
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9053 Articles for Front End Technology

102 Views
To find multiple matches, write a JavaScript regular expression. You can try to run the following code to implement regular expression for multiple matches −Example var url = 'https://www.example.com/new.html?ui=7&demo=one&demo=two&demo=three four', a = document.createElement('a'); a.href = url; var demoRegex = /(?:^|[&;])demo=([^&;]+)/g, matches, demo = []; while (matches = demoRegex.exec(a.search)) { demo.push(decodeURIComponent(matches[1])); } document.write(demo);

161 Views
To remove spaces in JavaScript, you can try to run the following regular expression. It removes the spaces from a string −Example Live Demo var str = "Welcome to Tutorialspoint"; document.write(str); //Removing Spaces document.write(""+str.replace(/\s/g, '')); Output

2K+ Views
In this tutorial, we will explore how we can replace a particular sub string in a string by using regular expressions in JavaScript. Sometimes we may want to replace a recurrent sub string in a string with something else. In such cases, regular expressions can be beneficial. Before using them to solve our current problem, let us see what regular expressions exactly are. Regular expressions are basically a pattern of characters that can be used to search different occurrences of that pattern in a string. Regular expressions make it possible to search for a particular pattern in a stream of ... Read More

104 Views
JavaScript uses try…catch…finally to handle exceptions. The latest versions of JavaScript added exception-handling capabilities. JavaScript implements the try...catch...finally construct as well as the throw operator to handle exceptions.You can catch programmer-generated and runtime exceptions, but you cannot catch JavaScript syntax errors.SyntaxHere is the try...catch...finally block syntax − ExampleYou can try to run the following code to learn how exceptions are handled in JavaScript − Click the following to see the result:

363 Views
In JavaScript, the functions wrapped with parenthesis are called “Immediately Invoked Function Expressions" or "Self Executing Functions.The purpose of wrapping is to the namespace and control the visibility of member functions. It wraps the code inside a function scope and decreases clashing with other libraries. This is what we call Immediately Invoked Function Expression (IIFE) or Self Executing Anonymous Function.SyntaxHere’s the syntax −(function() { // code })();As you can see above, the following pair of parentheses converts the code inside the parentheses into an expression −function(){...}In addition, the next pair, i.e. the second pair of parentheses continues the operation. ... Read More

101 Views
The Function() constructor expects any number of string arguments. The last argument is the body of the function - it can contain arbitrary JavaScript statements, separated from each other by semicolons.ExampleYou can try to run the following code to invoke a function with new Function Constructor − var func = new Function("x", "y", "return x*y;"); function multiplyFunction(){ var result; result = func(15,35); document.write ( result ); } Click the following button to call the function
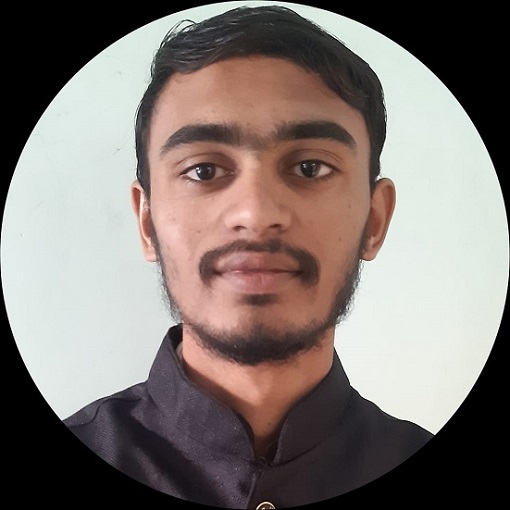
703 Views
In this tutorial, we will learn the different ways of defining functions in JavaScript. In programming, writing the same code again and again is not a great idea. It will increase the length and decrease the readability with more effort required for the program. The functions are there in the programming languages to make it easier for programmers. The function is like a container containing certain lines of programs inside. The function does not execute by only defining it. We have to call it somewhere in the program by which it can run the code it consists of. We can ... Read More

156 Views
To declare optional function parameters in JavaScript, use the “default” arguments.ExampleYou can try to run the following code to declare optional parameters − // default is set to 1 function inc(val1, inc = 1) { return val1 + inc; } document.write(inc(10,10)); document.write(""); document.write(inc(10));
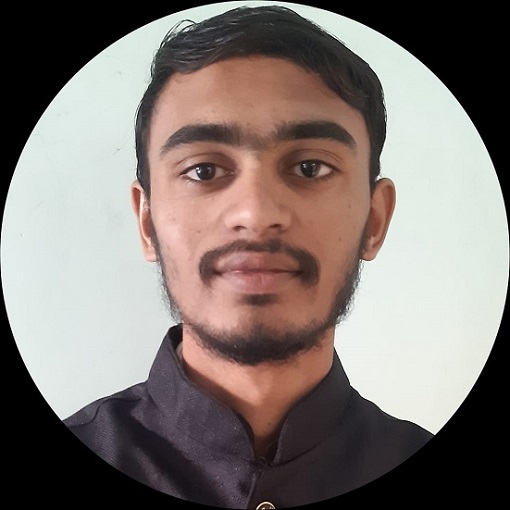
148 Views
In this tutorial, we will learn what "use strict" does in JavaScript. The "use strict" is a directive. JavaScript 1.8.5 is its origin. The use strict directive says that the JavaScript code must run in the strict mode. The strict directive is not a statement but rather a constant expression. Earlier JavaScript versions ignore this expression. We can't use undeclared variables when writing the code in strict mode. Except for IE9 and lower versions, all modern browsers support this JavaScript directive. Strict mode allows us to write a cleaner code as it throws errors when we use undeclared variables in ... Read More
To Continue Learning Please Login