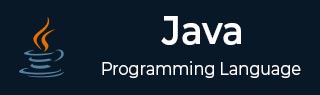
Java Tutorial
- Java - Home
- Java - Overview
- Java - History
- Java - Features
- Java vs C++
- Java Virtual Machine(JVM)
- Java - JDK vs JRE vs JVM
- Java - Hello World Program
- Java - Environment Setup
- Java - Basic Syntax
- Java - Variable Types
- Java - Data Types
- Java - Type Casting
- Java - Unicode System
- Java - Basic Operators
- Java - Comments
- Java - User Input
Java Control Statements
- Java - Loop Control
- Java - Decision Making
- Java - If-else
- Java - Switch
- Java - For Loops
- Java - For-Each Loops
- Java - While Loops
- Java - do-while Loops
- Java - Break
- Java - Continue
Object Oriented Programming
- Java - OOPs Concepts
- Java - Object & Classes
- Java - Class Attributes
- Java - Class Methods
- Java - Methods
- Java - Variables Scope
- Java - Constructors
- Java - Access Modifiers
- Java - Inheritance
- Java - Aggregation
- Java - Polymorphism
- Java - Overriding
- Java - Method Overloading
- Java - Dynamic Binding
- Java - Static Binding
- Java - Instance Initializer Block
- Java - Abstraction
- Java - Encapsulation
- Java - Interfaces
- Java - Packages
- Java - Inner Classes
- Java - Static Class
- Java - Anonymous Class
- Java - Singleton Class
- Java - Wrapper Classes
- Java - Enums
- Java - Enum Constructor
- Java - Enum Strings
Java Built-in Classes
Java File Handling
- Java - Files
- Java - Create a File
- Java - Write to File
- Java - Read Files
- Java - Delete Files
- Java - Directories
- Java - I/O Streams
Java Error & Exceptions
- Java - Exceptions
- Java - try-catch Block
- Java - try-with-resources
- Java - Multi-catch Block
- Java - Nested try Block
- Java - Finally Block
- Java - throw Exception
- Java - Exception Propagation
- Java - Built-in Exceptions
- Java - Custom Exception
Java Multithreading
- Java - Multithreading
- Java - Thread Life Cycle
- Java - Creating a Thread
- Java - Starting a Thread
- Java - Joining Threads
- Java - Naming Thread
- Java - Thread Scheduler
- Java - Thread Pools
- Java - Main Thread
- Java - Thread Priority
- Java - Daemon Threads
- Java - Thread Group
- Java - Shutdown Hook
Java Synchronization
- Java - Synchronization
- Java - Block Synchronization
- Java - Static Synchronization
- Java - Inter-thread Communication
- Java - Thread Deadlock
- Java - Interrupting a Thread
- Java - Thread Control
- Java - Reentrant Monitor
Java Networking
- Java - Networking
- Java - Socket Programming
- Java - URL Processing
- Java - URL Class
- Java - URLConnection Class
- Java - HttpURLConnection Class
- Java - Socket Class
- Java - Generics
Java Collections
Java List Interface
Java Queue Interface
Java Map Interface
- Java - Map Interface
- Java - HashMap
- Java - LinkedHashMap
- Java - WeakHashMap
- Java - EnumMap
- Java - SortedMap Interface
- Java - TreeMap
- Java - The IdentityHashMap Class
Java Set Interface
- Java - Set Interface
- Java - HashSet
- Java - EnumSet
- Java - LinkedHashSet
- Java - SortedSet Interface
- Java - TreeSet
Java Data Structures
- Java - Data Structures
- Java - Enumeration
- Java - BitSet Class
- Java - Dictionary
- Java - Hashtable
- Java - Properties
Java Collections Algorithms
Advanced Java
- Java - Command-Line Arguments
- Java - Lambda Expressions
- Java - Sending Email
- Java - Applet Basics
- Java - Javadoc Comments
- Java - Autoboxing and Unboxing
- Java - File Mismatch Method
- Java - REPL (JShell)
- Java - Multi-Release Jar Files
- Java - Private Interface Methods
- Java - Inner Class Diamond Operator
- Java - Multiresolution Image API
- Java - Collection Factory Methods
- Java - Module System
- Java - Nashorn JavaScript
- Java - Optional Class
- Java - Method References
- Java - Functional Interfaces
- Java - Default Methods
- Java - Base64 Encode Decode
- Java - Switch Expressions
- Java - Teeing Collectors
- Java - Microbenchmark
- Java - Text Blocks
- Java - Null Pointer Exception
- Java - Packaging Tools
- Java - Sealed Classes
- Java - Record Classes
- Java - Hidden Classes
- Java - Compact Number Formatting
Java Miscellaneous
- Java - Recursion
- Java - Regular Expressions
- Java - Serialization
- Java - Strings
- Java - Process API Improvements
- Java - Stream API Improvements
- Java - Enhanced @Deprecated Annotation
- Java - CompletableFuture API Improvements
- Java - Array Methods
- Java - Streams
- Java - Datetime Api
- Java 8 - New Features
- Java 9 - New Features
Java APIs & Frameworks
Java Useful Resources
Java - Default Methods in Interfaces
Java Default Methods
Java introduced a new concept of default method implementation in interfaces in Java 8. Prior to Java8, an interface could have only abstract methods. Default method capability was added for backward compatibility so that old interfaces could be used to leverage the lambda expression capability of Java 8.
