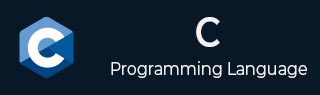
- C Programming Tutorial
- C - Home
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Tokens
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Type Conversion
- C - Booleans
- C - Constants
- C - Literals
- C - Escape sequences
- C - Format Specifiers
- C - Storage Classes
- C - Operators
- C - Arithmetic Operators
- C - Relational Operators
- C - Logical Operators
- C - Bitwise Operators
- C - Assignment Operators
- C - Unary Operators
- C - Increment and Decrement Operators
- C - Ternary Operator
- C - sizeof Operator
- C - Operator Precedence
- C - Misc Operators
- C - Decision Making
- C - if statement
- C - if...else statement
- C - nested if statements
- C - switch statement
- C - nested switch statements
- C - Loops
- C - While loop
- C - For loop
- C - Do...while loop
- C - Nested loop
- C - Infinite loop
- C - Break Statement
- C - Continue Statement
- C - goto Statement
- C - Functions
- C - Main Functions
- C - Function call by Value
- C - Function call by reference
- C - Nested Functions
- C - Variadic Functions
- C - User-Defined Functions
- C - Callback Function
- C - Return Statement
- C - Recursion
- C - Scope Rules
- C - Static Variables
- C - Global Variables
- C - Arrays
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
- C - Pointers
- C - Pointers and Arrays
- C - Applications of Pointers
- C - Pointer Arithmetics
- C - Array of Pointers
- C - Passing Pointers to Functions
- C - Strings
- C - Array of Strings
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Pointers to Structures
- C - Self-Referential Structures
- C - Nested Structures
- C - Unions
- C - Bit Fields
- C - Typedef
- C - Input & Output
- C - File I/O
- C - Preprocessors
- C - Header Files
- C - Type Casting
- C - Error Handling
- C - Variable Arguments
- C - Memory Management
- C - Command Line Arguments
- C Programming Resources
- C - Questions & Answers
- C - Quick Guide
- C - Useful Resources
- C - Discussion
Ternary Operator in C
The ternary operator (?:) in C is a type of conditional operator. The term "ternary" implies that the operator has three operands. The ternary operator is often used to put multiple conditional (if-else) statements in a more compact manner.
Syntax of Ternary Operator in C
The ternary operator is used with the following syntax −
exp1 ? exp2 : exp3
It uses three operands −
- exp1 − A Boolean expression evaluating to true or false
- exp2 − Returned by the ? operator when exp1 is true
- exp3 − Returned by the ? operator when exp1 is false
Example 1: Ternary Operator in C
The following C program uses the ternary operator to check if the value of a variable is even or odd.
#include <stdio.h> int main(){ int a = 10; (a % 2 == 0) ? printf("%d is Even \n", a) : printf("%d is Odd \n", a); return 0; }
Output
When you run this code, it will produce the following output −
10 is Even
Change the value of "a" to 15 and run the code again. Now you will get the following output −
15 is Odd
Example 2
The conditional operator is a compact representation of if–else construct. We can rewrite the logic of checking the odd/even number by the following code −
#include <stdio.h> int main(){ int a = 10; if (a % 2 == 0){ printf("%d is Even\n", a); } else{ printf("%d is Odd\n", a); } return 0; }
Output
Run the code and check its output −
10 is Even
Example 3
The following program compares the two variables "a" and "b", and assigns the one with the greater value to the variable "c".
#include <stdio.h> int main(){ int a = 100, b = 20, c; c = (a >= b) ? a : b; printf ("a: %d b: %d c: %d\n", a, b, c); return 0; }
Output
When you run this code, it will produce the following output −
a: 100 b: 20 c: 100
Example 4
The corresponding code with if–else construct is as follows −
#include <stdio.h> int main(){ int a = 100, b = 20, c; if (a >= b){ c = a; } else { c = b; } printf ("a: %d b: %d c: %d\n", a, b, c); return 0; }
Output
Run the code and check its output −
a: 100 b: 20 c: 100
Example 5
If you need to put multiple statements in the true and/or false operand of the ternary operator, you must separate them by commas, as shown below −
#include <stdio.h> int main(){ int a = 100, b = 20, c; c = (a >= b) ? printf ("a is larger "), c = a : printf("b is larger "), c = b; printf ("a: %d b: %d c: %d\n", a, b, c); return 0; }
Output
In this code, the greater number is assigned to "c", along with printing the appropriate message.
a is larger a: 100 b: 20 c: 20
Example 6
The corresponding program with the use of if–else statements is as follows −
#include <stdio.h> int main(){ int a = 100, b = 20, c; if(a >= b){ printf("a is larger \n"); c = a; } else{ printf("b is larger \n"); c = b; } printf ("a: %d b: %d c: %d\n", a, b, c); return 0; }
Output
Run the code and check its output −
a is larger a: 100 b: 20 c: 100
Nested Ternary Operator
Just as we can use nested if-else statements, we can use the ternary operator inside the True operand as well as the False operand.
exp1 ? (exp2 ? expr3 : expr4) : (exp5 ? expr6: expr7)
First C checks if expr1 is true. If so, it checks expr2. If it is true, the result is expr3; if false, the result is expr4.
If expr1 turns false, it may check if expr5 is true and return expr6 or expr7.
Example 1
Let us develop a C program to determine whether a number is divisible by 2 and 3, or by 2 but not 3, or 3 but not 2, or neither by 2 and 3. We will use nested condition operators for this purpose, as shown in the following code −
#include <stdio.h> int main(){ int a = 15; printf("a: %d\n", a); (a % 2 == 0) ? ( (a%3 == 0)? printf("divisible by 2 and 3") : printf("divisible by 2 but not 3")) : ( (a%3 == 0)? printf("divisible by 3 but not 2") : printf("not divisible by 2, not divisible by 3") ); return 0; }
Output
Check for different values −
a: 15 divisible by 3 but not 2 a: 16 divisible by 2 but not 3 a: 17 not divisible by 2, not divisible by 3 a: 18 divisible by 2 and 3
Example 2
In this program, we have used nested if–else statements for the same purpose instead of conditional operators −
#include <stdio.h> int main(){ int a = 15; printf("a: %d\n", a); if(a % 2 == 0){ if (a % 3 == 0){ printf("divisible by 2 and 3"); } else { printf("divisible by 2 but not 3"); } } else{ if(a % 3 == 0){ printf("divisible by 3 but not 2"); } else { printf("not divisible by 2, not divisible by 3"); } } return 0; }
Output
When you run this code, it will produce the following output −
a: 15 divisible by 3 but not 2
To Continue Learning Please Login