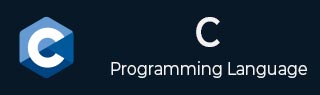
- C Programming Tutorial
- C - Home
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Tokens
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Type Conversion
- C - Booleans
- C - Constants
- C - Literals
- C - Escape sequences
- C - Format Specifiers
- C - Storage Classes
- C - Operators
- C - Arithmetic Operators
- C - Relational Operators
- C - Logical Operators
- C - Bitwise Operators
- C - Assignment Operators
- C - Unary Operators
- C - Increment and Decrement Operators
- C - Ternary Operator
- C - sizeof Operator
- C - Operator Precedence
- C - Misc Operators
- C - Decision Making
- C - if statement
- C - if...else statement
- C - nested if statements
- C - switch statement
- C - nested switch statements
- C - Loops
- C - While loop
- C - For loop
- C - Do...while loop
- C - Nested loop
- C - Infinite loop
- C - Break Statement
- C - Continue Statement
- C - goto Statement
- C - Functions
- C - Main Functions
- C - Function call by Value
- C - Function call by reference
- C - Nested Functions
- C - Variadic Functions
- C - User-Defined Functions
- C - Callback Function
- C - Return Statement
- C - Recursion
- C - Scope Rules
- C - Static Variables
- C - Global Variables
- C - Arrays
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
- C - Pointers
- C - Pointers and Arrays
- C - Applications of Pointers
- C - Pointer Arithmetics
- C - Array of Pointers
- C - Pointer to Pointer
- C - Passing Pointers to Functions
- C - Return Pointer from Functions
- C - Function Pointers
- C - Pointer to an Array
- C - Pointers to Structures
- C - Chain of Pointers
- C - Pointer vs Array
- C - Character Pointers and Functions
- C - NULL Pointer
- C - void Pointer
- C - Dangling Pointers
- C - Dereference Pointer
- C - Near, Far and Huge Pointers
- C - Initialization of Pointer Arrays
- C - Pointers vs. Multi-dimensional Arrays
- C - Strings
- C - Array of Strings
- C - Special Characters
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Self-Referential Structures
- C - Lookup Tables
- C - Dot (.) Operator
- C - Enumeration (or enum)
- C - Nested Structures
- C - Structure Padding and Packing
- C - Anonymous Structure and Union
- C - Unions
- C - Bit Fields
- C - Typedef
- C - Input & Output
- C - File I/O
- C - Preprocessors
- C - Pragmas
- C - Preprocessor Operators
- C - Macros
- C - Header Files
- C - Type Casting
- C - Error Handling
- C - Variable Arguments
- C - Memory Management
- C - Command Line Arguments
- C Programming Resources
- C - Questions & Answers
- C - Quick Guide
- C - Useful Resources
- C - Discussion
Near Far and Huge Pointers in C
Concepts like near pointers, far pointers, and huge pointers were used in the C programming language to handle segmented memory models. However, these concepts are no longer relevant in modern computing environments with improved CPU architecture.
The idea of near, far, and huge pointers was implemented in 16-bit Intel architectures, in the days of MS DOS operating system.
Near Pointer
The "near" keyword in C is used to declare a pointer that can only access memory within the current data segment. A "near pointer" on a 16-bit machine is a pointer that can store only 16-bit addresses.
A near pointer can only access data of a small size of about 64 kb in a given period, which is its main disadvantage. The size of a near pointer is 2 bytes.
Syntax
<data type> near <pointer definition> <data type> near <function definition>
The following statement declares a near pointer for the variable "ptr" −
char near *ptr;
Example
Take a look at the following example −
#include <stdio.h> int main(){ // declaring a near pointer int near *ptr; // size of the near pointer printf("Size of Near Pointer: %d bytes", sizeof(ptr)); return 0; }
Output
It will produce the following output −
Size of Near Pointer: 2 bytes
Far Pointer
A "far pointer" is a 32-bit pointer that can access information that is outside the computer memory in a given segment. To use this pointer, one must allocate the "sector register" to store data address in the segment and also another sector register must be stored within the most recent sector.
A far pointer stores both the offset and segment addresses to which the pointer is differencing. When the pointer is incremented or decremented, only the offset part is changing. The size of the far pointer is 4 bytes.
Syntax
<data type> far <pointer definition> <data type> far <function definition>
The following statements declares a far pointer for the variable "ptr" −
char far *s;
Example
Take a look at the following example −
#include <stdio.h> int main(){ int number=50; int far *p; p = &number; printf("Size of far pointer: %d bytes", sizeof(number)); return 0; }
Output
It will produce the following output −
Size of far pointer: 4 bytes
Huge Pointer
A "huge pointer" has the same size of 32-bit as that of a "far pointer". A huge pointer can also access bits that are located outside the sector.
A far pointer is fixed and hence that part of the sector in which they are located cannot be modified in any way; however huge pointers can be modified.
In a huge pointer, both the offset and segment address is changed. That is why we can jump from one segment to another using a huge Pointer. As they compare the absolute addresses, you can perform the relational operation on it. The size of a huge pointer is 4 bytes.
Example
Take a look at the following example −
#include <stdio.h> int main(){ int huge* ptr; printf("Size of the Huge Pointer: %d bytes", sizeof(ptr)); return 0; }
Output
It will produce the following output −
Size of Huge Pointer: 4 bytes
Points to Note about Near, Far and Huge Pointers
A near pointer can only store till the first 64kB addresses, while a far pointer can store the address of any memory location in the RAM. A huge pointer can move between multiple memory segments.
A near pointer can only store addresses in a single register. On the other hand, a far pointer uses two registers to store segment and offset addresses. The size of a near pointer is 2 bytes, while the size of far and huge pointers is 4 bytes.
Two far pointer values can point to the same location, while in the case of huge pointers, it is not possible.
Conclusion
The near, far, and huge pointers were used to manage memory access based on segment registers in segmented memory architectures. Modern systems use flat memory models where memory is addressed as a single contiguous space. Modern C compilers provide better memory management techniques that don't rely on segmentation concepts.
To Continue Learning Please Login