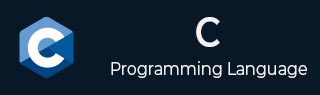
- C Programming Tutorial
- C - Home
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Tokens
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Type Conversion
- C - Booleans
- C - Constants
- C - Literals
- C - Escape sequences
- C - Format Specifiers
- C - Storage Classes
- C - Operators
- C - Arithmetic Operators
- C - Relational Operators
- C - Logical Operators
- C - Bitwise Operators
- C - Assignment Operators
- C - Unary Operators
- C - Increment and Decrement Operators
- C - Ternary Operator
- C - sizeof Operator
- C - Operator Precedence
- C - Misc Operators
- C - Decision Making
- C - if statement
- C - if...else statement
- C - nested if statements
- C - switch statement
- C - nested switch statements
- C - Loops
- C - While loop
- C - For loop
- C - Do...while loop
- C - Nested loop
- C - Infinite loop
- C - Break Statement
- C - Continue Statement
- C - goto Statement
- C - Functions
- C - Main Functions
- C - Function call by Value
- C - Function call by reference
- C - Nested Functions
- C - Variadic Functions
- C - User-Defined Functions
- C - Callback Function
- C - Return Statement
- C - Recursion
- C - Scope Rules
- C - Static Variables
- C - Global Variables
- C - Arrays
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
- C - Pointers
- C - Pointers and Arrays
- C - Applications of Pointers
- C - Pointer Arithmetics
- C - Array of Pointers
- C - Pointer to Pointer
- C - Passing Pointers to Functions
- C - Return Pointer from Functions
- C - Function Pointers
- C - Pointer to an Array
- C - Pointers to Structures
- C - Chain of Pointers
- C - Pointer vs Array
- C - Character Pointers and Functions
- C - NULL Pointer
- C - void Pointer
- C - Dangling Pointers
- C - Dereference Pointer
- C - Near, Far and Huge Pointers
- C - Initialization of Pointer Arrays
- C - Pointers vs. Multi-dimensional Arrays
- C - Strings
- C - Array of Strings
- C - Special Characters
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Self-Referential Structures
- C - Lookup Tables
- C - Dot (.) Operator
- C - Enumeration (or enum)
- C - Nested Structures
- C - Structure Padding and Packing
- C - Anonymous Structure and Union
- C - Unions
- C - Bit Fields
- C - Typedef
- C - Input & Output
- C - File I/O
- C - Preprocessors
- C - Pragmas
- C - Preprocessor Operators
- C - Macros
- C - Header Files
- C - Type Casting
- C - Error Handling
- C - Variable Arguments
- C - Memory Management
- C - Command Line Arguments
- C Programming Resources
- C - Questions & Answers
- C - Quick Guide
- C - Useful Resources
- C - Discussion
C Programming - Online Quiz
Following quiz provides Multiple Choice Questions (MCQs) related to C Programming Framework. You will have to read all the given answers and click over the correct answer. If you are not sure about the answer then you can check the answer using Show Answer button. You can use Next Quiz button to check new set of questions in the quiz.
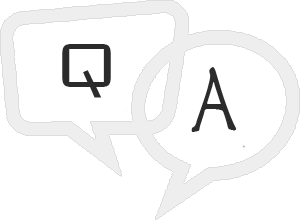
Q 1 - What is the output of the following code snippet?
#include<stdio.h> main() { short unsigned int i = 0; printf("%u\n", i--); }
Answer : A
Explanation
0, with post decrement operator value of the variable will be considered as the expression’s value and later gets decremented.
Q 2 - What is the output of the following program?
#include<stdio.h> main() { int i = 1; while( i++<=5 ) printf("%d ",i++); }
Answer : C
Explanation
2 4 6, at while first compared and later incremented and in printf printed first and incremented later.
Q 3 - What is the output of the following program?
#include<stdio.h> main() { int a[] = {2,1}; printf("%d", *a); }
Answer : C
Explanation
2, as ‘a’ refers to base address.
Q 4 - Choose the correct option in respect to the following program.
#include<stdio.h> void f(int const i) { i=5; } main() { int x = 10; f(x); }
I - Error in the statement ‘void f(int const i)’
II - Error in the statement i=5.
A - Statements I & II are true
Answer : D
Explanation
We cannot modify a constant as in statement i=5.
Q 5 - What is the output of the following program?
#include<stdio.h> main() { char s[20] = "Hello\0Hi"; printf("%d %d", strlen(s), sizeof(s)); }
Answer : C
Explanation
Length of the string is count of character upto ‘\0’. sizeof – reports the size of the array.
Q 6 - In C, what are the various types of real data type (floating point data type)?
Answer : C
Explanation
There are three types of floating point data type = 1) float with storage size 4 byte, 2) double with storage size 8 byte, and 3) long double with storage size 10 byte.
Q 7 - “Stderr” is a standard error.
Answer : B
Explanation
Standard error stream (Stderr) = Any program use it for error messages and diagnostics issue.
Q 8 - The return keyword used to transfer control from a function back to the calling function.
Answer : A
Explanation
In C, the return function stops the execution of a function and returns control with value to the calling function. Execution begins in the calling function by instantly following the call.
Q 9 - In C, what is the correct hierarchy of arithmetic operations?
Answer : C
Explanation
In C, there are 5 arithmetic operators (+, -, *, /, and %) that can be used in performing arithmetic operations.
Q 10 - In the given below code, what will be the value of a variable x?
#include<stdio.h> int main() { int y = 100; const int x = y; printf("%d\n", x); return 0; }
Answer : A
Explanation
Although, integer y = 100; and constant integer x is equal to y. here in the given above program we have to print the x value, so that it will be 100.
To Continue Learning Please Login