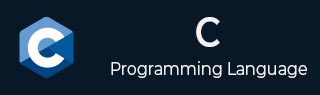
- C Programming Tutorial
- C - Home
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Tokens
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Type Conversion
- C - Booleans
- C - Constants
- C - Literals
- C - Escape sequences
- C - Format Specifiers
- C - Storage Classes
- C - Operators
- C - Arithmetic Operators
- C - Relational Operators
- C - Logical Operators
- C - Bitwise Operators
- C - Assignment Operators
- C - Unary Operators
- C - Increment and Decrement Operators
- C - Ternary Operator
- C - sizeof Operator
- C - Operator Precedence
- C - Misc Operators
- C - Decision Making
- C - if statement
- C - if...else statement
- C - nested if statements
- C - switch statement
- C - nested switch statements
- C - Loops
- C - While loop
- C - For loop
- C - Do...while loop
- C - Nested loop
- C - Infinite loop
- C - Break Statement
- C - Continue Statement
- C - goto Statement
- C - Functions
- C - Main Functions
- C - Function call by Value
- C - Function call by reference
- C - Nested Functions
- C - Variadic Functions
- C - User-Defined Functions
- C - Callback Function
- C - Return Statement
- C - Recursion
- C - Scope Rules
- C - Static Variables
- C - Global Variables
- C - Arrays
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
- C - Pointers
- C - Pointers and Arrays
- C - Applications of Pointers
- C - Pointer Arithmetics
- C - Array of Pointers
- C - Passing Pointers to Functions
- C - Strings
- C - Array of Strings
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Pointers to Structures
- C - Self-Referential Structures
- C - Nested Structures
- C - Unions
- C - Bit Fields
- C - Typedef
- C - Input & Output
- C - File I/O
- C - Preprocessors
- C - Header Files
- C - Type Casting
- C - Error Handling
- C - Variable Arguments
- C - Memory Management
- C - Command Line Arguments
- C Programming Resources
- C - Questions & Answers
- C - Quick Guide
- C - Useful Resources
- C - Discussion
Global Variables in C
Global variables are defined outside a function, usually at the top of a program. Global variables hold their values throughout the lifetime of a program and they can be accessed inside any of the functions defined for the program.
If a function accesses and modifies the value of a global variable, then the updated value is available for other function calls.
If a variable defined in a certain file, then you can still access it inside another code module as a global variable by using the extern keyword. The extern keyword can also be used to access a global variable instead of a local variable of the same name.
Declaring Global Variable
The declaration of a global variable in C is similar to the declaration of the normal (local) variables but global variables are declared outside of the functions.
Consider the following syntax to declare a global variable:
data_type variable_name; // main or any function int main() { }
Example of Global Variable in C
The following program shows how global variables are used in a program:
#include <stdio.h> /* global variable declaration */ int g = 10; int main(){ /* local variable declaration */ int a; /* actual initialization */ a = g * 2; printf("Value of a = %d, and g = %d\n", a, g); return 0; }
Output
When you run this code, it will produce the following output −
Value of a = 20, and g = 10
Accessing Global Variables
Global variables are accessible in all the functions in a C program. If any function updates the value of a global variable, then its updated value will subsequently be available for all the other functions.
Example of Accessing Global Variables
The following example demonstrates the example of accessing global variables in C language:
#include <stdio.h> /* global variable declaration */ int g = 10; int function1(); int function2(); int main(){ printf("Value of Global variable g = %d\n", g); function1(); printf("Updated value of Global variable g = %d\n", g); function2(); printf("Updated value of Global variable g = %d\n", g); return 0; } int function1(){ g = g + 10; printf("New value of g in function1(): %d\n", g); return 0; } int function2(){ printf("The value of g in function2(): %d\n", g); g = g + 10; return 0; }
Run the code and check its output −
Value of Global variable g = 10 New value of g in function1(): 20 Updated value of Global variable g = 20 The value of g in function2(): 20 Updated value of Global variable g = 30
Scope and Accessibility of Global Variables
Global variables are available to only those functions which are defined after their declaration. Global variables are declared outside of any function, so they can be accessed by all functions within the same file by default.
Example
In this example, we declared a global variable (x) before the main() function. There is another global variable y that is declared after the main() function but before function1(). In such a case, the variable y, even thought it is a global variable, is not available for use in the main() function, as it is declared afterwards. As a result, you get an error.
#include <stdio.h> /* global variable declaration */ int x = 10; int function1(); int main(){ printf("value of Global variable x= %d y=%d\n", x, y); function1(); return 0; } int y = 20; int function1(){ printf ("Value of Global variable x = %d y = %d\n", x, y); }
When you run this code, it will produce an error −
Line no 11: error: 'y' undeclared (first use in this function) 11 | printf ("Value of Global variable x = %d y = %d\n", x, y); | ^
Accessing Global Variables With extern Keyword
If you want to access a global variable when a local variable with same name is also there in the program, then you should use the extern keyword.
Example
In this C Program, we have a global variable and a local variable with the same name (x). Now, let's see how we can use the keyword "extern" to avoid confusion −
#include <stdio.h> // Global variable x int x = 50; int main(){ // Local variable x int x = 10;{ extern int x; printf("Value of global x is %d\n", x); } printf("Value of local x is %d\n", x); return 0; }
Output
When you run this code, it will produce the following output −
Value of global x is 50 Value of local x is 10
Avoid Using Global Variables
Global variables can simplify the programming logic. Global variables can be accessed across functions and you need not use a parameter passing technique to pass variables from one function to another. However, it is not wise or efficient to have too many global variables in a C program, as the memory occupied by these variables is not released till the end of the program.
Using global declaration is not considered a good programming practice because it doesn’t implement structured approach. Global declaration is also not advised from security point of view, as they are accessible to all the functions. Finally, using global declarations can make a program difficult to debug, maintain, and scale up.
To Continue Learning Please Login