Materials
- BabylonJS - Animations
- BabylonJS - Cameras
- BabylonJS - Lights
- BabylonJS - Parametric Shapes
- BabylonJS - Mesh
- VectorPosition and Rotation
- BabylonJS - Decals
- BabylonJS - Curve3
- BabylonJS - Dynamic Texture
- BabylonJS - Parallax Mapping
- BabylonJS - Lens Flares
- BabylonJS - Create ScreenShot
- BabylonJS - Reflection Probes
- Standard Rendering Pipeline
- BabylonJS - ShaderMaterial
- BabylonJS - Bones and Skeletons
- BabylonJS - Physics Engine
- BabylonJS - Playing Sounds & Music
- BabylonJS Useful Resources
- BabylonJS - Quick Guide
- BabylonJS - Useful Resources
- BabylonJS - Discussion
BabylonJS - Mesh FacetData
Facet data takes up a lot of memory and this feature is not enabled by default. To enable it, we need to create a mesh as required and update facet data to it. Consider the following example to understand this −
mesh.updateFacetData();
A mesh can have some planar faces. For example, a box has 6 sides, so 6 planar squared faces. Each of its faces are drawn at the WebGL level with 2 triangles.
var positions = mesh.getFacetLocalPositions(); // returns the array of facet positions in the local space var normals = mesh.getFacetLocalNormals(); // returns the array of facet normals in the local space
Using the co-ordinates of the normal,we will draw triangle on the facet normals on the sphere.
Demo
<!doctype html> <html> <head> <meta charset = "utf-8"> <title>BabylonJs - Basic Element-Creating Scene</title> <script src = "babylon.js"></script> <style> canvas {width: 100%; height: 100%;} </style> </head> <body> <canvas id = "renderCanvas"></canvas> <script type = "text/javascript"> var canvas = document.getElementById("renderCanvas"); var engine = new BABYLON.Engine(canvas, true); var createScene = function() { var scene = new BABYLON.Scene(engine); scene.clearColor = new BABYLON.Color3(0.35, 0.35, 0.42); var camera = new BABYLON.ArcRotateCamera("Camera", 0, 0, 0, BABYLON.Vector3.Zero(), scene); camera.attachControl(canvas, true); camera.setPosition(new BABYLON.Vector3(0.0, 3.0, -8.0)); var light = new BABYLON.HemisphericLight('light1', new BABYLON.Vector3(0, 1, 0), scene); light.intensity = 0.2; var pl = new BABYLON.PointLight('pl', camera.position, scene); pl.intensity = 0.9; var mesh = BABYLON.MeshBuilder.CreateIcoSphere("m", {radius: 2.0}, scene); mesh.updateFacetData(); var positions = mesh.getFacetLocalPositions(); var normals = mesh.getFacetLocalNormals(); var cone = []; var matcone = []; var texture = []; for (var i = 0; i < positions.length; i++) { console.log(positions[i].add(normals[i]).x); matcone[i] = new BABYLON.StandardMaterial("mat1", scene); matcone[i].alpha = 1.0; matcone[i].diffuseColor = new BABYLON.Color3(0.9, 0, 2); texture[i] = new BABYLON.Texture("images/cone.jpg", scene); matcone[i].diffuseTexture = texture[i]; cone[i] = BABYLON.MeshBuilder.CreateDisc("disc", {tessellation: 3}, scene); cone[i].position= new BABYLON.Vector3(positions[i].add(normals[i]).x,positions[i].add(normals[i]).y,positions[i].add(normals[i]).z); cone[i].material = matcone[i]; } return scene }; var scene = createScene(); engine.runRenderLoop(function() { scene.render(); }); </script> </body> </html>
Output
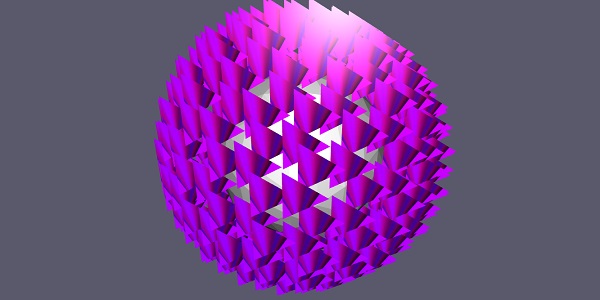
In this demo, we have used image cone.jpg. The images are stored in the images/ folder locally and are also pasted below for reference. You can download any image of your choice and use in the demo link.
images/cone.jpg
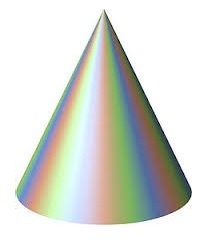
babylonjs_mesh.htm
Advertisements