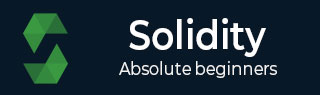
- Solidity Tutorial
- Solidity - Home
- Solidity - Overview
- Solidity - Environment Setup
- Solidity - Basic Syntax
- Solidity - First Application
- Solidity - Comments
- Solidity - Types
- Solidity - Variables
- Solidity - Variable Scope
- Solidity - Operators
- Solidity - Loops
- Solidity - Decision Making
- Solidity - Strings
- Solidity - Arrays
- Solidity - Enums
- Solidity - Structs
- Solidity - Mappings
- Solidity - Conversions
- Solidity - Ether Units
- Solidity - Special Variables
- Solidity - Style Guide
- Solidity Functions
- Solidity - Functions
- Solidity - Function Modifiers
- Solidity - View Functions
- Solidity - Pure Functions
- Solidity - Fallback Function
- Function Overloading
- Mathematical Functions
- Cryptographic Functions
- Solidity Common Patterns
- Solidity - Withdrawal Pattern
- Solidity - Restricted Access
- Solidity Advanced
- Solidity - Contracts
- Solidity - Inheritance
- Solidity - Constructors
- Solidity - Abstract Contracts
- Solidity - Interfaces
- Solidity - Libraries
- Solidity - Assembly
- Solidity - Events
- Solidity - Error Handling
- Solidity Useful Resources
- Solidity - Quick Guide
- Solidity - Useful Resources
- Solidity - Discussion
Solidity - Fallback Function
Fallback function is a special function available to a contract. It has following features −
It is called when a non-existent function is called on the contract.
It is required to be marked external.
It has no name.
It has no arguments
It can not return any thing.
It can be defined one per contract.
If not marked payable, it will throw exception if contract receives plain ether without data.
Following example shows the concept of a fallback function per contract.
Example
pragma solidity ^0.5.0; contract Test { uint public x ; function() external { x = 1; } } contract Sink { function() external payable { } } contract Caller { function callTest(Test test) public returns (bool) { (bool success,) = address(test).call(abi.encodeWithSignature("nonExistingFunction()")); require(success); // test.x is now 1 address payable testPayable = address(uint160(address(test))); // Sending ether to Test contract, // the transfer will fail, i.e. this returns false here. return (testPayable.send(2 ether)); } function callSink(Sink sink) public returns (bool) { address payable sinkPayable = address(sink); return (sinkPayable.send(2 ether)); } }
Advertisements