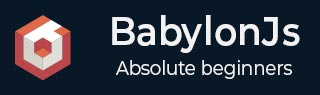
- BabylonJS Tutorial
- BabylonJS - Home
- BabylonJS - Introduction
- BabylonJS - Environment Setup
- BabylonJS - Overview
- BabylonJS - Basic Elements
- BabylonJS - Materials
- BabylonJS - Animations
- BabylonJS - Cameras
- BabylonJS - Lights
- BabylonJS - Parametric Shapes
- BabylonJS - Mesh
- VectorPosition and Rotation
- BabylonJS - Decals
- BabylonJS - Curve3
- BabylonJS - Dynamic Texture
- BabylonJS - Parallax Mapping
- BabylonJS - Lens Flares
- BabylonJS - Create ScreenShot
- BabylonJS - Reflection Probes
- Standard Rendering Pipeline
- BabylonJS - ShaderMaterial
- BabylonJS - Bones and Skeletons
- BabylonJS - Physics Engine
- BabylonJS - Playing Sounds & Music
- BabylonJS Useful Resources
- BabylonJS - Quick Guide
- BabylonJS - Useful Resources
- BabylonJS - Discussion
BabylonJS - Diffuse
The Diffuse property spreads the color all over the mesh to which it is attached.
Example
Consider the following example to understand how this works.
Syntax
materialforbox.diffuseColor = new BABYLON.Color3(1.0, 0.2, 0.7);
Demo
<!doctype html> <html> <head> <meta charset = "utf-8"> <title>BabylonJs - Basic Element-Creating Scene</title> <script src = "babylon.js"></script> <style> canvas {width: 100%; height: 100%;} </style> </head> <body> <canvas id = "renderCanvas"></canvas> <script type = "text/javascript"> var canvas = document.getElementById("renderCanvas"); var engine = new BABYLON.Engine(canvas, true); var createScene = function() { var scene = new BABYLON.Scene(engine); scene.clearColor = new BABYLON.Color3(0, 1, 0); var camera = new BABYLON.ArcRotateCamera("Camera", 1, 0.8, 10, new BABYLON.Vector3(0, 0, 0), scene); camera.attachControl(canvas, true); var light = new BABYLON.HemisphericLight("light1", new BABYLON.Vector3(0, 1, 0), scene); light.intensity = 0.7; var materialforbox = new BABYLON.StandardMaterial("texture1", scene); var box = BABYLON.Mesh.CreateBox("box", '3', scene); box.material = materialforbox; materialforbox.diffuseColor = new BABYLON.Color3(1.0, 0.7, 0.3); // color is applied to he box return scene; }; var scene = createScene(); engine.runRenderLoop(function() { scene.render(); }); </script> </body> </html>
Output
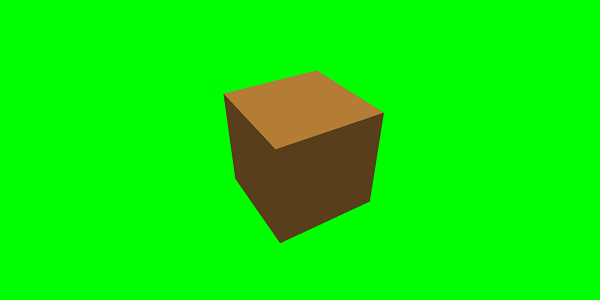
With the standard material, you can apply color to the shape it is attached to.
Demo with texture
The textures are created using an image.
<!doctype html> <html> <head> <meta charset = "utf-8"> <title>BabylonJs - Basic Element-Creating Scene</title> <script src = "babylon.js"></script> <style> canvas {width: 100%; height: 100%;} </style> </head> <body> <canvas id = "renderCanvas"></canvas> <script type = "text/javascript"> var canvas = document.getElementById("renderCanvas"); var engine = new BABYLON.Engine(canvas, true); var createScene = function() { var scene = new BABYLON.Scene(engine); scene.clearColor = new BABYLON.Color3(0, 1, 0); var camera = new BABYLON.ArcRotateCamera("Camera", 1, 0.8, 10, new BABYLON.Vector3(0, 0, 0), scene); camera.attachControl(canvas, true); var light = new BABYLON.HemisphericLight("light1", new BABYLON.Vector3(0, 1, 0), scene); light.intensity = 0.7; var materialforbox = new BABYLON.StandardMaterial("texture1", scene); var box = BABYLON.Mesh.CreateBox("box", '3', scene); box.material = materialforbox; materialforbox.diffuseTexture = new BABYLON.Texture("images/nature.jpg", scene); return scene; }; var scene = createScene(); engine.runRenderLoop(function() { scene.render(); }); </script> </body> </html>
Output
The above line of code generates the following output −
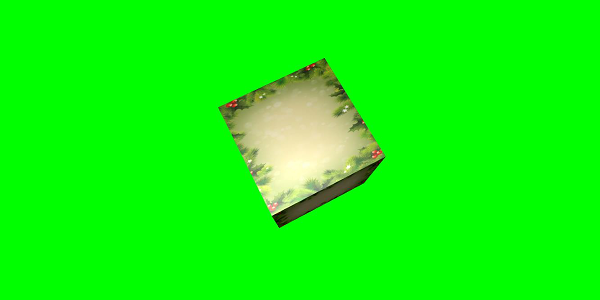
In this demo, we have used an image called nature.jpg. The images are stored in images/ folder locally and also pasted below for reference. You can download any image of your choice and use in the demo link.
