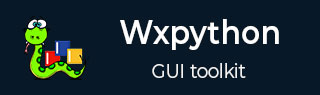
- wxPython Tutorial
- wxPython - Home
- wxPython - Introduction
- wxPython - Environment
- wxPython - Hello World
- wxPython - GUI Builder Tools
- wxPython - Major Classes
- wxPython - Event Handling
- wxPython - Layout Management
- wxPython - Buttons
- wxPython - Dockable Windows
- Multiple Document Interface
- wxPython - Drawing API
- wxPython - Drag and Drop
- wxPython Resources
- wxPython - Quick Guide
- wxPython - Useful Resources
- wxPython - Discussion
wxPython - FlexiGridSizer
This sizer also has a two dimensional grid. However, it provides little more flexibility in laying out the controls in the cells. Although all the controls in the same row have the same height, and all the controls in the same column have the same width, the size of each cell is not uniform as in GridSizer.
Width and/or height of cells in a single column/row can be allowed to extend by AddGrowableRow() and AddGrowableCol() method.
wx.FlexiGridSizer class constructor takes four parameters −
Wx.FlexiGridSizer(rows, cols, vgap, hgap)
A brief description of major methods of wx.FlexiGridSizer is given below −
S.N. | Methods & Description |
---|---|
1 | AddGrowableCol() Specifies a column of given index to grow if extra height is available. |
2 | AddGrowRow() Specifies a row of given index to grow if extra width is available. |
3 | SetFlexibleDirection() Specifies whether sizer’s flexibility affects the row, the column or both. |
Example
A two-column form is designed with the following code. The first column contains labels and the second contains text boxes. The second column is set to be growable. Similarly, the third row is set to be growable. (Note that the row index and the column index starts from 0). The second parameter in AddGrowableCol() and AddGrowableRow() function is the proportion of growth.
fgs.AddGrowableRow(2, 1) fgs.AddGrowableCol(1, 1)
The entire code is as follows −
import wx class Example(wx.Frame): def __init__(self, parent, title): super(Example, self).__init__(parent, title = title, size = (300, 250)) self.InitUI() self.Centre() self.Show() def InitUI(self): panel = wx.Panel(self) hbox = wx.BoxSizer(wx.HORIZONTAL) fgs = wx.FlexGridSizer(3, 2, 10,10) title = wx.StaticText(panel, label = "Title") author = wx.StaticText(panel, label = "Name of the Author") review = wx.StaticText(panel, label = "Review") tc1 = wx.TextCtrl(panel) tc2 = wx.TextCtrl(panel) tc3 = wx.TextCtrl(panel, style = wx.TE_MULTILINE) fgs.AddMany([(title), (tc1, 1, wx.EXPAND), (author), (tc2, 1, wx.EXPAND), (review, 1, wx.EXPAND), (tc3, 1, wx.EXPAND)]) fgs.AddGrowableRow(2, 1) fgs.AddGrowableCol(1, 1) hbox.Add(fgs, proportion = 2, flag = wx.ALL|wx.EXPAND, border = 15) panel.SetSizer(hbox) app = wx.App() Example(None, title = 'FlexiGrid Demo') app.MainLoop()
The above code produces the following output −
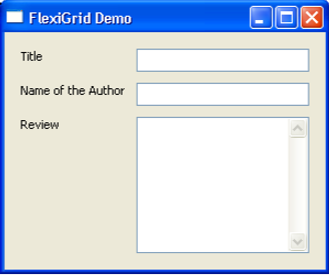