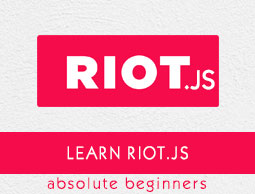
- RIOT.JS Tutorial
- RIOT.JS - Home
- RIOT.JS - Overview
- RIOT.JS - Environment Setup
- RIOT.JS - First Application
- RIOT.JS - Tags
- RIOT.JS - Expressions
- RIOT.JS - Styling
- RIOT.JS - Conditional
- RIOT.JS - Yield
- RIOT.JS - Event Handling
- RIOT.JS - Accessing DOM
- RIOT.JS - Loops
- RIOT.JS - Mixin
- RIOT.JS - Observables
- RIOT.JS Useful Resources
- RIOT.JS - Quick Guide
- RIOT.JS - Useful Resources
- RIOT.JS - Discussion
RIOT.JS - Quick Guide
RIOT.JS - Overview
RIOT.js is a very small size/lightweight Web Component based UI library to develop web-applications. It combines the benefits of React.JS and Polymer with very concise implementation and simple constructs to learn and use. It minified version is nearly of 10KB size.
Following are the key features of RIOT.js
Expression Bindings
Very small payload during DOM updates and reflows.
Changes propagates downwards from parent tags to children tags/controls.
Uses pre-compiled expressions and cache them for high performance.
Provides good constrol over lifecycle events.
Follows Standards
No proprietary event system
No dependency on any polyfill libraries.
No extra attributes added to existing HTML.
Integrates well with jQuery.
Core values
RIOT.js is developed considering the following values.
Simple and minimalistic.
Easy to learn and implement.
Provide Reactive Views to build user interfaces.
Provide Event Library to build APIs with independent modules.
To take care of application behaviour with browser back button.
RIOT.JS - Environment Setup
There are two ways to use RIOT js.
Local Installation − You can download RIOT library on your local machine and include it in your HTML code.
CDN Based Version − You can include RIOT library into your HTML code directly from Content Delivery Network (CDN).
Local Installation
Go to the https://v3.riotjs.vercel.app/download/ to download the latest version available.
Now put downloaded riot.min.js file in a directory of your website, e.g. /riotjs.
Example
Now you can include riotjs library in your HTML file as follows −
<!DOCTYPE html> <html> <head> <script src = "/riotjs/riot.min.js"></script> <script src = "https://cdnjs.cloudflare.com/ajax/libs/riot/3.13.2/riot+compiler.min.js"></script> </head> <body> <messageTag></messageTag> <script> var tagHtml = "<h1>Hello World!</h1>"; riot.tag("messageTag", tagHtml); riot.mount("messageTag"); </script> </body> </html>
This will produce following result −
CDN Based Version
You can include RIOT js library into your HTML code directly from Content Delivery Network (CDN). Google and Microsoft provides content deliver for the latest version.
Note − We are using CDNJS version of the library throughout this tutorial.
Example
Now let us rewrite above example using jQuery library from CDNJS.
<!DOCTYPE html> <html> <head> <script src = "https://cdnjs.cloudflare.com/ajax/libs/riot/3.13.2/riot+compiler.min.js"></script> </head> <body> <messageTag></messageTag> <script> var tagHtml = "<h1>Hello World!</h1>"; riot.tag("messageTag", tagHtml); riot.mount("messageTag"); </script> </body> </html>
This will produce following result −
RIOT.JS - First Application
RIOT works by building custom, reusable html tags. These tags are similar to Web components and are reusable across pages and web apps.
Steps to use RIOT
Import riot.js in the html page.
<head> <script src = "https://cdnjs.cloudflare.com/ajax/libs/riot/3.13.2/riot+compiler.min.js"></script> </head>
Start a script section and define tag content as html. Script can also be included which we'll see later in the tutorial.
var tagHtml = "<h1>Hello World!</h1>";
Define a tag using riot.tag() method. Pass it the name of the tag, messageTag and variable containing tag content.
riot.tag("messageTag", tagHtml);
Mount the tag using riot.mount() method. Pass it the name of the tag, messageTag. Mounting process mounts the messageTag in all its occurrences in the html page. MessageTag tag should be defined using riot.js prior to mounting.
riot.mount("messageTag"); </script>
Following is the complete example.
Example
<!DOCTYPE html> <html> <head> <script src = "https://cdnjs.cloudflare.com/ajax/libs/riot/3.13.2/riot+compiler.min.js"></script> </head> <body> <messageTag></messageTag> <script> var tagHtml = "<h1>Hello World!</h1>"; riot.tag("messageTag", tagHtml); riot.mount("messageTag"); </script> </body> </html>
This will produce following result −
RIOT.JS - Tags
RIOT works by building custom, reusable html tags. These tags are similar to Web components and are reusable across pages and web apps. When you include the RIOT framework in your HTML page, the imported js creates a riot variable pointing to a riot object. This object contains the functions which is required to interact with the RIOT.js like creating and mounting tags.
