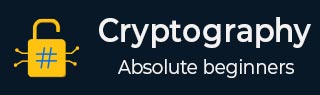
- Cryptography Tutorial
- Cryptography - Home
- Cryptography - Origin
- Cryptography - History
- Cryptography - Principles
- Cryptography - Applications
- Cryptography - Benefits & Drawbacks
- Cryptography - Modern Age
- Cryptography - Traditional Ciphers
- Cryptography - Need for Encryption
- Cryptography - Double Strength Encryption
- Cryptosystems
- Cryptosystems
- Cryptosystems - Components
- Attacks On Cryptosystem
- Cryptosystems - Rainbow table attack
- Cryptosystems - Dictionary attack
- Cryptosystems - Brute force attack
- Cryptosystems - Cryptanalysis Techniques
- Types of Cryptography
- Cryptosystems - Types
- Public Key Encryption
- Modern Symmetric Key Encryption
- Cryptography Hash functions
- Key Management
- Cryptosystems - Key Generation
- Cryptosystems - Key Storage
- Cryptosystems - Key Distribution
- Cryptosystems - Key Revocation
- Block Ciphers
- Cryptosystems - Stream Cipher
- Cryptography - Block Cipher
- Cryptography - Feistel Block Cipher
- Block Cipher Modes of Operation
- Block Cipher Modes of Operation
- Electronic Code Book (ECB) Mode
- Cipher Block Chaining (CBC) Mode
- Cipher Feedback (CFB) Mode
- Output Feedback (OFB) Mode
- Counter (CTR) Mode
- Classic Ciphers
- Cryptography - Reverse Cipher
- Cryptography - Caesar Cipher
- Cryptography - ROT13 Algorithm
- Cryptography - Transposition Cipher
- Cryptography - Encryption Transposition Cipher
- Cryptography - Decryption Transposition Cipher
- Cryptography - Multiplicative Cipher
- Cryptography - Affine Ciphers
- Cryptography - Simple Substitution Cipher
- Cryptography - Encryption of Simple Substitution Cipher
- Cryptography - Decryption of Simple Substitution Cipher
- Cryptography - Vigenere Cipher
- Cryptography - Implementing Vigenere Cipher
- Modern Ciphers
- Base64 Encoding & Decoding
- Cryptography - XOR Encryption
- Substitution techniques
- Cryptography - MonoAlphabetic Cipher
- Cryptography - Hacking Monoalphabetic Cipher
- Cryptography - Polyalphabetic Cipher
- Cryptography - Playfair Cipher
- Cryptography - Hill Cipher
- Polyalphabetic Ciphers
- Cryptography - One-Time Pad Cipher
- Implementation of One Time Pad Cipher
- Cryptography - Transposition Techniques
- Cryptography - Rail Fence Cipher
- Cryptography - Columnar Transposition
- Cryptography - Steganography
- Symmetric Algorithms
- Cryptography - Data Encryption
- Cryptography - Encryption Algorithms
- Cryptography - Data Encryption Standard
- Cryptography - Triple DES
- Cryptography - Double DES
- Advanced Encryption Standard
- Cryptography - AES Structure
- Cryptography - AES Transformation Function
- Cryptography - Substitute Bytes Transformation
- Cryptography - ShiftRows Transformation
- Cryptography - MixColumns Transformation
- Cryptography - AddRoundKey Transformation
- Cryptography - AES Key Expansion Algorithm
- Cryptography - Blowfish Algorithm
- Cryptography - SHA Algorithm
- Cryptography - RC4 Algorithm
- Cryptography - Camellia Encryption Algorithm
- Cryptography - ChaCha20 Encryption Algorithm
- Cryptography - CAST5 Encryption Algorithm
- Cryptography - SEED Encryption Algorithm
- Cryptography - SM4 Encryption Algorithm
- IDEA - International Data Encryption Algorithm
- Public Key (Asymmetric) Cryptography Algorithms
- Cryptography - RSA Algorithm
- Cryptography - RSA Encryption
- Cryptography - RSA Decryption
- Cryptography - Creating RSA Keys
- Cryptography - Hacking RSA Cipher
- Cryptography - ECDSA Algorithm
- Cryptography - DSA Algorithm
- Cryptography - Diffie-Hellman Algorithm
- Data Integrity in Cryptography
- Data Integrity in Cryptography
- Message Authentication
- Cryptography Digital signatures
- Public Key Infrastructure
- Cryptography Useful Resources
- Cryptography - Quick Guide
- Cryptography - Discussion
Cryptography - Transposition Cipher
Now let us discuss the transposition cipher algorithm. The ciphers we used up to this point have been substitution ciphers, in which characters from the plaintext were changed to other characters, numbers, or symbols. The transposition cipher is an additional type of cipher. Transposition ciphers reorder the letters in the plaintext message while still using them.
