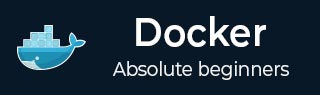
- Docker Tutorial
- Docker - Home
- Docker - Overview
- Docker - Installing on Linux
- Docker - Installation
- Docker - Hub
- Docker - Images
- Docker - Containers
- Docker - Registries
- Docker - Compose
- Docker - Working With Containers
- Docker - Architecture
- Docker - Layers
- Docker - Container & Hosts
- Docker - Configuration
- Docker - Containers & Shells
- Docker - Dockerfile
- Docker - Building Files
- Docker - Public Repositories
- Docker - Managing Ports
- Docker - Web Server
- Docker - Commands
- Docker - Container Linking
- Docker - Data Storage
- Docker - Networking
- Docker - Toolbox
- Docker - Cloud
- Docker - Logging
- Docker - Continuous Integration
- Docker - Kubernetes Architecture
- Docker - Working of Kubernetes
- Docker Setting Services
- Docker - Setting Node.js
- Docker - Setting MongoDB
- Docker - Setting NGINX
- Docker - Setting ASP.Net
- Docker Setting - Python
- Docker Setting - Java
- Docker Setting - Redis
- Docker Setting - Alpine
- Docker Setting - BusyBox
- Docker Useful Resources
- Docker - Quick Guide
- Docker - Useful Resources
- Docker - Discussion
How to Run Python in a Docker Container?
Python has revolutionized the software development industry because of its simplicity, extensive set of libraries, and versatility. When projects scale along with increased complexities in the development and deployment environments, it becomes very difficult to manage the Python dependencies. Consequently, significant challenges arise in ensuring that the runtime is consistent across multiple environments. This is where running Python in Docker comes into the picture.
Docker is a leading containerization platform that offers a streamlined approach to package, distribute, and run applications across different environments. Running Python in Docker comes with a lot of benefits - it enhances portability, dependency management, isolation, and scalability. Docker encapsulates Python applications with their dependencies in lightweight containers ensuring consistent behavior across development, testing, and production environments.
The major ways to run Python inside Docker containers are −
- Use Dockerfiles with official Python Docker base images.
- Leverage Docker Compose to define and run multi-container Python Docker applications.
- Create a virtual environment within the Docker container to isolate Python dependencies.
In this chapter, let’s discuss how to run Python in Docker containers using different ways with the help of a step-by-step approach, Docker commands, and examples.
How to run Python inside Docker using Dockerfiles?
Here’s a step-by-step process of running Python inside Docker with the help of Dockerfiles.
Step 1: Create a Dockerfile
Start by creating a Dockerfile in the project directory. The Dockerfile should contain the instruction to build the custom Docker image on top of the base Python image. Here’s an example Python Dockerfile.
# Use the official Python image as the base image FROM python:3.9 # Set the working directory within the container WORKDIR /app # Copy the requirements.txt file into the container COPY requirements.txt /app/ # Install Python dependencies listed in requirements.txt RUN pip install -r requirements.txt # Copy the rest of the application code into the container COPY . /app # Specify the command to run when the container starts CMD ["python", "app.py"]
Step 2: Define Python Dependencies
You can create a requirements.txt file if your Python application relies on external dependencies. This file should contain a list of all the dependencies along with the version that will be used by the Dockerfile to install while building the image.
Flask==2.1.0 requests==2.26.0
Step 3: Build the Docker Image
Next, navigate to the Dockerfile location inside the terminal and run the following Docker build command to build the Python Docker image.
docker build -t my-python-app .
- `-t my-python-app` − The -t flag tags the Docker image with the name `my-python-app`.
Step 4: Run the Docker Container
Once you have successfully built the Docker image, you can run the Docker container for that image using the Docker run command.
docker run -d -p 5000:5000 my-python-app
- `-d` − This flag detaches the container and helps you to run it in the background.
- `-p 5000:5000` − The -p flag maps port 5000 on the host machine to port 5000 inside the Docker container. You can adjust the port numbers as per your requirements.
- `my-python-app` − Here, you have to specify the name of the Docker image to be used for creating the container.
Step 5: Access the Python Application
If your Python application is running on a web server, you open a web browser and navigate to `http://localhost:5000` to access the web application.
How to run Python using Docker Compose?
Next, let’s understand how to run Python using Docker Compose. Docker Compose helps you to simplify multi-container Docker application management using a single YAML file. It lets you orchestrate services and streamline development workflows, ensuring consistency across environments.
Step 1: Create Docker Compose Configuration
Start by creating a docker-compose.yml in the project directory. In this file, you have to mention the services and their configurations.
version: '3' services: web: build: . ports: - "5000:5000"
- `version: '3'` − Specifies the version of the Docker Compose file format.
- `services` − Defines the services to be run by Docker Compose.
- `web` − Name of the service.
