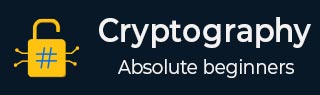
- Cryptography Tutorial
- Cryptography - Home
- Cryptography - Origin
- Cryptography - History
- Cryptography - Principles
- Cryptography - Applications
- Cryptography - Benefits & Drawbacks
- Cryptography - Modern Age
- Cryptography - Traditional Ciphers
- Cryptography - Need for Encryption
- Cryptography - Double Strength Encryption
- Cryptosystems
- Cryptosystems
- Cryptosystems - Components
- Attacks On Cryptosystem
- Cryptosystems - Rainbow table attack
- Cryptosystems - Dictionary attack
- Cryptosystems - Brute force attack
- Cryptosystems - Cryptanalysis Techniques
- Types of Cryptography
- Cryptosystems - Types
- Public Key Encryption
- Modern Symmetric Key Encryption
- Cryptography Hash functions
- Key Management
- Cryptosystems - Key Generation
- Cryptosystems - Key Storage
- Cryptosystems - Key Distribution
- Cryptosystems - Key Revocation
- Block Ciphers
- Cryptosystems - Stream Cipher
- Cryptography - Block Cipher
- Cryptography - Feistel Block Cipher
- Block Cipher Modes of Operation
- Block Cipher Modes of Operation
- Electronic Code Book (ECB) Mode
- Cipher Block Chaining (CBC) Mode
- Cipher Feedback (CFB) Mode
- Output Feedback (OFB) Mode
- Counter (CTR) Mode
- Classic Ciphers
- Cryptography - Reverse Cipher
- Cryptography - Caesar Cipher
- Cryptography - ROT13 Algorithm
- Cryptography - Transposition Cipher
- Cryptography - Encryption Transposition Cipher
- Cryptography - Decryption Transposition Cipher
- Cryptography - Multiplicative Cipher
- Cryptography - Affine Ciphers
- Cryptography - Simple Substitution Cipher
- Cryptography - Encryption of Simple Substitution Cipher
- Cryptography - Decryption of Simple Substitution Cipher
- Cryptography - Vigenere Cipher
- Cryptography - Implementing Vigenere Cipher
- Modern Ciphers
- Base64 Encoding & Decoding
- Cryptography - XOR Encryption
- Substitution techniques
- Cryptography - MonoAlphabetic Cipher
- Cryptography - Hacking Monoalphabetic Cipher
- Cryptography - Polyalphabetic Cipher
- Cryptography - Playfair Cipher
- Cryptography - Hill Cipher
- Polyalphabetic Ciphers
- Cryptography - One-Time Pad Cipher
- Implementation of One Time Pad Cipher
- Cryptography - Transposition Techniques
- Cryptography - Rail Fence Cipher
- Cryptography - Columnar Transposition
- Cryptography - Steganography
- Symmetric Algorithms
- Cryptography - Data Encryption
- Cryptography - Encryption Algorithms
- Cryptography - Data Encryption Standard
- Cryptography - Triple DES
- Cryptography - Double DES
- Advanced Encryption Standard
- Cryptography - AES Structure
- Cryptography - AES Transformation Function
- Cryptography - Substitute Bytes Transformation
- Cryptography - ShiftRows Transformation
- Cryptography - MixColumns Transformation
- Cryptography - AddRoundKey Transformation
- Cryptography - AES Key Expansion Algorithm
- Cryptography - Blowfish Algorithm
- Cryptography - SHA Algorithm
- Cryptography - RC4 Algorithm
- Cryptography - Camellia Encryption Algorithm
- Cryptography - ChaCha20 Encryption Algorithm
- Cryptography - CAST5 Encryption Algorithm
- Cryptography - SEED Encryption Algorithm
- Cryptography - SM4 Encryption Algorithm
- IDEA - International Data Encryption Algorithm
- Public Key (Asymmetric) Cryptography Algorithms
- Cryptography - RSA Algorithm
- Cryptography - RSA Encryption
- Cryptography - RSA Decryption
- Cryptography - Creating RSA Keys
- Cryptography - Hacking RSA Cipher
- Cryptography - ECDSA Algorithm
- Cryptography - DSA Algorithm
- Cryptography - Diffie-Hellman Algorithm
- Data Integrity in Cryptography
- Data Integrity in Cryptography
- Message Authentication
- Cryptography Digital signatures
- Public Key Infrastructure
- Cryptography Useful Resources
- Cryptography - Quick Guide
- Cryptography - Discussion
Cryptography - Simple Substitution Cipher
If you are looking for a simple and widely used cipher, the simple substitution cipher is the excellent choice. In this cipher we will learn about what exactly the simple Substitution Cipher is and how it works in very simple way. So basically in this technique we can replace each plaintext character with a corresponding ciphertext character. Unlike the Caesar cipher algorithm, the simple substitution cipher changes the order of the alphabets so this process adds an extra layer of complexity to the encryption process.