A transposition cipher should be simple to identify since the letter frequencies should resemble those of English, with high frequencies for the letters a, e, i, n, o, r, s, and t. However, a transposition cipher may be challenging to cryptanalyze. Anagramming, or rearranging the ciphertext letters to "make sense," is the key strategy. The pattern of rearrangement holds the secret of the cipher.
For example the word LAUGH has five different letters. Five different letters can be arranged in 5! 120= ways, and exactly one of those arrangements should result in a word. A brute force attack would try different letter combinations until the word had been identified; at most 120 attempts will be required. Utilising patterns of language to connect word fragments and arrange them to build a word is a more effective strategy.
In this chapter, we will see different types of transposition cipher algorithms, their working principles, and their implementation in different ways.
How does Transposition Cipher work?
As we have seen above a Transposition Cipher works by re arranging the letters in a message as per the specific pattern or rule. So let us imagine that you have a message like "HELLO" and you want to keep it private. Instead of changing the letters themselves, we rearrange them based on a rule.
For example, let us say the rule is to write the message in a grid with a specific number of columns. If we use 2 columns, "HELLO" will become −

So now we will read the given columns in different order, like from left to right, top to bottom. So the encrypted message will be "HLOEL".
To decrypt it, we just have to know the rule used to rearrange the letters. In this case, we know it was a 2-column grid, so we will rearrange the letters back into "HELLO".
That's the main concept of how a Transposition Cipher works. It is like playing a game of rearranging letters in a secret way.
Types of Transposition Cipher Algorithm
Rail Fence cipher − The transposition cipher known as the "Rail Fence" cipher takes its name from how it is encoded. The plaintext of the rail fence cipher is written downward on successive "rails" of an imaginary fence, moving upward at the bottom. Then, rows of the message are read out.
Route cipher − A route cipher reads the plaintext in a pattern given in the key after it has been written down in a grid of given dimensions. Compared to a rail fence, route ciphers have a lot more keys. In reality, even with present technology, the number of possible keys could be too large for messages of a suitable length. But not every key is created equal. Weak route selection will result in large sections of plaintext-text that has just been reversed−which will provide cryptanalysts with a hint as to the routes.
Columnar transposition − When a message is transposed column by column, it is typed out in rows of a certain length and then read out again, with the columns selected in an irregular order. In most cases, a keyword defines both the row width and the column permutation. Any empty positions in an irregular columnar transposition cipher are left unfilled, whereas in a standard columnar transposition cipher, the spaces are filled with nulls. Lastly, the message is read out in columns in the keyword-designated order.
Double transposition − One way to attack a single columnar transposition is to estimate potential column lengths, write the message in its columns and then search for possible anagrams. Therefore, a double transposition was frequently utilised to make it stronger. All that's going on here is two columnar transpositions. For the two transpositions, one key or two separate keys might be used.
Myszkowski transposition − Emile Victor Theodore Myszkowski created a variant type of columnar transposition in 1902, but it required a keyword with recurrent letters. Typically, a keyword letter is regarded as the next letter in alphabetical order when it appears more than once.
Disrupted transposition − Certain grid points are blanked out and not used while filling in the plaintext in a disrupted transposition. This disrupts regular patterns and increases the difficulty of the cryptanalyst's task.
