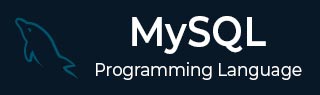
- MySQL Basics
- MySQL - Home
- MySQL - Introduction
- MySQL - Features
- MySQL - Versions
- MySQL - Variables
- MySQL - Installation
- MySQL - Administration
- MySQL - PHP Syntax
- MySQL - Node.js Syntax
- MySQL - Java Syntax
- MySQL - Python Syntax
- MySQL - Connection
- MySQL - Workbench
- MySQL Databases
- MySQL - Create Database
- MySQL - Drop Database
- MySQL - Select Database
- MySQL - Show Database
- MySQL - Copy Database
- MySQL - Database Export
- MySQL - Database Import
- MySQL - Database Info
- MySQL Users
- MySQL - Create Users
- MySQL - Drop Users
- MySQL - Show Users
- MySQL - Change Password
- MySQL - Grant Privileges
- MySQL - Show Privileges
- MySQL - Revoke Privileges
- MySQL - Lock User Account
- MySQL - Unlock User Account
- MySQL Tables
- MySQL - Create Tables
- MySQL - Show Tables
- MySQL - Alter Tables
- MySQL - Rename Tables
- MySQL - Clone Tables
- MySQL - Truncate Tables
- MySQL - Temporary Tables
- MySQL - Repair Tables
- MySQL - Describe Tables
- MySQL - Add/Delete Columns
- MySQL - Show Columns
- MySQL - Rename Columns
- MySQL - Table Locking
- MySQL - Drop Tables
- MySQL - Derived Tables
- MySQL Queries
- MySQL - Queries
- MySQL - Constraints
- MySQL - Insert Query
- MySQL - Select Query
- MySQL - Update Query
- MySQL - Delete Query
- MySQL - Replace Query
- MySQL - Insert Ignore
- MySQL - Insert on Duplicate Key Update
- MySQL - Insert Into Select
- MySQL Indexes
- MySQL - Indexes
- MySQL - Create Index
- MySQL - Drop Index
- MySQL - Show Indexes
- MySQL - Unique Index
- MySQL - Clustered Index
- MySQL - Non-Clustered Index
- MySQL Operators and Clauses
- MySQL - Where Clause
- MySQL - Limit Clause
- MySQL - Distinct Clause
- MySQL - Order By Clause
- MySQL - Group By Clause
- MySQL - Having Clause
- MySQL - AND Operator
- MySQL - OR Operator
- MySQL - Like Operator
- MySQL - IN Operator
- MySQL - ANY Operator
- MySQL - EXISTS Operator
- MySQL - NOT Operator
- MySQL - NOT EQUAL Operator
- MySQL - IS NULL Operator
- MySQL - IS NOT NULL Operator
- MySQL - Between Operator
- MySQL - UNION Operator
- MySQL - UNION vs UNION ALL
- MySQL - MINUS Operator
- MySQL - INTERSECT Operator
- MySQL - INTERVAL Operator
- MySQL Joins
- MySQL - Using Joins
- MySQL - Inner Join
- MySQL - Left Join
- MySQL - Right Join
- MySQL - Cross Join
- MySQL - Full Join
- MySQL - Self Join
- MySQL - Delete Join
- MySQL - Update Join
- MySQL - Union vs Join
- MySQL Keys
- MySQL - Unique Key
- MySQL - Primary Key
- MySQL - Foreign Key
- MySQL - Composite Key
- MySQL - Alternate Key
- MySQL Triggers
- MySQL - Triggers
- MySQL - Create Trigger
- MySQL - Show Trigger
- MySQL - Drop Trigger
