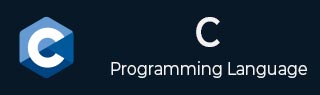
- C Programming Tutorial
- C - Home
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Tokens
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Type Conversion
- C - Booleans
- C - Constants
- C - Literals
- C - Escape sequences
- C - Format Specifiers
- C - Storage Classes
- C - Operators
- C - Arithmetic Operators
- C - Relational Operators
- C - Logical Operators
- C - Bitwise Operators
- C - Assignment Operators
- C - Unary Operators
- C - Increment and Decrement Operators
- C - Ternary Operator
- C - sizeof Operator
- C - Operator Precedence
- C - Misc Operators
- C - Decision Making
- C - if statement
- C - if...else statement
- C - nested if statements
- C - switch statement
- C - nested switch statements
- C - Loops
- C - While loop
- C - For loop
- C - Do...while loop
- C - Nested loop
- C - Infinite loop
- C - Break Statement
- C - Continue Statement
- C - goto Statement
- C - Functions
- C - Main Functions
- C - Function call by Value
- C - Function call by reference
- C - Nested Functions
- C - Variadic Functions
- C - User-Defined Functions
- C - Callback Function
- C - Return Statement
- C - Recursion
- C - Scope Rules
- C - Static Variables
- C - Global Variables
- C - Arrays
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
- C - Pointers
- C - Pointers and Arrays
- C - Applications of Pointers
- C - Pointer Arithmetics
- C - Array of Pointers
- C - Pointer to Pointer
- C - Passing Pointers to Functions
- C - Return Pointer from Functions
- C - Function Pointers
- C - Pointer to an Array
- C - Pointers to Structures
- C - Chain of Pointers
- C - Pointer vs Array
- C - Character Pointers and Functions
- C - NULL Pointer
- C - void Pointer
- C - Dangling Pointers
- C - Dereference Pointer
- C - Near, Far and Huge Pointers
- C - Initialization of Pointer Arrays
- C - Pointers vs. Multi-dimensional Arrays
- C - Strings
- C - Array of Strings
- C - Special Characters
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Self-Referential Structures
- C - Lookup Tables
- C - Dot (.) Operator
- C - Enumeration (or enum)
- C - Nested Structures
- C - Structure Padding and Packing
- C - Anonymous Structure and Union
- C - Unions
- C - Bit Fields
- C - Typedef
- C - Input & Output
- C - File I/O
- C - Preprocessors
- C - Pragmas
- C - Preprocessor Operators
- C - Macros
- C - Header Files
- C - Type Casting
- C - Error Handling
- C - Variable Arguments
- C - Memory Management
- C - Command Line Arguments
- C Programming Resources
- C - Questions & Answers
- C - Quick Guide
- C - Useful Resources
- C - Discussion
void Pointer in C
A void pointer in C is a type of pointer that is not associated with any data type. A void pointer can hold an address of any type and can be typecasted to any type. They are also called general-purpose or generic pointers.
In C programming, the function malloc() and calloc() return "void *" or generic pointers.
Syntax
This is the syntax you would use to declare a void pointer −
void *ptr;
A void pointer cannot work with increment or decrement operators because it doesn’t have a specific type. Pointer arithmetic is not possible with a void pointer due to its concrete size.
Example
The following example shows how you can use a void pointer in a C program −
#include <stdio.h> int main(){ int a = 10; char b = 'x'; // void pointer holds address of int a void *ptr = &a; printf("Address of 'a': %d", &a); printf("\nVoid pointer points to: %d", ptr); // it now points to char b ptr = &b; printf("\nAddress of 'b': %d", &b); printf("\nVoid pointer points to: %d", ptr); }
Output
Run the code and check its output −
Address of 'a': 853377452 Void pointer points to: 853377452 Address of 'b': 853377451 Void pointer points to: 853377451
An Array of void Pointers
We can declare an array of void pointers and store pointers to different data types.
A void pointer is a pointer that can hold the memory address of any data type in C. Hence, an array of void pointers is an array that can store memory addresses, but these addresses can point to variables of different data types.
You can also store pointers to any user-defined data types such as arrays and structures in a void pointer.
Example
In this example program, we have declared an array of void pointers and stored in it the pointers to variables of different types (int, float, and char *) in each of its subscripts.
#include <stdio.h> int main(){ void *arr[3]; int a = 100; float b = 20.5; char *c = "Hello"; arr[0] = &a; arr[1] = &b; arr[2] = &c; printf("Integer: %d\n", *((int *)arr[0])); printf("Float: %f\n", *((float *)arr[1])); printf("String: %s\n", *((char **)arr[2])); return 0; }
Output
When you run this code, it will produce the following output −
Integer: 100 Float: 20.500000 String: Hello
Application of void Pointers
The malloc() function is available as a library function in the header file "stdlib.h". It dynamically allocates a block of memory during the runtime of a program. Normal declaration of variables causes the memory to be allocated at the compile time.
void *malloc(size_t size);
Void pointers are used to implement generic functions. The dynamic allocation functions malloc() and calloc() return "void *" type and this feature allows these functions to be used to allocate memory of any data type.
The most common application of void pointers is in the implementation of data structures such as linked lists, trees, and queues, i.e., dynamic data structures.
To Continue Learning Please Login