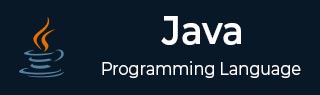
Java Tutorial
- Java - Home
- Java - Overview
- Java - History
- Java - Features
- Java vs C++
- Java Virtual Machine(JVM)
- Java - JDK vs JRE vs JVM
- Java - Hello World Program
- Java - Environment Setup
- Java - Basic Syntax
- Java - Variable Types
- Java - Data Types
- Java - Type Casting
- Java - Unicode System
- Java - Basic Operators
- Java - Comments
- Java - User Input
Java Control Statements
- Java - Loop Control
- Java - Decision Making
- Java - If-else
- Java - Switch
- Java - For Loops
- Java - For-Each Loops
- Java - While Loops
- Java - do-while Loops
- Java - Break
- Java - Continue
Object Oriented Programming
- Java - OOPs Concepts
- Java - Object & Classes
- Java - Class Attributes
- Java - Class Methods
- Java - Methods
- Java - Variables Scope
- Java - Constructors
- Java - Access Modifiers
- Java - Inheritance
- Java - Aggregation
- Java - Polymorphism
- Java - Overriding
- Java - Method Overloading
- Java - Dynamic Binding
- Java - Static Binding
- Java - Instance Initializer Block
- Java - Abstraction
- Java - Encapsulation
- Java - Interfaces
- Java - Packages
- Java - Inner Classes
- Java - Static Class
- Java - Anonymous Class
- Java - Singleton Class
- Java - Wrapper Classes
- Java - Enums
- Java - Enum Constructor
- Java - Enum Strings
Java Built-in Classes
Java File Handling
- Java - Files
- Java - Create a File
- Java - Write to File
- Java - Read Files
- Java - Delete Files
- Java - Directories
- Java - I/O Streams
Java Error & Exceptions
- Java - Exceptions
- Java - try-catch Block
- Java - try-with-resources
- Java - Multi-catch Block
- Java - Nested try Block
- Java - Finally Block
- Java - throw Exception
- Java - Exception Propagation
- Java - Built-in Exceptions
- Java - Custom Exception
Java Multithreading
- Java - Multithreading
- Java - Thread Life Cycle
- Java - Creating a Thread
- Java - Starting a Thread
- Java - Joining Threads
- Java - Naming Thread
- Java - Thread Scheduler
- Java - Thread Pools
- Java - Main Thread
- Java - Thread Priority
- Java - Daemon Threads
- Java - Thread Group
- Java - Shutdown Hook
Java Synchronization
- Java - Synchronization
- Java - Block Synchronization
- Java - Static Synchronization
- Java - Inter-thread Communication
- Java - Thread Deadlock
- Java - Interrupting a Thread
- Java - Thread Control
- Java - Reentrant Monitor
Java Networking
- Java - Networking
- Java - Socket Programming
- Java - URL Processing
- Java - URL Class
- Java - URLConnection Class
- Java - HttpURLConnection Class
- Java - Socket Class
- Java - Generics
Java Collections
Java List Interface
Java Queue Interface
Java Map Interface
- Java - Map Interface
- Java - HashMap
- Java - LinkedHashMap
- Java - WeakHashMap
- Java - EnumMap
- Java - SortedMap Interface
- Java - TreeMap
- Java - The IdentityHashMap Class
Java Set Interface
- Java - Set Interface
- Java - HashSet
- Java - EnumSet
- Java - LinkedHashSet
- Java - SortedSet Interface
- Java - TreeSet
Java Data Structures
- Java - Data Structures
- Java - Enumeration
- Java - BitSet Class
- Java - Dictionary
- Java - Hashtable
- Java - Properties
Java Collections Algorithms
Advanced Java
- Java - Command-Line Arguments
- Java - Lambda Expressions
- Java - Sending Email
- Java - Applet Basics
- Java - Javadoc Comments
- Java - Autoboxing and Unboxing
- Java - File Mismatch Method
- Java - REPL (JShell)
- Java - Multi-Release Jar Files
- Java - Private Interface Methods
- Java - Inner Class Diamond Operator
- Java - Multiresolution Image API
- Java - Collection Factory Methods
- Java - Module System
- Java - Nashorn JavaScript
- Java - Optional Class
- Java - Method References
- Java - Functional Interfaces
- Java - Default Methods
- Java - Base64 Encode Decode
- Java - Switch Expressions
- Java - Teeing Collectors
- Java - Microbenchmark
- Java - Text Blocks
- Java - Null Pointer Exception
- Java - Packaging Tools
- Java - Sealed Classes
- Java - Record Classes
- Java - Hidden Classes
- Java - Compact Number Formatting
Java Miscellaneous
- Java - Recursion
- Java - Regular Expressions
- Java - Serialization
- Java - Strings
- Java - Process API Improvements
- Java - Stream API Improvements
- Java - Enhanced @Deprecated Annotation
- Java - CompletableFuture API Improvements
- Java - Array Methods
- Java - Streams
- Java - Datetime Api
- Java 8 - New Features
- Java 9 - New Features
Java APIs & Frameworks
Java Useful Resources
Java - Type Casting
Java Type Casting (Type Conversion)
Type casting is a technique that is used either by the compiler or a programmer to convert one data type to another. For example, converting int to double, double to int, short to int, etc. Type typing is also known as Type conversion.