- MySQL - Before Insert Trigger
- MySQL - After Insert Trigger
- MySQL - Before Update Trigger
- MySQL - After Update Trigger
- MySQL - Before Delete Trigger
- MySQL - After Delete Trigger
- MySQL Data Types
- MySQL - Data Types
- MySQL - VARCHAR
- MySQL - BOOLEAN
- MySQL - ENUM
- MySQL - DECIMAL
- MySQL - INT
- MySQL - FLOAT
- MySQL - BIT
- MySQL - TINYINT
- MySQL - BLOB
- MySQL - SET
- MySQL Regular Expressions
- MySQL - Regular Expressions
- MySQL - RLIKE Operator
- MySQL - NOT LIKE Operator
- MySQL - NOT REGEXP Operator
- MySQL - regexp_instr() Function
- MySQL - regexp_like() Function
- MySQL - regexp_replace() Function
- MySQL - regexp_substr() Function
- MySQL Fulltext Search
- MySQL - Fulltext Search
- MySQL - Natural Language Fulltext Search
- MySQL - Boolean Fulltext Search
- MySQL - Query Expansion Fulltext Search
- MySQL - ngram Fulltext Parser
- MySQL Functions & Operators
- MySQL - Date and Time Functions
- MySQL - Arithmetic Operators
- MySQL - Numeric Functions
- MySQL - String Functions
- MySQL - Aggregate Functions
- MySQL Misc Concepts
- MySQL - NULL Values
- MySQL - Transactions
- MySQL - Using Sequences
- MySQL - Handling Duplicates
- MySQL - SQL Injection
- MySQL - SubQuery
- MySQL - Comments
- MySQL - Check Constraints
- MySQL - Storage Engines
- MySQL - Export Table into CSV File
- MySQL - Import CSV File into Database
- MySQL - UUID
- MySQL - Common Table Expressions
- MySQL - On Delete Cascade
- MySQL - Upsert
- MySQL - Horizontal Partitioning
- MySQL - Vertical Partitioning
- MySQL - Cursor
- MySQL - Stored Functions
- MySQL - Signal
- MySQL - Resignal
- MySQL - Character Set
- MySQL - Collation
- MySQL - Wildcards
- MySQL - Alias
- MySQL - ROLLUP
- MySQL - Today Date
- MySQL - Literals
- MySQL - Stored Procedure
- MySQL - Explain
- MySQL - JSON
- MySQL - Standard Deviation
- MySQL - Find Duplicate Records
- MySQL - Delete Duplicate Records
- MySQL - Select Random Records
- MySQL - Show Processlist
- MySQL - Change Column Type
- MySQL - Reset Auto-Increment
- MySQL - Coalesce() Function
MySQL − Insert on Duplicate Key Update
The INSERT INTO statement in MySQL is used to insert new records into a specific table.
MySQL Insert on Duplicate Key Update Statement
When we are trying to insert a new row into a MySQL table column with a UNIQUE INDEX or PRIMARY KEY, MySQL will issue an error, if the value being inserted already exists in the column. This will happen because these constraints require unique values, and duplicate values are not allowed.
However, if we use the MySQL ON DUPLICATE KEY UPDATE clause with with the INSERT INTO statement, MySQL will update the existing rows with the new values instead of showing an error.
