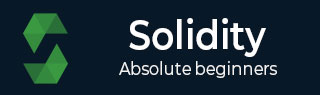
- Solidity Tutorial
- Solidity - Home
- Solidity - Overview
- Solidity - Environment Setup
- Solidity - Basic Syntax
- Solidity - First Application
- Solidity - Comments
- Solidity - Types
- Solidity - Variables
- Solidity - Variable Scope
- Solidity - Operators
- Solidity - Loops
- Solidity - Decision Making
- Solidity - Strings
- Solidity - Arrays
- Solidity - Enums
- Solidity - Structs
- Solidity - Mappings
- Solidity - Conversions
- Solidity - Ether Units
- Solidity - Special Variables
- Solidity - Style Guide
- Solidity Functions
- Solidity - Functions
- Solidity - Function Modifiers
- Solidity - View Functions
- Solidity - Pure Functions
- Solidity - Fallback Function
- Function Overloading
- Mathematical Functions
- Cryptographic Functions
- Solidity Common Patterns
- Solidity - Withdrawal Pattern
- Solidity - Restricted Access
- Solidity Advanced
- Solidity - Contracts
- Solidity - Inheritance
- Solidity - Constructors
- Solidity - Abstract Contracts
- Solidity - Interfaces
- Solidity - Libraries
- Solidity - Assembly
- Solidity - Events
- Solidity - Error Handling
- Solidity Useful Resources
- Solidity - Quick Guide
- Solidity - Useful Resources
- Solidity - Discussion
Solidity - Basic Syntax
A Solidity source files can contain an any number of contract definitions, import directives and pragma directives.
Let's start with a simple source file of Solidity. Following is an example of a Solidity file −
pragma solidity >=0.4.0 <0.6.0; contract SimpleStorage { uint storedData; function set(uint x) public { storedData = x; } function get() public view returns (uint) { return storedData; } }
Pragma
The first line is a pragma directive which tells that the source code is written for Solidity version 0.4.0 or anything newer that does not break functionality up to, but not including, version 0.6.0.
A pragma directive is always local to a source file and if you import another file, the pragma from that file will not automatically apply to the importing file.
So a pragma for a file which will not compile earlier than version 0.4.0 and it will also not work on a compiler starting from version 0.5.0 will be written as follows −
pragma solidity ^0.4.0;
Here the second condition is added by using ^.
Contract
A Solidity contract is a collection of code (its functions) and data (its state) that resides at a specific address on the Ethereumblockchain.
The line uintstoredData declares a state variable called storedData of type uint and the functions set and get can be used to modify or retrieve the value of the variable.
Importing Files
Though above example does not have an import statement but Solidity supports import statements that are very similar to those available in JavaScript.
The following statement imports all global symbols from "filename".
import "filename";
The following example creates a new global symbol symbolName whose members are all the global symbols from "filename".
import * as symbolName from "filename";
To import a file x from the same directory as the current file, use import "./x" as x;. If you use import "x" as x; instead, a different file could be referenced in a global "include directory".
Reserved Keywords
Following are the reserved keywords in Solidity −
abstract | after | alias | apply |
auto | case | catch | copyof |
default | define | final | immutable |
implements | in | inline | let |
macro | match | mutable | null |
of | override | partial | promise |
reference | relocatable | sealed | sizeof |
static | supports | switch | try |
typedef | typeof | unchecked |