For example, List or Collection interfaces do not have 'forEach' method declaration. Thus, adding such method will simply break the collection framework implementations. Java 8 introduces default method so that List/Collection interface can have a default implementation of forEach method, and the class implementing these interfaces need not implement the same.
Syntax
The following is the syntax of the default method in interface in Java -
public interface vehicle { default void print() { System.out.println("I am a vehicle!"); } }
Java Default Method Example
package com.tutorialspoint; interface vehicle { // default method must have an implementation default void print() { System.out.println("I am a vehicle!"); } } // implementing class needs not to implement the default method // of an interface. public class Tester implements vehicle { public static void main(String args[]) { Tester tester = new Tester(); // implementing class can access the default method as its own method tester.print(); } }
Let us compile and run the above program, this will produce the following result −
I am a vehicle!
Default Methods in Multiple Inheritance
With default functions in interfaces, there is a possibility that a class is implementing two interfaces with same default methods. The following code explains how this ambiguity can be resolved.
public interface vehicle { default void print() { System.out.println("I am a vehicle!"); } } public interface fourWheeler { default void print() { System.out.println("I am a four wheeler!"); } }
First solution is to create an own method that overrides the default implementation.
public class car implements vehicle, fourWheeler { public void print() { System.out.println("I am a four wheeler car vehicle!"); } }
Example: Overriding default method of interfaces with own implementation
In this example, we've created two interfaces with same default method print(). As Car class is implementing both the interfaces, so it has to override the default method otherwise compiler will complain for duplicate default methods. After overriding the default method with own implementation, we can easily use the print method of the Car class as shown below:
package com.tutorialspoint; interface Vehicle { default void print() { System.out.println("I am a vehicle!"); } } interface FourWheeler { default void print() { System.out.println("I am a four wheeler!"); } } class Car implements Vehicle, FourWheeler { // overriding the default method will resolve the ambiguity public void print() { System.out.println("I am a four wheeler car vehicle!"); } } public class Tester { public static void main(String args[]) { Car car = new Car(); car.print(); } }
Let us compile and run the above program, this will produce the following result −
I am a four wheeler car vehicle!
Second solution is to call the default method of the specified interface using super.
public class car implements vehicle, fourWheeler { public void print() { vehicle.super.print(); } }
Example: Calling default method of interfaces
In this example, we've created two interfaces with same default method print(). As Car class is implementing both the interfaces, so it has to override the default method otherwise compiler will complain for duplicate default methods. After overriding the default method with own implementation, we can easily use the print method of the Car class as shown below:
package com.tutorialspoint; interface Vehicle { default void print() { System.out.println("I am a vehicle!"); } } interface FourWheeler { default void print() { System.out.println("I am a four wheeler!"); } } class Car implements Vehicle, FourWheeler { // use the default method of a interface public void print() { FourWheeler.super.print(); } } public class Tester { public static void main(String args[]) { Car car = new Car(); car.print(); } }
Let us compile and run the above program, this will produce the following result −
I am a four wheeler!
Static Default Methods in Java
An interface can also have static default methods from Java 8 onwards. These static methods acts as helper or utility functions and helps in better encapsulation of code.
public interface vehicle { default void print() { System.out.println("I am a vehicle!"); } static void blowHorn() { System.out.println("Blowing horn!!!"); } }
Example: Calling static default method of interface
In this example, we've created two interfaces with same default method print(). As Car class is implementing both the interfaces, so it has to override the default method. After overriding the default method with calls to interfaces implementation, we've called the static method directly as shown below:
package com.tutorialspoint; interface Vehicle { default void print() { System.out.println("I am a vehicle!"); } static void blowHorn() { System.out.println("Blowing horn!!!"); } } interface FourWheeler { default void print() { System.out.println("I am a four wheeler!"); } } class Car implements Vehicle, FourWheeler { public void print() { // call the Vehicle interface default print method Vehicle.super.print(); FourWheeler.super.print(); // call the Vehicle interface static blowHorn method Vehicle.blowHorn(); System.out.println("I am a car!"); } } public class Tester { public static void main(String args[]) { Vehicle vehicle = new Car(); vehicle.print(); // call the Vehicle interface static blowHorn method Vehicle.blowHorn(); } }
Let us compile and run the above program, this will produce the following result −
I am a vehicle! I am a four wheeler! Blowing horn!!! I am a car! Blowing horn!!!
To Continue Learning Please Login