We can create and use tags in two ways.
Inline HTML − By calling riot.tag() function. This function takes the tag name and tag definition to create a tag. Tag definition can contain HTML, JavaScript and CSS etc.
Seperate Tag file − By storing the tag definition in tag file. This tag file contains tag definition to create a tag . This file needs to be imported inplace of riot.tag() call.
<script src = "/riotjs/src/messageTag.tag" type = "riot/tag"></script<
Following is the example of inline tag.
Example
<!DOCTYPE html> <html> <head> <script src = "https://cdnjs.cloudflare.com/ajax/libs/riot/3.13.2/riot+compiler.min.js"></script> </head> <body> <messageTag></messageTag> <script> var tagHtml = "<h1>Hello World!</h1>"; riot.tag("messageTag", tagHtml); riot.mount("messageTag"); </script> </body> </html>
This will produce following result −
Following is the example of external file tag.
Example
messageTag.tag
<messageTag> <h1>Hello World!</h1> </messageTag>
index.htm
<!DOCTYPE html> <html> <head> <script src = "https://cdnjs.cloudflare.com/ajax/libs/riot/3.13.2/riot+compiler.min.js"></script> </head> <body> <messageTag></messageTag> <script src = "messageTag.tag" type = "riot/tag"></script> <script> riot.mount("messageTag"); </script> </body> </html>
This will produce following result −
RIOT.JS - Expressions
RIOT js uses {} to define expressions. RIOT js allows following types of expressions.
Simple Expression − Define a variable and use within a tag.
<customTag> <h1>{title}</h1> <script> this.title = "Welcome to TutorialsPoint.COM"; </script> </customTag>
Evaluate Expression − Evaluate a variable when use in an operation.
<customTag> <h2>{val * 5}</h2> <script> this.val = 4; </script> </customTag>
Get value from Options object − To get the value passed to tag via attributes.
Example
Following is the complete example of above concepts.
customTag.tag
<customTag> <h1>{title}</h1> <h2>{val * 5}</h2> <h2>{opts.color}</h2> <script> this.title = "Welcome to TutorialsPoint.COM"; this.val = 4; </script> </customTag>
index.htm
<!DOCTYPE html> <html> <head> <script src = "https://cdnjs.cloudflare.com/ajax/libs/riot/3.13.2/riot+compiler.min.js"></script> </head> <body> <customTag color="red"></customTag> <script src = "customTag.tag" type = "riot/tag"></script> <script> riot.mount("customTag"); </script> </body> </html>
This will produce following result −
RIOT.JS - Styling
RIOT js tags can have their own style and we can define styles within tags which will affect only the content within the tag. We can also set a style class using scripts as well within a tag. Following is the syntax how to achieve styling of RIOT tags.
custom1Tag.tag
<custom1Tag> <h1>{title}</h1> <h2 class = "subTitleClass">{subTitle}</h2> <style> h1 { color: red; } .subHeader { color: green; } </style> <script> this.title = "Welcome to TutorialsPoint.COM"; this.subTitle = "Learning RIOT JS"; this.subTitleClass = "subHeader"; </script> </custom1Tag>
index.htm
<!DOCTYPE html> <html> <head> <script src = "https://cdnjs.cloudflare.com/ajax/libs/riot/3.13.2/riot+compiler.min.js"></script> </head> <body> <h1>Non RIOT Heading</h1> <custom1Tag></custom1Tag> <script src = "custom1Tag.tag" type = "riot/tag"></script> <script> riot.mount("custom1Tag"); </script> </body> </html>
This will produce following result −
RIOT.JS - Conditional
Conditionals are constructs which are used to show/hide elements of RIOT tags. Following are the three conditionals RIOT supports −
if − add/remove element based on value passed.
<custom2Tag> <h2 if = {showMessage}>Using if!</h2> <script> this.showMessage = true; </script> </custom2Tag>
show − shows an element using style = "display:' ' " if passed true.
<custom2Tag> <h2 show = {showMessage}>Using show!</h2> <script> this.showMessage = true; </script> </custom2Tag>
hide − hides an element using style = "display:'none' " if passed true.