Syntax
Following is the basic syntax of ON DUPLICATE KEY UPDATE clause in MySQL −
INSERT INTO my_table (col1, col2, ...) VALUES (val1, val2), (val3, val4), ... ON DUPLICATE KEY UPDATE <col1>=<val1>, <col2>=<val2>,...;
Example
First of all, let us create a table named CUSTOMERS using the following query −
CREATE TABLE CUSTOMERS ( ID int NOT NULL, NAME varchar(20) NOT NULL, AGE int NOT NULL, ADDRESS char (25), SALARY decimal (18, 2), PRIMARY KEY (ID) );
Here, we are inserting some records into the above-created table using the INSERT INTO statement as shown below −
INSERT INTO CUSTOMERS (ID,NAME,AGE,ADDRESS,SALARY) VALUES (1, 'Ramesh', 32, 'Ahmedabad', 2000.00 ), (2, 'Khilan', 25, 'Delhi', 1500.00 ), (3, 'Kaushik', 23, 'Kota', 2000.00 );
Execute the following query to display the records present in the above created CUSTOMERS table −
SELECT * FROM CUSTOMERS;
Following are the records in CUSTOMERS table −
ID | NAME | AGE | ADDRESS | SALARY |
---|---|---|---|---|
1 | Ramesh | 32 | Ahmedabad | 2000.00 |
2 | Khilan | 25 | Delhi | 1500.00 |
3 | Kaushik | 23 | Kota | 2000.00 |
Here, we are inserting another row into the CUSTOMERS table with an ID value 3 using the INSERT INTO statement −
INSERT INTO CUSTOMERS (ID, NAME, AGE, ADDRESS, SALARY) VALUES (3, 'Chaitali', 25, 'Mumbai', 6500.00);
As a result, MySQL will issue an error because we are inserting a duplicate ID value −
ERROR 1062 (23000): Duplicate entry '3' for key 'customers.PRIMARY'
We can avoid the above error and update the existing row with the new information using the ON DUPLICATE KEY UPDATE clause along with INSERT INTO statement as shown below −
INSERT INTO CUSTOMERS (ID, NAME, AGE, ADDRESS, SALARY) VALUES (3, 'Chaitali', 25, 'Mumbai', 6500.00) ON DUPLICATE KEY UPDATE NAME = "Chaitali", AGE = 25, ADDRESS = "Mumbai", SALARY = 6500.00;
Output
As we can see in the output, the above query updated the existing row in the CUSTOMERS table. As a result, it returns two affected-rows.
Query OK, 2 rows affected (0.01 sec)
Verification
Execute the following query to verify whether the existing row got updated with new information or not −
SELECT * FROM CUSTOMERS;
As we observe the third row in the table, the records got updated.
ID | NAME | AGE | ADDRESS | SALARY |
---|---|---|---|---|
1 | Ramesh | 32 | Ahmedabad | 2000.00 |
2 | Khilan | 25 | Delhi | 1500.00 |
3 | Chaitali | 25 | Mumbai | 6500.00 |
Example
In the following query, we are trying to insert a new row into the CUSTOMERS table using the INSERT INTO statement along with the ON DUPLICATE KEY UPDATE clause −
INSERT INTO CUSTOMERS (ID, NAME, AGE, ADDRESS, SALARY) VALUES (4, 'Hardik', 27, 'Bhopal', 8500.00) ON DUPLICATE KEY UPDATE NAME = "Hardik", AGE = 27, ADDRESS = "Bhopal", SALARY = 8500.00;
Output
As we can see in the output, there is no conflict occurred while inserting the new row. As a result, it returns one affected-row.
Query OK, 1 row affected (0.01 sec)
Verification
We can verify whether the new row is inserted in the CUSTOMERS table or not using the following query −
SELECT * FROM CUSTOMERS;
As we observe the output below, the new row has been inserted.
ID | NAME | AGE | ADDRESS | SALARY |
---|---|---|---|---|
1 | Ramesh | 32 | Ahmedabad | 2000.00 |
2 | Khilan | 25 | Delhi | 1500.00 |
3 | Chaitali | 25 | Mumbai | 6500.00 |
4 | Hardik | 27 | Bhopal | 8500.00 |
INSERT or UPDATE multiple records at once
While inserting or updating multiple records at the same time in MySQL, the value to set for each column may vary depending on which record or records have a conflict.
For example, if we are trying to insert four new rows, but the third has an ID column that conflicts with an existing record, we most likely want to update the existing row based on the data you had in mind for the third row.