We have used the following image for the box − images/nature.jpg
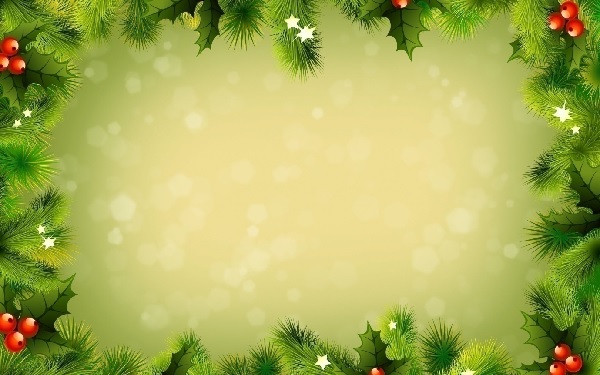
Diffuse using offsets
Lets us now see how to diffuse using offsets.
Syntax
materialforbox.diffuseTexture.uOffset = 0.5; materialforbox.diffuseTexture.vOffset = 0.5;
U and v refers to the x & y axis −
<!doctype html> <html> <head> <meta charset = "utf-8"> <title>BabylonJs - Basic Element-Creating Scene</title> <script src = "babylon.js"></script> <style> canvas {width: 100%; height: 100%;} </style> </head> <body> <canvas id = "renderCanvas"></canvas> <script type = "text/javascript"> var canvas = document.getElementById("renderCanvas"); var engine = new BABYLON.Engine(canvas, true); var createScene = function() { var scene = new BABYLON.Scene(engine); scene.clearColor = new BABYLON.Color3(0, 1, 0); var camera = new BABYLON.ArcRotateCamera("Camera", 1, 0.8, 10, new BABYLON.Vector3(0, 0, 0), scene); camera.attachControl(canvas, true); var light = new BABYLON.HemisphericLight("light1", new BABYLON.Vector3(0, 1, 0), scene); light.intensity = 0.7; var materialforbox = new BABYLON.StandardMaterial("texture1", scene); var box = BABYLON.Mesh.CreateBox("box", '3', scene); box.material = materialforbox; materialforbox.diffuseTexture = new BABYLON.Texture("images/nature.jpg", scene); materialforbox.diffuseTexture.uOffset = 0.5; materialforbox.diffuseTexture.vOffset = 0.5; return scene; }; var scene = createScene(); engine.runRenderLoop(function() { scene.render(); }); </script> </body> </html>
Output
The above line of code generates the following output −
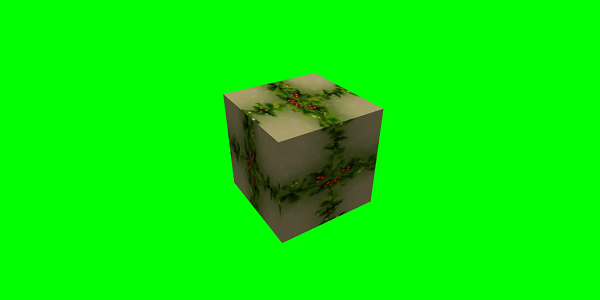
In this demo, we have used an image called nature.jpg. The images are stored in images/ folder locally. Here, we have used the same image as in the previous demo. The image is already pasted above for reference. You can download any image of your choice and use in the demo link.
Diffuse with scaling
Let us now see how to diffuse with scaling.
Syntax
materialforbox.diffuseTexture.uScale = 5.0; materialforbox.diffuseTexture.vScale = 5.0;
Demo
<!doctype html> <html> <head> <meta charset = "utf-8"> <title>BabylonJs - Basic Element-Creating Scene</title> <script src = "babylon.js"></script> <style> canvas {width: 100%; height: 100%;} </style> </head> <body> <canvas id = "renderCanvas"></canvas> <script type = "text/javascript"> var canvas = document.getElementById("renderCanvas"); var engine = new BABYLON.Engine(canvas, true); var createScene = function() { var scene = new BABYLON.Scene(engine); scene.clearColor = new BABYLON.Color3(0, 1, 0); var camera = new BABYLON.ArcRotateCamera("Camera", 1, 0.8, 10, new BABYLON.Vector3(0, 0, 0), scene); camera.attachControl(canvas, true); var light = new BABYLON.HemisphericLight("light1", new BABYLON.Vector3(0, 1, 0), scene); light.intensity = 0.7; var materialforbox = new BABYLON.StandardMaterial("texture1", scene); var box = BABYLON.Mesh.CreateBox("box", '3', scene); box.material = materialforbox; materialforbox.diffuseTexture = new BABYLON.Texture("images/nature.jpg", scene); materialforbox.diffuseTexture.uOffset = 0.5; materialforbox.diffuseTexture.vOffset = 0.5; materialforbox.diffuseTexture.uScale = 5.0; materialforbox.diffuseTexture.vScale = 5.0; return scene; }; var scene = createScene(); engine.runRenderLoop(function() { scene.render(); }); </script> </body> </html>
Output
The above line of code generates the following output −
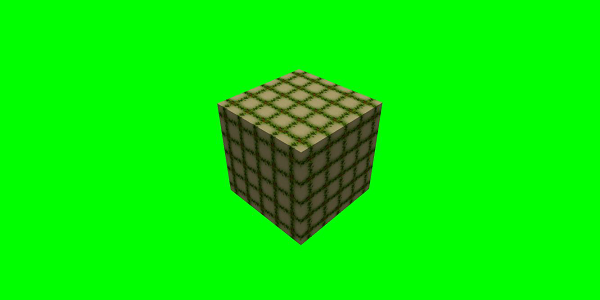
In this demo, we have used an image called nature.jpg. The images are stored in images/ folder locally. We have used the same image as in a previous demo. The image is already pasted above for reference. You can download any image of your choice and use in the demo link.