Grilles: Grilles, or actual masks with cutouts, are a different type of transposition cipher that does not rely on a mathematical formula. The correspondents must maintain the confidentiality of a physical key in order to achieve the highly irregular transposition that can be produced throughout the duration determined by the grille's size.
Detection and cryptanalysis − A frequency count can be used by the cryptanalyst to quickly identify basic transposition since it has no impact on the frequency of individual symbols. It is most likely a transposition if the frequency distribution of the ciphertext matches that of the plaintext. Anagramming is a common method of attacking this, which involves moving around portions of the ciphertext, searching for passages that appear to be anagrams of English words, then solving the anagrams. Once such anagrams are located, they can be extended because they provide information on the transposition pattern.
Combinations − Transposition is frequently used in conjunction with other methods. For example, the weakness of both can be avoided by combining a columnar transposition with a straightforward substitution cipher. Because of the transposition, parts of the plaintext stay hidden when high frequency ciphertext symbols are substituted out for high frequency plaintext letters. The replacement makes it impossible to anagrammatically translate the transposition. This method is very effective when paired with fractionation.
Fractionation − When fractionation is used, a prior step that divides each plaintext symbol into many ciphertext symbols, transposition works very well. For example, the plaintext alphabet can be typed out in a grid and each letter in the message could subsequently be substituted with its coordinates. Simply translating the message to Morse code, which uses symbols for dots, dashes, and spaces, is another way to fractionate a message.
Implementation using Python
This implementation shows you how to encrypt and decrypt a message using the Transposition Cipher with a given key in Python. This is a very basic implementation of this cryptography. The key used in the code, shows the number of rows in the grid for encryption and decryption.
Example
Here is the basic implementation of a Transposition Cipher using Python. Check the code below −
def transposition_encrypt(message, key): # Create an empty grid grid = [''] * key # Fill the grid with the message for i, char in enumerate(message): row = i % key grid[row] += char # Join the rows together encrypted_msg = ''.join(grid) return encrypted_msg def transposition_decrypt(encrypted_msg, key): # Calculate the number of columns cols = len(encrypted_msg) // key # Create an empty grid grid = [''] * key # Fill the grid for i in range(cols): for j in range(key): grid[j] += encrypted_msg[i * key + j] # Join the columns decrypted_msg = ''.join(grid) return decrypted_msg # function execution message = "HI THIS IS RIYA" key = 2 encrypted_msg = transposition_encrypt(message, key) print("The Encrypted message:", encrypted_msg) decrypted_msg = transposition_decrypt(encrypted_msg, key) print("The Decrypted message:", decrypted_msg)
Following is the output of the above example −
Input/Output
Encrypted message: H HSI IAITI SRY Decrypted message: HHIIIIS S AT R
Features of Transposition Cipher
Here are some specific features of Transposition Cipher −
Transposition Cipher is simple to figure out because it only rearranges the letters rather than altering them.
There are other methods for rearranging the letters, like writing them in a grid or shifting them to a specific distance.
To decode the message, we have to understand the algorithm or pattern that was applied to the letter rearrangement.
Drawback
As we can see, the Transposition Cipher is not very secure as we compare it with some other encryption methods. Here is the reason why −
This technique only changes the order of letters, which means it is easy for hackers to find out the pattern and decrypt the message.
As we do not change the letters, it is possible to find out the frequency of letters and guess the pattern used to rearrange them.
The security of Transposition Cipher relies mainly on keeping the method of rearranging letters secret. If the pattern or rule is known, the message can be easily decrypted.
It is not suitable for large messages as the rearrangement process can be time-consuming.
In summary, the Transposition Cipher is simple and straightforward to use, but its simplicity makes it less secure for protecting sensitive data.
Summary
In this chapter, we have learned about Transposition Cipher, its working mechanism, features, drawbacks and also a basic implementation in Python. In the coming chapters we will see encryption and decryption algorithms for Transposition Cipher.
To Continue Learning Please Login