Example
Before we perform the next operation, let's look into the records of updated CUSTOMERS table −
SELECT * FROM CUSTOMERS;
Following is the updated CUSTOMERS table −
ID | NAME | AGE | ADDRESS | SALARY |
---|---|---|---|---|
1 | Ramesh | 32 | Ahmedabad | 2000.00 |
2 | Khilan | 25 | Delhi | 1500.00 |
3 | Chaitali | 25 | Mumbai | 6500.00 |
4 | Hardik | 27 | Bhopal | 8500.00 |
The following query adds two new rows into the CUSTOMERS table −
INSERT INTO CUSTOMERS (ID, NAME, AGE, ADDRESS, SALARY) VALUES (5, "Komal", 22, "Hyderabad", 4500.00), (4, "Kaushik", 23, "Kota", 2000.00) ON DUPLICATE KEY UPDATE NAME = VALUES(NAME), AGE = VALUES(AGE), ADDRESS = VALUES(ADDRESS), SALARY = VALUES(SALARY);
Output
As we can see in the output, there are two new rows (ID 5, and 4) and one updated row (ID 4) where it conflicated with an existing row (there is already a row with an ID of "4").
Query OK, 3 rows affected, 4 warnings (0.01 sec) Records: 2 Duplicates: 1 Warnings: 4
Verification
Execute the following query to verify whether the records have been inserted into the CUSTOMERS table.
SELECT * FROM CUSTOMERS;
If we look at the "CUSTOMERS" table below, we can see that the two new rows added as expected and the values of the conflicted rows have been updated with the new information.
ID | NAME | AGE | ADDRESS | SALARY |
---|---|---|---|---|
1 | Ramesh | 32 | Ahmedabad | 2000.00 |
2 | Khilan | 25 | Delhi | 1500.00 |
3 | Chaitali | 25 | Mumbai | 6500.00 |
4 | Kaushik | 23 | Kota | 2000.00 |
5 | Komal | 22 | Hyderabad | 4500.00 |
Client Program
In addition to perform the Insert On Duplicate key Update Query in MySQL table using MySQL query, we can also perform the same operation on a table using a client program.
Syntax
Following are the syntaxes of this operation in various programming languages −
To update a duplicate row with new one in MySQL table through a PHP program, we use the DUPLICATE KEY UPDATE along with INSERT statement using the mysqli function query() as −
$sql = "INSERT INTO my_table (column1, column2, ...) VALUES (value1, value2), (value3, value4), ... ON DUPLICATE KEY UPDATE column1 = value1, column2 = value2, ..."; $mysqli->query($sql);
To update the duplicate row with new one in MySQL table through a Node.js program, we use the DUPLICATE KEY UPDATE along with INSERT statement using the query() function of the mysql2 library as −
sql = "INSERT INTO my_table (column1, column2, ...) VALUES (value1, value2), (value3, value4), ... ON DUPLICATE KEY UPDATE column1 = value1, column2 = value2, ..."; con.query(sql);
To update the duplicate row with new one in MySQL table through a Java program, we use the DUPLICATE KEY UPDATE along with INSERT statement using the JDBC function executeUpdate() as −
String sql = "INSERT INTO my_table (column1, column2, ...) VALUES (value1, value2), (value3, value4), ... ON DUPLICATE KEY UPDATE column1 = value1, column2 = value2, ..."; statement.executeUpdate(sql);
To update the duplicate row with new one in MySQL tablet through a Python program, we use the DUPLICATE KEY UPDATE along with INSERT statement using the execute() function of the MySQL Connector/Python as −
insert_on_duplicate_key_update_query = "INSERT INTO table_name (column1, column2, ...) VALUES (value1, value2, ...) ON DUPLICATE KEY UPDATE column1 = VALUES(column1), column2 = VALUES(column2), ..." cursorObj.execute(insert_on_duplicate_key_update_query)
Example
Following are the programs −
$dbhost = 'localhost'; $dbuser = 'root'; $dbpass = 'password'; $dbname = 'TUTORIALS'; $mysqli = new mysqli($dbhost, $dbuser, $dbpass, $dbname); if($mysqli->connect_errno ) { printf("Connect failed: %s
", $mysqli->connect_error); exit(); } //printf('Connected successfully.
