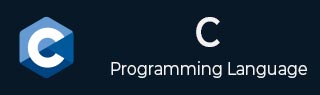
- C Programming Tutorial
- C - Home
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Tokens
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Type Conversion
- C - Booleans
- C - Constants
- C - Literals
- C - Escape sequences
- C - Format Specifiers
- C - Storage Classes
- C - Operators
- C - Arithmetic Operators
- C - Relational Operators
- C - Logical Operators
- C - Bitwise Operators
- C - Assignment Operators
- C - Unary Operators
- C - Increment and Decrement Operators
- C - Ternary Operator
- C - sizeof Operator
- C - Operator Precedence
- C - Misc Operators
- C - Decision Making
- C - if statement
- C - if...else statement
- C - nested if statements
- C - switch statement
- C - nested switch statements
- C - Loops
- C - While loop
- C - For loop
- C - Do...while loop
- C - Nested loop
- C - Infinite loop
- C - Break Statement
- C - Continue Statement
- C - goto Statement
- C - Functions
- C - Main Functions
- C - Function call by Value
- C - Function call by reference
- C - Nested Functions
- C - Variadic Functions
- C - User-Defined Functions
- C - Callback Function
- C - Return Statement
- C - Recursion
- C - Scope Rules
- C - Static Variables
- C - Global Variables
- C - Arrays
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
- C - Pointers
- C - Pointers and Arrays
- C - Applications of Pointers
- C - Pointer Arithmetics
- C - Array of Pointers
- C - Pointer to Pointer
- C - Passing Pointers to Functions
- C - Return Pointer from Functions
- C - Function Pointers
- C - Pointer to an Array
- C - Pointers to Structures
- C - Chain of Pointers
- C - Pointer vs Array
- C - Character Pointers and Functions
- C - NULL Pointer
- C - void Pointer
- C - Dangling Pointers
- C - Dereference Pointer
- C - Near, Far and Huge Pointers
- C - Initialization of Pointer Arrays
- C - Pointers vs. Multi-dimensional Arrays
- C - Strings
- C - Array of Strings
- C - Special Characters
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Self-Referential Structures
- C - Lookup Tables
- C - Dot (.) Operator
- C - Enumeration (or enum)
- C - Nested Structures
- C - Structure Padding and Packing
- C - Anonymous Structure and Union
- C - Unions
- C - Bit Fields
- C - Typedef
- C - Input & Output
- C - File I/O
- C - Preprocessors
- C - Pragmas
- C - Preprocessor Operators
- C - Macros
- C - Header Files
- C - Type Casting
- C - Error Handling
- C - Variable Arguments
- C - Memory Management
- C - Command Line Arguments
- C Programming Resources
- C - Questions & Answers
- C - Quick Guide
- C - Useful Resources
- C - Discussion
Array of Pointers in C
What is an Array of Pointers?
Just like an integer array holds a collection of integer variables, an array of pointers would hold variables of pointer type. It means each variable in an array of pointers is a pointer that points to another address.
The name of an array can be used as a pointer because it holds the address to the first element of the array. If we store the address of an array in another pointer, then it is possible to manipulate the array using pointer arithmetic.
Create an Array of Pointers
To create an array of pointers in C language, you need to declare an array of pointers in the same way as a pointer declaration. Use the data type then an asterisk sign followed by an identifier (array of pointers variable name) with a subscript ([]) containing the size of the array.
In an array of pointers, each element contains the pointer to a specific type.
Example of Creating an Array of Pointers
The following example demonstrates how you can create and use an array of pointers. Here, we are declaring three integer variables and to access and use them, we are creating an array of pointers. With the help of an array of pointers, we are printing the values of the variables.
#include <stdio.h> int main() { // Declaring integers int var1 = 1; int var2 = 2; int var3 = 3; // Declaring an array of pointers to integers int *ptr[3]; // Initializing each element of // array of pointers with the addresses of // integer variables ptr[0] = &var1; ptr[1] = &var2; ptr[2] = &var3; // Accessing values for (int i = 0; i < 3; i++) { printf("Value at ptr[%d] = %d\n", i, *ptr[i]); } return 0; }
Output
When the above code is compiled and executed, it produces the following result −
Value of var[0] = 10 Value of var[1] = 100 Value of var[2] = 200
There may be a situation when we want to maintain an array that can store pointers to an "int" or "char" or any other data type available.
An Array of Pointers to Integers
Here is the declaration of an array of pointers to an integer −
int *ptr[MAX];
It declares ptr as an array of MAX integer pointers. Thus, each element in ptr holds a pointer to an int value.
Example
The following example uses three integers, which are stored in an array of pointers, as follows −
#include <stdio.h> const int MAX = 3; int main(){ int var[] = {10, 100, 200}; int i, *ptr[MAX]; for(i = 0; i < MAX; i++){ ptr[i] = &var[i]; /* assign the address of integer. */ } for (i = 0; i < MAX; i++){ printf("Value of var[%d] = %d\n", i, *ptr[i]); } return 0; }
Output
When the above code is compiled and executed, it produces the following result −
Value of var[0] = 10 Value of var[1] = 100 Value of var[2] = 200
An Array of Pointers to Characters
You can also use an array of pointers to character to store a list of strings as follows −
#include <stdio.h> const int MAX = 4; int main(){ char *names[] = { "Zara Ali", "Hina Ali", "Nuha Ali", "Sara Ali" }; int i = 0; for(i = 0; i < MAX; i++){ printf("Value of names[%d] = %s\n", i, names[i]); } return 0; }
Output
When the above code is compiled and executed, it produces the following result −
Value of names[0] = Zara Ali Value of names[1] = Hina Ali Value of names[2] = Nuha Ali Value of names[3] = Sara Ali
An Array of Pointers to Structures
When you have a list of structures and want to manage it using a pointer. You can declare an array of structures to access and manipulate the list of structures.
Example
The below example demonstrates the use of an array of pointers to structures.
#include <stdio.h> #include <stdlib.h> #include <string.h> // Declaring a structure typedef struct { char title[50]; float price; } Book; const int MAX = 3; int main() { Book *book[MAX]; // Initialize each book (pointer) for (int i = 0; i < MAX; i++) { book[i] = malloc(sizeof(Book)); snprintf(book[i]->title, 50, "Book %d", i + 1); book[i]->price = 100 + i; } // Print details of each book for (int i = 0; i < MAX; i++) { printf("Title: %s, Price: %.2f\n", book[i]->title, book[i]->price); } // Free allocated memory for (int i = 0; i < MAX; i++) { free(book[i]); } return 0; }
Output
When the above code is compiled and executed, it produces the following result −
Title: Book 1, Price: 100.00 Title: Book 2, Price: 101.00 Title: Book 3, Price: 102.00
To Continue Learning Please Login