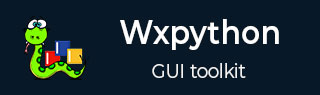
- wxPython Tutorial
- wxPython - Home
- wxPython - Introduction
- wxPython - Environment
- wxPython - Hello World
- wxPython - GUI Builder Tools
- wxPython - Major Classes
- wxPython - Event Handling
- wxPython - Layout Management
- wxPython - Buttons
- wxPython - Dockable Windows
- Multiple Document Interface
- wxPython - Drawing API
- wxPython - Drag and Drop
- wxPython Resources
- wxPython - Quick Guide
- wxPython - Useful Resources
- wxPython - Discussion
wxPython - TextCtrl Class
In a GUI interface, the input is most commonly collected in a text box where the user can type using the keyboard. In wxPython, an object of wx.TextCtrl class serves this purpose. It is a control in which the text can be displayed and edited. The TextCtrl widget can be a single line, multi-line or a password field. TextCtrl class constructor takes the following form −
wx.TextCtrl(parent, id, value, pos, size, style)
The style parameter takes one or more constants from the following list −
S.N. | Parameters & Description |
---|---|
1 | wx.TE_MULTILINE The text control allows multiple lines. If this style is not specified, the line break characters should not be used in the controls value. |
2 | wx.TE_PASSWORD The text will be echoed as asterisks |
3 | wx.TE_READONLY The text will not be user-editable |
4 | wxTE_LEFT The text in the control will be left-justified (default) |
5 | wxTE_CENTRE The text in the control will be centered |
6 | wxTE_RIGHT The text in the control will be right-justified |
The important methods of wx.TextCtrl class are −
S.N. | Methods & Description |
---|---|
1 | AppendText() Adds text to the end of text control |
2 | Clear() Clears the contents |
3 | GetValue() Returns the contents of the text box |
4 | Replace() Replaces the entire or portion of text in the box |
5 | SetEditable() Makes the text box editable or read-only |
6 | SetMaxLength() Sets maximum number of characters the control can hold |
7 | SetValue() Sets the contents in the text box programmatically |
8 | IsMultiLine() Returns true if set to TE_MULTILINE |
The following event binders are responsible for event handling related to entering text in TextCtrl box −
S.N. | Events & Description |
---|---|
1 | EVT_TEXT Responds to changes in the contents of text box, either by manually keying in, or programmatically |
2 | EVT_TEXT_ENTER Invokes associated handler when Enter key is pressed in the text box |
3 | EVT_TEXT_MAXLEN Triggers associated handler as soon as the length of text entered reaches the value of SetMaxLength() function |
Example
In the following example, four objects of wx.TextCtrl class with different attributes are placed on the panel.
self.t1 = wx.TextCtrl(panel) self.t2 = wx.TextCtrl(panel,style = wx.TE_PASSWORD) self.t3 = wx.TextCtrl(panel,size = (200,100),style = wx.TE_MULTILINE) self.t4 = wx.TextCtrl ( panel, value = "ReadOnly Text", style = wx.TE_READONLY | wx.TE_CENTER )
While the first is a normal text box, the second is a password field. The third one is a multiline text box and the last text box is non-editable.
EVT_TEXT binder on first box triggers OnKeyTyped() method for each key stroke in it. The second box is having its MaxLength set to 5. EVT_TEXT_MAXLEN binder sends OnMaxLen() function running as soon as the user tries to type more than 5 characters. The multiline text box responds to Enter key pressed because of EVT_TEXT_ENTER binder.
The complete code is as follows −
import wx class Mywin(wx.Frame): def __init__(self, parent, title): super(Mywin, self).__init__(parent, title = title,size = (350,250)) panel = wx.Panel(self) vbox = wx.BoxSizer(wx.VERTICAL) hbox1 = wx.BoxSizer(wx.HORIZONTAL) l1 = wx.StaticText(panel, -1, "Text Field") hbox1.Add(l1, 1, wx.EXPAND|wx.ALIGN_LEFT|wx.ALL,5) self.t1 = wx.TextCtrl(panel) hbox1.Add(self.t1,1,wx.EXPAND|wx.ALIGN_LEFT|wx.ALL,5) self.t1.Bind(wx.EVT_TEXT,self.OnKeyTyped) vbox.Add(hbox1) hbox2 = wx.BoxSizer(wx.HORIZONTAL) l2 = wx.StaticText(panel, -1, "password field") hbox2.Add(l2, 1, wx.ALIGN_LEFT|wx.ALL,5) self.t2 = wx.TextCtrl(panel,style = wx.TE_PASSWORD) self.t2.SetMaxLength(5) hbox2.Add(self.t2,1,wx.EXPAND|wx.ALIGN_LEFT|wx.ALL,5) vbox.Add(hbox2) self.t2.Bind(wx.EVT_TEXT_MAXLEN,self.OnMaxLen) hbox3 = wx.BoxSizer(wx.HORIZONTAL) l3 = wx.StaticText(panel, -1, "Multiline Text") hbox3.Add(l3,1, wx.EXPAND|wx.ALIGN_LEFT|wx.ALL,5) self.t3 = wx.TextCtrl(panel,size = (200,100),style = wx.TE_MULTILINE) hbox3.Add(self.t3,1,wx.EXPAND|wx.ALIGN_LEFT|wx.ALL,5) vbox.Add(hbox3) self.t3.Bind(wx.EVT_TEXT_ENTER,self.OnEnterPressed) hbox4 = wx.BoxSizer(wx.HORIZONTAL) l4 = wx.StaticText(panel, -1, "Read only text") hbox4.Add(l4, 1, wx.EXPAND|wx.ALIGN_LEFT|wx.ALL,5) self.t4 = wx.TextCtrl(panel, value = "ReadOnly Text",style = wx.TE_READONLY|wx.TE_CENTER) hbox4.Add(self.t4,1,wx.EXPAND|wx.ALIGN_LEFT|wx.ALL,5) vbox.Add(hbox4) panel.SetSizer(vbox) self.Centre() self.Show() self.Fit() def OnKeyTyped(self, event): print event.GetString() def OnEnterPressed(self,event): print "Enter pressed" def OnMaxLen(self,event): print "Maximum length reached" app = wx.App() Mywin(None, 'TextCtrl demo') app.MainLoop()
The above code produces the following output −
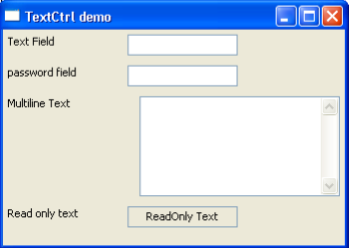