'); $q = "SELECT * FROM tutorials_tbl"; if($res = $mysqli->query($q)){ printf("Select query executed successfully..!\n"); printf("The table 'tutorials_tbl' records before insert into duplicate key update query executed: \n"); while($r = mysqli_fetch_array($res)){ print_r ($r); } } $sql = "INSERT INTO tutorials_tbl(tutorial_id, tutorial_title, tutorial_author, submission_date) VALUES(2, 'PHP Tut', 'unknown2', '2023-08-12') ON DUPLICATE KEY UPDATE tutorial_author = 'New Author'"; if($result = $mysqli->query($sql)){ printf("Insert on Duplicate Key Update query executed successfully..! \n"); } $q = "SELECT * FROM tutorials_tbl"; if($res = $mysqli->query($q)){ printf("Select query executed successfully..!\n"); printf("The table 'tutorials_tbl' records after insert into duplicate key update query executed: \n"); while($r = mysqli_fetch_array($res)){ print_r ($r); } } if($mysqli->error){ printf("Failed..!" , $mysqli->error); } $mysqli->close();
Output
The output obtained is as follows −
Select query executed successfully..! The table 'tutorials_tbl' records before insert into duplicate key update query executed: Array ( [0] => 1 [tutorial_id] => 1 [1] => Java Tutorial [tutorial_title] => Java Tutorial [2] => new_author [tutorial_author] => new_author [3] => [submission_date] => ) Array ( [0] => 2 [tutorial_id] => 2 [1] => PHP Tut [tutorial_title] => PHP Tut [2] => unknown2 [tutorial_author] => unknown2 [3] => 2023-08-12 [submission_date] => 2023-08-12 ) Insert on Duplicate Key Update query executed successfully..! Select query executed successfully..! The table 'tutorials_tbl' records after insert into duplicate key update query executed: Array ( [0] => 1 [tutorial_id] => 1 [1] => Java Tutorial [tutorial_title] => Java Tutorial [2] => new_author [tutorial_author] => new_author [3] => [submission_date] => ) Array ( [0] => 2 [tutorial_id] => 2 [1] => PHP Tut [tutorial_title] => PHP Tut [2] => New Author [tutorial_author] => New Author [3] => 2023-08-12 [submission_date] => 2023-08-12 )
var mysql = require('mysql2'); var con = mysql.createConnection({ host: "localhost", user: "root", password: "Nr5a0204@123" }); //Connecting to MySQL con.connect(function (err) { if (err) throw err; console.log("Connected!"); console.log("----------------------------------------"); //Selecting a Database sql = "USE TUTORIALS" con.query(sql); //Creating Table sql = "CREATE TABLE Actors (ID int auto_increment,NAME varchar(20) NOT NULL,LATEST_FILM varchar(20),Primary Key (ID));" con.query(sql); sql= "INSERT INTO Actors (NAME, LATEST_FILM)VALUES ('Prabhas', 'Salaar'),('Ram Charan', 'Game changer'),('Allu Arjun', 'Pushpa2');" con.query(sql, function (err, result) { if (err) throw err; console.log(result); console.log("----------------------------------------"); }); sql = "SELECT * FROM Actors;" con.query(sql, function (err, result) { if (err) throw err; console.log(result); console.log("----------------------------------------"); }); sql = "INSERT INTO Actors (ID, NAME) VALUES (3, 'Fahad') ON DUPLICATE KEY UPDATE NAME = 'Fahad';" con.query(sql, function (err, result) { if (err) throw err; console.log(result); console.log("----------------------------------------"); }); sql = "SELECT * FROM Actors;" con.query(sql, function (err, result) { if (err) throw err; console.log(result); }); });
Output
The output produced is as follows −