<custom2Tag> <h2 show = {showMessage}>Using show!</h2> <script> this.showMessage = true; </script> </custom2Tag>
Example
Following is the complete example.
custom2Tag.tag
<custom2Tag> <h2 if = {showMessage}>Using if!</h2> <h2 if = {show}>Welcome!</h1> <h2 show = {showMessage}>Using show!</h2> <h2 hide = {show}>Using hide!</h2> <script> this.showMessage = true; this.show = false; </script> </custom2Tag>
custom2.htm
<!DOCTYPE html> <html> <head> <script src = "https://cdnjs.cloudflare.com/ajax/libs/riot/3.13.2/riot+compiler.min.js"></script> </head> <body> <custom2Tag></custom2Tag> <script src = "custom2Tag.tag" type = "riot/tag"></script> <script> riot.mount("custom2Tag"); </script> </body> </html>
This will produce following result −
RIOT.JS - Yield
Yield is a mechanism to put external html content into a RIOT tag. There are multiple ways to do an yield.
Simple Yield − If we want to replace a single placeholder in tag. Then use this mechanism.
<custom3Tag> Hello <yield/> </custom3Tag>
<custom3Tag><b>User</b></custom3Tag>
Multiple Yield − If we want to replace multiple placeholders in tag. Then use this mechanism.
<custom4Tag> <br/><br/> Hello <yield from = "first"/> <br/><br/> Hello <yield from = "second"/> </custom4Tag>
<custom4Tag> <yield to = "first">User 1</yield> <yield to = "second">User 2</yield> </custom4Tag>
Example
Following is the complete example.
custom3Tag.tag
<custom3Tag> Hello <yield/> </custom3Tag>
custom4Tag.tag
<custom4Tag> <br/><br/> Hello <yield from = "first"/> <br/><br/> Hello <yield from = "second"/> </custom4Tag>
custom3.htm
<html> <head> <script src = "https://cdnjs.cloudflare.com/ajax/libs/riot/3.13.2/riot+compiler.min.js"></script> </head> <body> <custom3Tag><b>User</b></custom3Tag> <custom4Tag> <yield to = "first">User 1</yield> <yield to = "second">User 2</yield> </custom4Tag> <script src = "custom3Tag.tag" type = "riot/tag"></script> <script src = "custom4Tag.tag" type = "riot/tag"></script> <script> riot.mount("custom3Tag"); riot.mount("custom4Tag"); </script> </body> </html>
This will produce following result −
RIOT.JS - Event Handling
We can attach event to HTML elements in the similar way how we access HTML elements using refs object. As a first step we add a ref attribute to a DOM element and access it using this.ref in the script block of the tag.
Attach ref − Add ref attribute to a DOM element.
<button ref = "clickButton">Click Me!</button>
Use the refs object − Now use the refs object in mount event. This event is fired when RIOT mounts the custom tag and it populates the refs object.
this.on("mount", function() { console.log("Mounting"); console.log(this.refs.username.value); })
Example
Following is the complete example.
custom5Tag.tag
<custom5Tag> <form> <input ref = "username" type = "text" value = "Mahesh"/> <input type = "submit" value = "Click Me!" /> </form> <script> this.on("mount", function() { console.log("Mounting"); console.log(this.refs.username.value); }) </script> </custom5Tag>
custom5.htm
<html> <head> <script src = "https://cdnjs.cloudflare.com/ajax/libs/riot/3.13.2/riot+compiler.min.js"></script> </head> <body> <custom5Tag></custom5Tag> <script src = "custom5Tag.tag" type = "riot/tag"></script> <script> riot.mount("custom5Tag"); </script> </body> </html>
This will produce following result −
RIOT.JS - Accessing DOM
We can access HTML elements using refs object. As a first step we add a ref attribute to a DOM element and access it using this.ref in the script block of the tag.
Attach ref − Add ref attribute to a DOM element.
<button ref = "clickButton">Click Me!</button>
Use the refs object − Now use the refs object in mount event. This event is fired when RIOT mounts the custom tag and it populates the refs object.
this.on("mount", function() { this.refs.clickButton.onclick = function(e) { console.log("clickButton clicked"); return false; }; })
Example
Following is the complete example.
custom6Tag.tag
<custom6Tag> <form ref = "customForm"> <input ref = "username" type = "text" value = "Mahesh"/> <button ref = "clickButton">Click Me!</button> <input type = "submit" value = "Submit" /> </form> <script> this.on("mount", function() { this.refs.clickButton.onclick = function(e) { console.log("clickButton clicked"); return false; }; this.refs.customForm.onsubmit = function(e) { console.log("Form submitted"); return false; }; }) </script> </custom6Tag>
custom6.htm
<html> <head> <script src = "https://cdnjs.cloudflare.com/ajax/libs/riot/3.13.2/riot+compiler.min.js"></script> </head> <body> <custom6Tag></custom6Tag> <script src = "custom6Tag.tag" type = "riot/tag"></script> <script> riot.mount("custom6Tag"); </script> </body> </html>
This will produce following result −
RIOT.JS - Loops
We can iterate through RIOT array of primitives or of Objects and create/update the html elements on the go. Using "each" construct we can achieve it.
