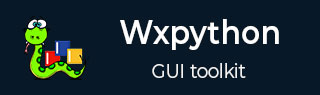
- wxPython Tutorial
- wxPython - Home
- wxPython - Introduction
- wxPython - Environment
- wxPython - Hello World
- wxPython - GUI Builder Tools
- wxPython - Major Classes
- wxPython - Event Handling
- wxPython - Layout Management
- wxPython - Buttons
- wxPython - Dockable Windows
- Multiple Document Interface
- wxPython - Drawing API
- wxPython - Drag and Drop
- wxPython Resources
- wxPython - Quick Guide
- wxPython - Useful Resources
- wxPython - Discussion
wxPython - CheckBox Class
A checkbox displays a small labeled rectangular box. When clicked, a checkmark appears inside the rectangle to indicate that a choice is made. Checkboxes are preferred over radio buttons when the user is to be allowed to make more than one choice. In this case, the third state is called mixed or undetermined state, generally used in ‘doesn’t apply’ scenario.
Normally, a checkbox object has two states (checked or unchecked). Tristate checkbox can also be constructed if the appropriate style parameter is given.
wx.CheckBox class constructor takes the following parameters −
Wx.CheckBox(parent, id, label, pos, size, style)
The following style parameter values can be used −
S.N. | Parameters & Description |
---|---|
1 | wx.CHK_2STATE Creates two state checkbox. Default |
2 | wx.CHK_3STATE Creates three state checkbox |
3 | wx.ALIGN_RIGHT Puts a box label to the left of the checkbox |
This class has two important methods − GetState() returns true or false depending on if the checkbox is checked or not. SetValue() is used to select a checkbox programmatically.
wx.EVT_CHECKBOX is the only event binder available. Associated event handler will be invoked every time any checkbox on the frame is checked or unchecked.
Example
Following is a simple example demonstrating the use of three checkboxes. Handler function OnChecked() identifies the checkbox, which is responsible for the event and displays its state.
The complete code is −
import wx class Example(wx.Frame): def __init__(self, parent, title): super(Example, self).__init__(parent, title = title,size = (200,200)) self.InitUI() def InitUI(self): pnl = wx.Panel(self) self.cb1 = wx.CheckBox(pnl, label = 'Value A',pos = (10,10)) self.cb2 = wx.CheckBox(pnl, label = 'Value B',pos = (10,40)) self.cb3 = wx.CheckBox(pnl, label = 'Value C',pos = (10,70)) self.Bind(wx.EVT_CHECKBOX,self.onChecked) self.Centre() self.Show(True) def onChecked(self, e): cb = e.GetEventObject() print cb.GetLabel(),' is clicked',cb.GetValue() ex = wx.App() Example(None,'CheckBox') ex.MainLoop()
The above code produces the following output −
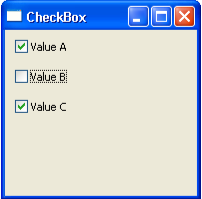
Value A is clicked True
Value B is clicked True
Value C is clicked True
Value B is clicked False