Connected! ---------------------------------------- ResultSetHeader { fieldCount: 0, affectedRows: 3, insertId: 1, info: 'Records: 3 Duplicates: 0 Warnings: 0', serverStatus: 2, warningStatus: 0, changedRows: 0 } ---------------------------------------- [ { ID: 1, NAME: 'Prabhas', LATEST_FILM: 'Salaar' }, { ID: 2, NAME: 'Ram Charan', LATEST_FILM: 'Game changer' }, { ID: 3, NAME: 'Allu Arjun', LATEST_FILM: 'Pushpa2' } ] ---------------------------------------- ResultSetHeader { fieldCount: 0, affectedRows: 2, insertId: 3, info: '', serverStatus: 2, warningStatus: 0, changedRows: 0 } ---------------------------------------- [ { ID: 1, NAME: 'Prabhas', LATEST_FILM: 'Salaar' }, { ID: 2, NAME: 'Ram Charan', LATEST_FILM: 'Game changer' }, { ID: 3, NAME: 'Fahad', LATEST_FILM: 'Pushpa2' } ]
public class InsertOnDuplicate { public static void main(String[] args) { String url = "jdbc:mysql://localhost:3306/TUTORIALS"; String user = "root"; String password = "password"; ResultSet rs; try { Class.forName("com.mysql.cj.jdbc.Driver"); Connection con = DriverManager.getConnection(url, user, password); Statement st = con.createStatement(); //System.out.println("Database connected successfully...!"); String sql = "SELECT * FROM Actors"; rs = st.executeQuery(sql); System.out.println("Table records before insert on duplicate key update: "); while(rs.next()) { String id = rs.getString("ID"); String name = rs.getString("NAME"); String latest_film = rs.getString("LATEST_FILM"); System.out.println("Id: " + id + ", Name: " + name + ", Latest_film: " + latest_film); } //let use insert on duplicate update String sql1 = "INSERT INTO Actors (ID, NAME) VALUES (3, \"Ravi\") ON DUPLICATE KEY UPDATE NAME = \"Ravi\""; st.executeUpdate(sql1); System.out.println("Query insert on duplicate key update executed successfully....!"); String sql2 = "SELECT * FROM Actors"; rs = st.executeQuery(sql2); System.out.println("Table records after insert on duplicate update: "); while(rs.next()) { String id = rs.getString("ID"); String name = rs.getString("NAME"); String latest_film = rs.getString("LATEST_FILM"); System.out.println("Id: " + id + ", Name: " + name + ", Latest_film: " + latest_film); } }catch(Exception e) { e.printStackTrace(); } } }
Output
The output obtained is as shown below −
Table records before insert on duplicate key update: Id: 1, Name: Prabhas, Latest_film: Salaar Id: 2, Name: Ram Charan, Latest_film: Game changer Id: 3, Name: Allu Arjun, Latest_film: Pushpa2 Query insert on duplicate key update executed successfully....! Table records after insert on duplicate update: Id: 1, Name: Prabhas, Latest_film: Salaar Id: 2, Name: Ram Charan, Latest_film: Game changer Id: 3, Name: Ravi, Latest_film: Pushpa2
import mysql.connector #establishing the connection connection = mysql.connector.connect( host='localhost', user='root', password='password', database='tut' ) cursorObj = connection.cursor() insert_on_duplicate_key_update = "INSERT INTO tutorials_tbl (tutorial_id, tutorial_title, tutorial_author, submission_date) VALUES (7, 'New Tutorial', 'John Doe', '2023-07-25') ON DUPLICATE KEY UPDATE tutorial_title='Updated Tutorial', tutorial_author='Jane Smith', submission_date='2023-07-28'" cursorObj.execute(insert_on_duplicate_key_update) connection.commit() print("INSERT on duplicate key update query executed successfully.") cursorObj.close() connection.close()
Output
Following is the output of the above code −
INSERT on duplicate key update query executed successfully.