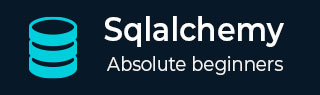
- SQLAlchemy Tutorial
- SQLAlchemy - Home
- SQLAlchemy - Introduction
- SQLAlchemy Core
- Expression Language
- Connecting to Database
- Creating Table
- SQL Expressions
- Executing Expression
- Selecting Rows
- Using Textual SQL
- Using Aliases
- Using UPDATE Expression
- Using DELETE Expression
- Using Multiple Tables
- Using Multiple Table Updates
- Parameter-Ordered Updates
- Multiple Table Deletes
- Using Joins
- Using Conjunctions
- Using Functions
- Using Set Operations
- SQLAlchemy ORM
- Declaring Mapping
- Creating Session
- Adding Objects
- Using Query
- Updating Objects
- Applying Filter
- Filter Operators
- Returning List and Scalars
- Textual SQL
- Building Relationship
- Working with Related Objects
- Working with Joins
- Common Relationship Operators
- Eager Loading
- Deleting Related Objects
- Many to Many Relationships
- Dialects
- SQLAlchemy Useful Resources
- SQLAlchemy - Quick Guide
- SQLAlchemy - Useful Resources
- SQLAlchemy - Discussion
SQLAlchemy Core - Using Multiple Tables
One of the important features of RDBMS is establishing relation between tables. SQL operations like SELECT, UPDATE and DELETE can be performed on related tables. This section describes these operations using SQLAlchemy.
For this purpose, two tables are created in our SQLite database (college.db). The students table has the same structure as given in the previous section; whereas the addresses table has st_id column which is mapped to id column in students table using foreign key constraint.
The following code will create two tables in college.db −
from sqlalchemy import create_engine, MetaData, Table, Column, Integer, String, ForeignKey engine = create_engine('sqlite:///college.db', echo=True) meta = MetaData() students = Table( 'students', meta, Column('id', Integer, primary_key = True), Column('name', String), Column('lastname', String), ) addresses = Table( 'addresses', meta, Column('id', Integer, primary_key = True), Column('st_id', Integer, ForeignKey('students.id')), Column('postal_add', String), Column('email_add', String)) meta.create_all(engine)
Above code will translate to CREATE TABLE queries for students and addresses table as below −
CREATE TABLE students ( id INTEGER NOT NULL, name VARCHAR, lastname VARCHAR, PRIMARY KEY (id) ) CREATE TABLE addresses ( id INTEGER NOT NULL, st_id INTEGER, postal_add VARCHAR, email_add VARCHAR, PRIMARY KEY (id), FOREIGN KEY(st_id) REFERENCES students (id) )
The following screenshots present the above code very clearly −
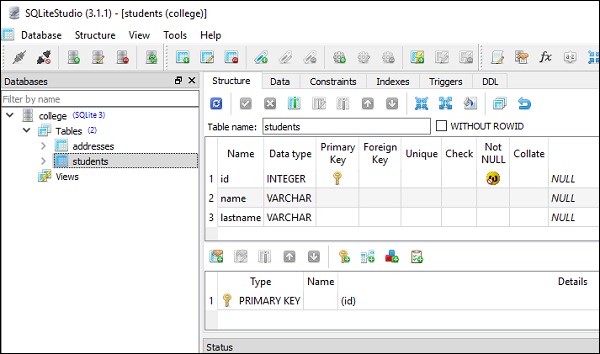

These tables are populated with data by executing insert() method of table objects. To insert 5 rows in students table, you can use the code given below −
from sqlalchemy import create_engine, MetaData, Table, Column, Integer, String engine = create_engine('sqlite:///college.db', echo = True) meta = MetaData() conn = engine.connect() students = Table( 'students', meta, Column('id', Integer, primary_key = True), Column('name', String), Column('lastname', String), ) conn.execute(students.insert(), [ {'name':'Ravi', 'lastname':'Kapoor'}, {'name':'Rajiv', 'lastname' : 'Khanna'}, {'name':'Komal','lastname' : 'Bhandari'}, {'name':'Abdul','lastname' : 'Sattar'}, {'name':'Priya','lastname' : 'Rajhans'}, ])
Rows are added in addresses table with the help of the following code −
from sqlalchemy import create_engine, MetaData, Table, Column, Integer, String engine = create_engine('sqlite:///college.db', echo = True) meta = MetaData() conn = engine.connect() addresses = Table( 'addresses', meta, Column('id', Integer, primary_key = True), Column('st_id', Integer), Column('postal_add', String), Column('email_add', String) ) conn.execute(addresses.insert(), [ {'st_id':1, 'postal_add':'Shivajinagar Pune', 'email_add':'ravi@gmail.com'}, {'st_id':1, 'postal_add':'ChurchGate Mumbai', 'email_add':'kapoor@gmail.com'}, {'st_id':3, 'postal_add':'Jubilee Hills Hyderabad', 'email_add':'komal@gmail.com'}, {'st_id':5, 'postal_add':'MG Road Bangaluru', 'email_add':'as@yahoo.com'}, {'st_id':2, 'postal_add':'Cannought Place new Delhi', 'email_add':'admin@khanna.com'}, ])
Note that the st_id column in addresses table refers to id column in students table. We can now use this relation to fetch data from both the tables. We want to fetch name and lastname from students table corresponding to st_id in the addresses table.
from sqlalchemy.sql import select s = select([students, addresses]).where(students.c.id == addresses.c.st_id) result = conn.execute(s) for row in result: print (row)
The select objects will effectively translate into following SQL expression joining two tables on common relation −
SELECT students.id, students.name, students.lastname, addresses.id, addresses.st_id, addresses.postal_add, addresses.email_add FROM students, addresses WHERE students.id = addresses.st_id
This will produce output extracting corresponding data from both tables as follows −
(1, 'Ravi', 'Kapoor', 1, 1, 'Shivajinagar Pune', 'ravi@gmail.com') (1, 'Ravi', 'Kapoor', 2, 1, 'ChurchGate Mumbai', 'kapoor@gmail.com') (3, 'Komal', 'Bhandari', 3, 3, 'Jubilee Hills Hyderabad', 'komal@gmail.com') (5, 'Priya', 'Rajhans', 4, 5, 'MG Road Bangaluru', 'as@yahoo.com') (2, 'Rajiv', 'Khanna', 5, 2, 'Cannought Place new Delhi', 'admin@khanna.com')