Create array − Create an array of object.
this.cities = [ { city : "Shanghai" , country:"China" , done: true }, { city : "Seoul" , country:"South Korea" }, { city : "Moscow" , country:"Russia" } ];
Add each attribute − Now use the "each" attribute.
<ul> <li each = { cities } ></li> </ul>
Iterate array of objects − Iterate the array using object properties.
<input type = "checkbox" checked = { done }> { city } - { country }
Example
Following is the complete example.
custom7Tag.tag
<custom7Tag> <style> ul { list-style-type: none; } </style> <ul> <li each = { cities } > <input type = "checkbox" checked = { done }> { city } - { country } </li> </ul> <script> this.cities = [ { city : "Shanghai" , country:"China" , done: true }, { city : "Seoul" , country:"South Korea" }, { city : "Moscow" , country:"Russia" } ]; </script> </custom7Tag>
custom7.htm
<html> <head> <script src = "https://cdnjs.cloudflare.com/ajax/libs/riot/3.13.2/riot+compiler.min.js"></script> </head> <body> <custom7Tag></custom6Tag> <script src = "custom7Tag.tag" type = "riot/tag"></script> <script> riot.mount("custom7Tag"); </script> </body> </html>
This will produce following result −
RIOT.JS - Mixin
Through Mixin, we can share common functionality among tags. Mixin can be a function, class or object. Consider a case of Authentication Service which each tag should be using.
Define Mixin − Define mixin using riot.mixin() method before calling mount().
riot.mixin('authService', { init: function() { console.log('AuthService Created!') }, login: function(user, password) { if(user == "admin" && password == "admin"){ return 'User is authentic!' }else{ return 'Authentication failed!' } } });
Initialize mixin − Initialize mixin in each tag.
this.mixin('authService')
Use mixin − After initializing, mixin can be used within tag.
this.message = this.login("admin","admin");
Example
Following is the complete example.
custom8Tag.tag
<custom8Tag> <h1>{ message }</h1> <script> this.mixin('authService') this.message = this.login("admin","admin") </script> </custom8Tag>
custom9Tag.tag
<custom9Tag> <h1>{ message }</h1> <script> this.mixin('authService') this.message = this.login("admin1","admin") </script> </custom9Tag>
custom8.htm
<html> <head> <script src = "https://cdnjs.cloudflare.com/ajax/libs/riot/3.13.2/riot+compiler.min.js"></script> </head> <body> <custom8Tag></custom8Tag> <custom9Tag></custom9Tag> <script src = "custom8Tag.tag" type = "riot/tag"></script> <script src = "custom9Tag.tag" type = "riot/tag"></script> <script> riot.mixin('authService', { init: function() { console.log('AuthService Created!') }, login: function(user, password) { if(user == "admin" && password == "admin"){ return 'User is authentic!' }else{ return 'Authentication failed!' } } }); riot.mount("*"); </script> </body> </html>
This will produce following result −
RIOT.JS - Observables
Observables mechanism allows RIOT to send events from one tag to another. Following APIs are important to understand RIOT observables.
riot.observable(element) − Adds Observer support for the given object element or if the argument is empty a new observable instance is created and returned. After this the object is able to trigger and listen to events.
var EventBus = function(){ riot.observable(this); }
element.trigger(events) − Execute all callback functions that listen to the given event.
sendMessage() { riot.eventBus.trigger('message', 'Custom 10 Button Clicked!'); }
element.on(events, callback) − Listen to the given event and execute the callback each time an event is triggered.
riot.eventBus.on('message', function(input) { console.log(input); });
Example
Following is the complete example.
custom10Tag.tag
<custom10Tag> <button onclick = {sendMessage}>Custom 10</button> <script> sendMessage() { riot.eventBus.trigger('message', 'Custom 10 Button Clicked!'); } </script> </custom10Tag>
custom11Tag.tag
<custom11Tag> <script> riot.eventBus.on('message', function(input) { console.log(input); }); </script> </custom11Tag>
custom9.htm
<html> <head> <script src = "https://cdnjs.cloudflare.com/ajax/libs/riot/3.13.2/riot+compiler.min.js"></script> </head> <body> <custom10Tag></custom10Tag> <custom11Tag></custom11Tag> <script src = "custom10Tag.tag" type = "riot/tag"></script> <script src = "custom11Tag.tag" type = "riot/tag"></script> <script> var EventBus = function(){ riot.observable(this); } riot.eventBus = new EventBus(); riot.mount("*"); </script> </body> </html>
This will produce following result −
To Continue Learning Please Login