
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9053 Articles for Front End Technology
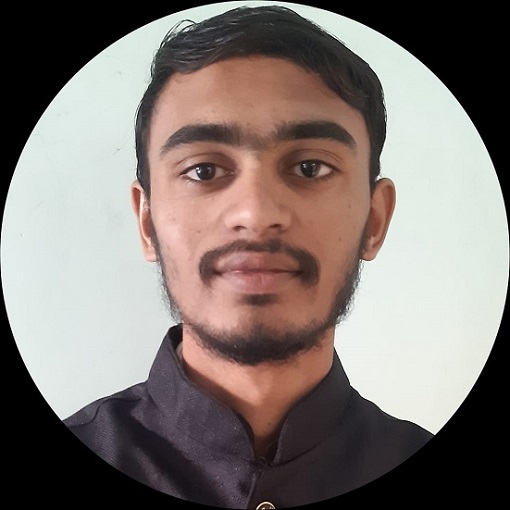
8K+ Views
HTML contains special characters such as ‘, ’ ‘/, ’ and many more such as single and double commas. These special characters are used for the HTML tag, such as ‘’ is used to close the HTML tag. This tutorial teaches us to escape HTML special characters in JavaScript.Now, the question is that what if we want to use these characters inside the HTML content? If we use special characters normally in the HTML content, it considers it the opening or closing HTML tag and produces an unknown error.For example, we need to render the below string to the browser. ... Read More

476 Views
The isNan() method is used in JavaScript to check whether there’s the existence of an undefined value or can say checks for a NaN object. ExampleTo check whether a JavaScript date is valid or not, you can try to run the following code − var date1, date2; date1 = new Date("2018/1/1"); if( ! isNaN ( date1.getMonth() )) { document.write("Valid date1: "+date1); } else { document.write("Invalid date1"); } date2 = new Date("20181/1"); if( ! isNaN ( date2.getMonth() )) { document.write(" Valid date2: "+date2); } else { document.write("Invalid date2"); } The isNaN(null) == false is semantically correct. This is because null is not NaN.

104 Views
Try any of the following for the width of the image you want to create −myImg.setAttribute('width', '5px');OrmyImg.width = '5';OrmyImg.style.width = '5px';You can also try the following code to add the width and height to the background image −var myImg = new Image(5,5); myImg.src = 'http://www.example.com';

923 Views
To get only the else part, just negate, using ! the operator in JavaScript. You can try to run the following code to achieve this −var arr = {}; if (!(id in arr)) { } else { }In addition, if you want the existence of a property in an object, then use hasOwnProperty −if (!arr.hasOwnProperty(id)) { } else { }

472 Views
Use the keydown event in JavaScript to get to know which keys are pressed at once. The following is the script −Examplevar log = $('#log')[0], keyPressed = []; $(document.body).keydown(function (evt) { var li = keyPressed [evt.keyCode]; if (!li) { li = log.appendChild(document.createElement('li')); keyPressed [evt.keyCode] = li; } $(li).text(Key Down: ' + evt.keyCode); $(li).removeClass('key-up'); }); $(document.body).keyup(function (evt) { var li = keyPressed [evt.keyCode]; if (!li) { li = log.appendChild(document.createElement('li')); } $(li).text('Key Up: ' + evt.keyCode); $(li).addClass('key-up'); });
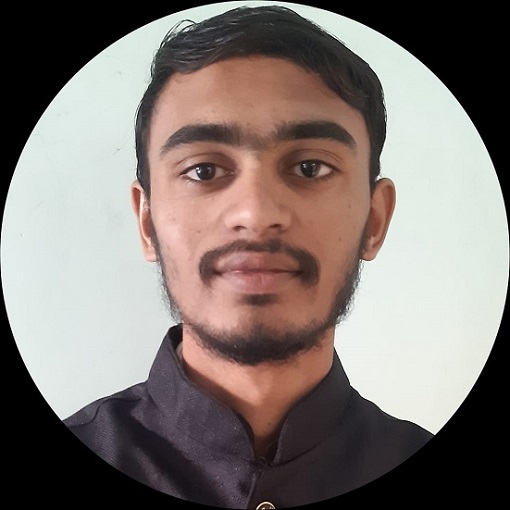
191 Views
In this tutorial, we shall learn how to set the style of the left border with JavaScript. To set the style of the left border in JavaScript, use the borderLeftStyle property. Set the style under this property that you want for the border i.e. solid, dashed, etc. Let us discuss our topic in brief. Using the borderLeftStyl Property With this property, we can set or return the style of the left border of an element. Users can follow the syntax below for using this property. Syntax object.style.borderLeftStyle = style; This syntax allows the required border style to be ... Read More
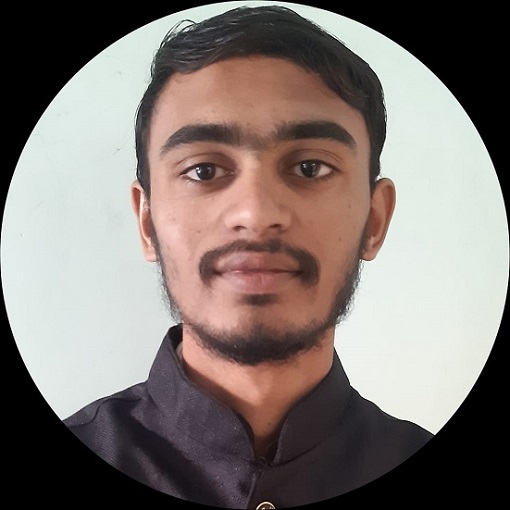
155 Views
In this tutorial, we will learn how and why ‘z’[‘toUpperCase’]() works in JavaScript. From the given format, we can say that it calls the toUpperCase() method for the ‘z’ string. It will work the same as the toUpperCase() method, which we invoke by taking any string as a reference. Let’s understand the syntax of the ‘z’[‘toUpperCase’]() below. Syntax let result = 'z'['toUpperCase'](); // returns 'Z', string in upper case The above syntax is same as the below. Example let string = 'z'; let result = string.toUpperCase(); Why does ‘z’[‘toUpperCase’]() work? In JavaScript toUpperCase() method is used to convert ... Read More
To Continue Learning Please Login