- `build: .` − Specifies the build context for the service, indicating that the Dockerfile is located in the current directory.
- `ports` − Maps ports between the host and the container.
Step 2: Create a Dockerfile
Next, create a Dockerfile in the project directory containing the instructions to build the Docker image.
FROM python:3.9 WORKDIR /app COPY requirements.txt /app/ RUN pip install -r requirements.txt COPY . /app CMD ["python", "app.py"]
Step 3: Define Python Dependencies
Mention your external dependencies in the requirements.txt file.
Flask==2.1.0 requests==2.26.0
Step 4: Build and Run with Docker Compose
The next step is to build and run using Docker Compose. Navigate to the directory containing the containing the `docker-compose.yml` file. Execute the following command to build and run the services defined in the Compose file −
docker-compose up -d
- `-d` − It allows you to detach the containers and run them in the background.
Step 5: Access the Python Application
You can access your Python application web server by opening a web browser and navigating to `http://localhost:5000`.
Step 6: Stopping the Services
If you want to stop the services defined in the `docker-compose.yml` file, you can run the following command −
docker-compose down
This command will help you to stop and remove the containers, their networks, and volumes associated with the services.
How to run Python in a virtual environment within the Docker?
Next, if you want to run Python in a virtual environment within Docker, you can follow the below steps. Virtual environments are used to isolate Python dependencies within a project.
Step 1: Create a Dockerfile
Create a Dockerfile containing all the instructions.
# Use the official Python image as the base image FROM python:3.9 # Set the working directory within the container WORKDIR /app # Copy the requirements.txt file into the container COPY requirements.txt /app/ # Create and activate a virtual environment RUN python -m venv venv RUN . venv/bin/activate && pip install -r requirements.txt # Copy the rest of the application code into the container COPY . /app # Specify the command to run when the container starts CMD ["python", "app.py"]
In this Dockerfile, the first run command creates a virtual environment named `venv` within the Docker container using Python's built-in `venv` module. The second run command activates the virtual environment (`venv`) and installs the Python dependencies listed in the `requirements.txt` file using `pip`. This isolates the dependencies from the global Python environment.
Step 2: Define Python Dependencies
Define your external dependencies in a `requirements.txt` file listing all the required packages.
Flask==2.1.0 requests==2.26.0
Step 3: Build the Docker Image
Navigate to your project directory containing the Dockerfile in the terminal and execute the following command to build the Docker image −
docker build -t my-python-app .
Step 4: Run the Docker Container
Now that you have built the Docker image successfully, you can run a Docker container based on that image using the following command −
docker run -d -p 5000:5000 my-python-app
You can access your Python web server by opening a web browser and navigating to `http://localhost:5000`.
Conclusion
In this chapter, we have learned how to run Python inside Docker containers. This allowed us a streamlined and efficient approach to managing dependencies. Whether through Dockerfiles, Docker Compose, or virtual environments within Docker containers, we can containerize Python applications and their dependencies.
Frequently Asked Questions
Q1. How to run Python tests in Docker?
You can create a Dockerfile with the required test dependencies and instructions in order to run Python tests in Docker. This includes setting up test frameworks in the Docker image, such as pytest or unittest, and executing the tests from the entry point or command of the container.
To guarantee that any changes made to the code are reflected in the tests run within the container, you may also mount your test code inside the Docker container using volumes. This creates a consistent testing environment across several platforms.
Q2. How to use Python debugger in docker?
You can set up the Dockerfile to include the Python debugger package (e.g., `pdb}) together with your application code in order to use the Python debugger in a Docker container. Then, when the container is operating, you may use the debugging commands or breakpoints to launch the debugger inside the container.
Making sure that your Docker container is set up for interactive debugging is essential. Usually, this is done by running the container in interactive mode (`docker run -it}) and opening the ports required for remote debugging, if needed.
Q3. How do I open a VSCode inside a docker container?
VSCode's "Remote - Containers" plugin must be used in order to launch Visual Studio Code (VSCode) inside of a Docker container. Once the extension is installed, you can create, launch, and attach to a Docker container that is set up for your project by opening your project folder in VSCode and using the "Remote-Containers: Open Folder in Container" command.
This allows you to build, debug, and test your Python apps right within the container with access to all of the VSCode features and extensions. It does this by seamlessly integrating the VSCode IDE into the Docker environment.
Q4. How to remote debug the Python docker container?
You can set up your Dockerfile to expose the relevant debugging ports (5678 for PyCharm remote debugging, for example) and include any necessary debugger packages (such `pydevd}) in order to remotely debug a Python Docker container. Ensure that the host machine is mapped to the exposed debugging ports when the Docker container is operating, so the debugger can connect from an external IDE or debugger client.
To enable remote debugging within the Docker container, further, ensure that your Python application is launched in debug mode and set up to listen for incoming debugger connections.
To Continue Learning Please Login