A substitution cipher is an encoding technique where ciphertext is substituted for plaintext units in a predefined system. The "units" can be individual letters (which are the most common), pairs of letters, triplets of letters, or combinations of the aforementioned. By using the inverse substitution, the recipient deciphers the text. We substitute different letters for the plaintext letters in substitution ciphers.
Encrypt the Message − Two elements are needed to encrypt a message: the key (the letter replacements) and the encryption technique (simple substitution, in our case). Note that we should continue to the same technique (simple replacement) but change the key (use alternative letter substitutions) if we believed our messages were no longer secure.
Decrypt the Message − This should not be an issue if you know the key. The key is the same for encryption and decryption, regardless of the possibility that having the ciphertext characters in alphabetical order will be helpful.
For example with a shift of 2, A will be replaced by c, B will become D, C becomes E and so on.
How does it work?
First we have to decide that which letter in the alphabet will be substituted for each given original letter.
So for using a Simple Substitution Cipher for encrypting a message we have to replace each character in the given message with its respective character in the secret character map. For example, if 'A' is mapped to 'D', so each 'A' in the message should be replaced by 'D', and so on for each character.
To decrypt the given encoded message, we will have to reverse the process of encryption. So we will use the secret alphabet mapping to replace each encoded letter with its original letter.
The secret alphabet mapping is the key to the cipher. Without knowing this mapping, it will be very difficult to decrypt the message.
Example
Keys for a simple substitution cipher basically consist of 26 letters. An example key is −
plain alphabet : | abcdefghijklmnopqrstuvwxyz |
cipher alphabet : | phqgiumeaylnofdxjkrcvstzwb |
An example encryption using the above key is −
plaintext : | hello, this world is so beautiful! |
ciphertext : | gtyyj, ngzf cjpyk zf fj htvbnzdby! |
Implementation using Python
So we have implemented a simple substitution cipher with the help of string and random module of Python.
The string module is used to get all lowercase letters (string.ascii_lowercase) and the random module to shuffle the alphabet. We have generated a random mapping of letters in the alphabet, encrypt a message with the help of given mapping, and decrypt an encrypted message with the help of mapping.
Example
Below is a Python implementation for the simple substitution cipher algorithm using string and random module of Python. See the program below −
import string import random def generate_mapping(): alphabet = list(string.ascii_lowercase) # Get all lowercase letters shuffled_alphabet = alphabet[:] # Make a copy of the alphabet random.shuffle(shuffled_alphabet) # Shuffle the copy return dict(zip(alphabet, shuffled_alphabet)) # function for encryption def encrypt(message, mapping): encrypted_message = '' for char in message.lower(): if char in mapping: encrypted_message += mapping[char] else: encrypted_message += char return encrypted_message # function for decryption def decrypt(encrypted_message, mapping): inverse_mapping = {v: k for k, v in mapping.items()} # Create an inverse mapping decrypted_message = '' for char in encrypted_message.lower(): if char in inverse_mapping: decrypted_message += inverse_mapping[char] else: decrypted_message += char return decrypted_message # generate a random mapping mapping = generate_mapping() # message to encrypt message = "Hello, world!" # encrypt the message encrypted_message = encrypt(message, mapping) print("Our Encrypted Message:", encrypted_message) # eecrypt the message decrypted_message = decrypt(encrypted_message, mapping) print("Our Decrypted Message:", decrypted_message)
Following is the output of the above example −
Input/Output
Our Encrypted Message: nsgga, taigj! Our Decrypted Message: hello, world!
Drawbacks
The basic disadvantage of this Cipher is that it is very easy to crack. Once an attacker finds out the mapping which is used to substitute letters, so he can easily decode any message.
And it can also be cracked using frequency analysis. When you look at how often some letters or groups of letters appear in the encrypted message, it becomes easier to guess it. It means that the Simple Substitution Cipher is not very secure for securing our private data.
Cryptanalysis of Simple Substitution Cipher
Cryptanalysis of simple substitution cipher refers to deciphering a secret message is a code in which a letter is typically substituted with another letter. We can decipher it by comparing the code's letter frequency to the known letter frequency of the language. Using indications like punctuation and spaces, together with a few typical patterns, we can gradually decipher the message. It is the same as figuring out a puzzle by guessing which elements represent based on how frequently they appear.
Summary
In this chapter, we learned about a simple Substitution Cipher and its simple implementations in different programming languages. A substitution cipher is very easy to understand and use, but when it comes to security, it is not secure. The reason behind this is its simplicity: once attacker cracks the map so he can easily decipher any message. One of the techniques used to break these ciphers is frequency analysis. So it is not recommended for situations where strong security is required.
To Continue Learning Please Login