There are two types of cast typing allowed in Java programming:
- Widening type casting
- Narrowing type casting
Widening Type Casting
Widening Type Casting is also known as implicit type casting in which a smaller type is converted into a larger type, it is done by the compiler automatically.
Here is the hierarchy of widening type casting in Java:
byte > short > char > int > long > float > double
The compiler plays a role in the type conversion but not the programmers. It changes the type of the variables at the compile time. Also, type conversion occurs from the small data type to large data type only.
Example 1: Widening Type Casting
In the example below, we demonstrated that we can get an error when the compiler tries to convert a large data type to a small data type. Here, we created the num1 integer and num2 double variable. The sum of num1 and num2 will be double, and when we try to store it to the sum of the int type, the compiler gives an error.
package com.tutorialspoint; public class Tester { // Main driver method public static void main(String[] args) { // Define int variables int num1 = 5004; double num2 = 2.5; int sum = num1 + num2; // show output System.out.println("The sum of " + num1 + " and " + num2 + " is " + sum); } }
Compile and run Tester. This will produce the following result −
Output
Exception in thread "main" java.lang.Error: Unresolved compilation problem: Type mismatch: cannot convert from double to int at com.tutorialspoint.Tester.main(Tester.java:9)
Example 2: Widening Type Casting
In the example below, we created the num1 and num2 variables as same in the first example. We store the sum of both numbers in the sum variable of double type. In the output, we can observe the sum of double type.
package com.tutorialspoint; public class Tester { // Main driver method public static void main(String[] args) { // Define int variables int num1 = 5004; double num2 = 2.5; double sum = num1 + num2; // show output System.out.println("The sum of " + num1 + " and " + num2 + " is " + sum); } }
Compile and run Tester. This will produce the following result −
Output
The sum of 5004 and 2.5 is 5006.5
Narrowing Type Casting
Narrowing type casting is also known as explicit type casting or explicit type conversion which is done by the programmer manually. In the narrowing type casting a larger type can be converted into a smaller type.
When a programmer changes the variable type while writing the code. We can use the cast operator to change the type of the variable. For example, double to int or int to double.
Syntax
Below is the syntax for narrowing type casting i.e., to manually type conversion:
double doubleNum = (double) num;
The above code statement will convert the variable to double type.
Example: Narrowing Type Casting
In the example below, we define the num variable of integer type and initialize it with the value. Also, we define the doubleNum variable of double type and store the num variable's value after converting it to the double.
Next, We created the 'convertedInt' integer type variable and stored the double value after type casting to int. In the output, we can observe the value of the double and int variables.
package com.tutorialspoint; public class Tester { // Main driver method public static void main(String[] args) { // Define int variable int num = 5004; // Type casting int to double double doubleNum = (double) num; // show output System.out.println("The value of " + num + " after converting to the double is " + doubleNum); // Type casting double to int int convertedInt = (int) doubleNum; // show output System.out.println("The value of " + doubleNum + " after converting to the int again is " + convertedInt); } }
Compile and run Tester. This will produce the following result −
Output
The value of 5004 after converting to the double is 5004.0 The value of 5004.0 after converting to the int again is 5004
To Continue Learning Please Login